pthread 创建一个计时器线程,但是该线程可以被想要时候终结
时间: 2023-08-10 11:21:30 浏览: 83
可以使用pthread_create()函数创建一个计时器线程,该线程在一定时间后会执行某个任务,而且可以在任何时候被终止。下面是一个示例代码:
```c
#include <stdio.h>
#include <pthread.h>
#include <unistd.h>
// 定义全局变量,用来表示线程是否需要终止
int g_terminate = 0;
// 计时器线程函数
void* timer_thread(void* arg)
{
int count = 0;
while (!g_terminate) {
printf("Timer: %d\n", count++);
sleep(1);
}
printf("Timer thread terminated.\n");
pthread_exit(NULL);
}
int main()
{
pthread_t tid;
int ret;
// 创建计时器线程
ret = pthread_create(&tid, NULL, timer_thread, NULL);
if (ret != 0) {
printf("Failed to create timer thread.\n");
return -1;
}
// 在需要时终止计时器线程
printf("Press any key to terminate timer thread.\n");
getchar();
g_terminate = 1;
// 等待计时器线程结束
pthread_join(tid, NULL);
printf("Main thread exit.\n");
return 0;
}
```
在上面的示例代码中,我们使用一个全局变量`g_terminate`来表示线程是否需要终止。计时器线程每秒钟打印一个计数器,当`g_terminate`变量被设置为1时,线程终止。在主线程中,我们使用`getchar()`函数等待用户输入任意键,然后设置`g_terminate`变量,终止计时器线程。最后,主线程使用pthread_join()函数等待计时器线程结束。
阅读全文
相关推荐
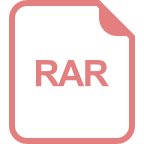
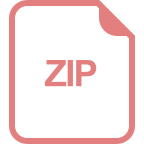
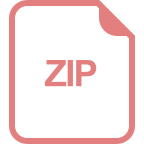
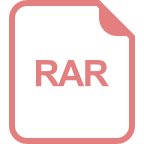
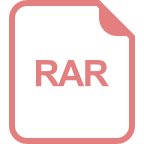
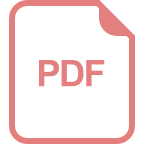
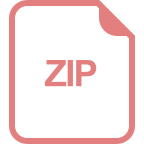
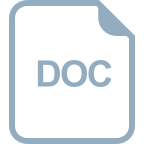
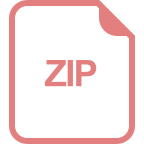
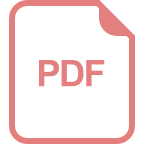
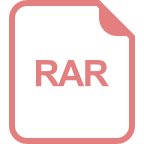
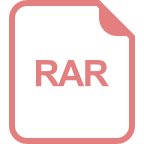
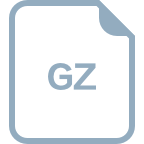
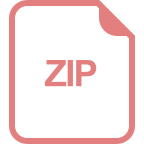
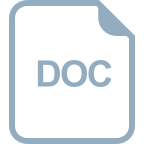