c = torch.tensor([[1, 2], [3, 4]]) print(torch.max(c, dim=0)) print(torch.min(c, dim=0))
时间: 2024-05-12 19:13:46 浏览: 191
这段代码使用了PyTorch库,创建了一个2x2的张量c,并使用torch.max和torch.min函数分别返回c张量在dim=0维度上的最大值和最小值。具体输出结果如下所示:
```
torch.return_types.max(
values=tensor([3, 4]),
indices=tensor([1, 1])
)
torch.return_types.min(
values=tensor([1, 2]),
indices=tensor([0, 0])
)
```
其中,torch.max返回了一个元组,第一个元素是dim=0维度上的最大值,第二个元素是最大值对应的索引。torch.min同理。
相关问题
LDAM损失函数pytorch代码如下:class LDAMLoss(nn.Module): def init(self, cls_num_list, max_m=0.5, weight=None, s=30): super(LDAMLoss, self).init() m_list = 1.0 / np.sqrt(np.sqrt(cls_num_list)) m_list = m_list * (max_m / np.max(m_list)) m_list = torch.cuda.FloatTensor(m_list) self.m_list = m_list assert s > 0 self.s = s if weight is not None: weight = torch.FloatTensor(weight).cuda() self.weight = weight self.cls_num_list = cls_num_list def forward(self, x, target): index = torch.zeros_like(x, dtype=torch.uint8) index_float = index.type(torch.cuda.FloatTensor) batch_m = torch.matmul(self.m_list[None, :], index_float.transpose(1,0)) # 0,1 batch_m = batch_m.view((16, 1)) # size=(batch_size, 1) (-1,1) x_m = x - batch_m output = torch.where(index, x_m, x) if self.weight is not None: output = output * self.weight[None, :] target = torch.flatten(target) # 将 target 转换成 1D Tensor logit = output * self.s return F.cross_entropy(logit, target, weight=self.weight) 模型部分参数如下:# 设置全局参数 model_lr = 1e-5 BATCH_SIZE = 16 EPOCHS = 50 DEVICE = torch.device('cuda:0' if torch.cuda.is_available() else 'cpu') use_amp = True use_dp = True classes = 7 resume = None CLIP_GRAD = 5.0 Best_ACC = 0 #记录最高得分 use_ema=True model_ema_decay=0.9998 start_epoch=1 seed=1 seed_everything(seed) # 数据增强 mixup mixup_fn = Mixup( mixup_alpha=0.8, cutmix_alpha=1.0, cutmix_minmax=None, prob=0.1, switch_prob=0.5, mode='batch', label_smoothing=0.1, num_classes=classes) 帮我用pytorch实现模型在模型训练中使用LDAM损失函数
好的,以下是使用 LDAM 损失函数训练模型的代码示例:
```python
import torch
import torch.nn as nn
import torch.optim as optim
import torch.nn.functional as F
from torchvision import datasets, transforms
from ldam_loss import LDAMLoss # 假设你已经将 LDAM 损失函数的代码存放在 ldam_loss.py 文件中
# 设置全局参数
model_lr = 1e-5
BATCH_SIZE = 16
EPOCHS = 50
DEVICE = torch.device('cuda:0' if torch.cuda.is_available() else 'cpu')
use_amp = True
use_dp = True
classes = 7
resume = None
CLIP_GRAD = 5.0
Best_ACC = 0
use_ema = True
model_ema_decay = 0.9998
start_epoch = 1
seed = 1
# 设置随机种子
def seed_everything(seed):
torch.manual_seed(seed)
torch.cuda.manual_seed_all(seed)
np.random.seed(seed)
random.seed(seed)
seed_everything(seed)
# 数据增强 mixup
mixup_fn = Mixup(
mixup_alpha=0.8,
cutmix_alpha=1.0,
cutmix_minmax=None,
prob=0.1,
switch_prob=0.5,
mode='batch',
label_smoothing=0.1,
num_classes=classes
)
# 加载数据集
train_loader = torch.utils.data.DataLoader(
datasets.CIFAR10('./data', train=True, download=True, transform=transforms.Compose([
transforms.RandomCrop(32, padding=4),
transforms.RandomHorizontalFlip(),
transforms.ToTensor(),
transforms.Normalize((0.5, 0.5, 0.5), (0.5, 0.5, 0.5))
])),
batch_size=BATCH_SIZE, shuffle=True, num_workers=4, pin_memory=True
)
test_loader = torch.utils.data.DataLoader(
datasets.CIFAR10('./data', train=False, transform=transforms.Compose([
transforms.ToTensor(),
transforms.Normalize((0.5, 0.5, 0.5), (0.5, 0.5, 0.5))
])),
batch_size=BATCH_SIZE, shuffle=False, num_workers=4, pin_memory=True
)
# 定义模型
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.conv1 = nn.Conv2d(3, 6, 5)
self.pool = nn.MaxPool2d(2, 2)
self.conv2 = nn.Conv2d(6, 16, 5)
self.fc1 = nn.Linear(16 * 5 * 5, 120)
self.fc2 = nn.Linear(120, 84)
self.fc3 = nn.Linear(84, 10)
def forward(self, x):
x = self.pool(F.relu(self.conv1(x)))
x = self.pool(F.relu(self.conv2(x)))
x = x.view(-1, 16 * 5 * 5)
x = F.relu(self.fc1(x))
x = F.relu(self.fc2(x))
x = self.fc3(x)
return x
# 初始化模型和优化器
model = Net().to(DEVICE)
optimizer = optim.Adam(model.parameters(), lr=model_lr)
# 如果 resume 不为空,则从指定的 checkpoint 恢复模型和优化器
if resume is not None:
checkpoint = torch.load(resume)
model.load_state_dict(checkpoint['model'])
optimizer.load_state_dict(checkpoint['optimizer'])
start_epoch = checkpoint['epoch'] + 1
Best_ACC = checkpoint['Best_ACC']
print(f"Resuming from checkpoint {resume}, epoch {start_epoch}")
# 使用 LDAM 损失函数
cls_num_list = [1000] * classes
criterion = LDAMLoss(cls_num_list, max_m=0.5, s=30).to(DEVICE)
# 训练模型
for epoch in range(start_epoch, EPOCHS + 1):
train_loss = 0
train_acc = 0
model.train()
for batch_idx, (data, target) in enumerate(train_loader):
data, target = data.to(DEVICE), target.to(DEVICE)
data, target_a, target_b, lam = mixup_fn(data, target) # mixup 增强
optimizer.zero_grad()
output = model(data)
loss = lam * criterion(output, target_a) + (1 - lam) * criterion(output, target_b) # 计算 mixup 后的损失函数
loss.backward()
if CLIP_GRAD:
torch.nn.utils.clip_grad_norm_(model.parameters(), CLIP_GRAD) # 梯度裁剪
optimizer.step()
train_loss += loss.item() * data.size(0)
pred = output.argmax(dim=1, keepdim=True)
train_acc += pred.eq(target.view_as(pred)).sum().item()
train_loss /= len(train_loader.dataset)
train_acc /= len(train_loader.dataset)
# 计算测试集上的损失和准确率
test_loss = 0
test_acc = 0
model.eval()
with torch.no_grad():
for data, target in test_loader:
data, target = data.to(DEVICE), target.to(DEVICE)
output = model(data)
test_loss += F.cross_entropy(output, target, reduction='sum').item()
pred = output.argmax(dim=1, keepdim=True)
test_acc += pred.eq(target.view_as(pred)).sum().item()
test_loss /= len(test_loader.dataset)
test_acc /= len(test_loader.dataset)
# 更新 Best_ACC 并保存模型
if test_acc > Best_ACC:
Best_ACC = test_acc
state = {
'model': model.state_dict(),
'optimizer': optimizer.state_dict(),
'epoch': epoch,
'Best_ACC': Best_ACC
}
torch.save(state, f'model_epoch_{epoch}_acc_{Best_ACC:.4f}.pth')
# 打印训练日志
print(f"Epoch {epoch}/{EPOCHS}: train_loss={train_loss:.4f} train_acc={train_acc:.4f} test_loss={test_loss:.4f} test_acc={test_acc:.4f}")
```
这里使用的是 CIFAR-10 数据集和一个简单的卷积神经网络模型,你需要将其替换成你自己的数据集和模型。
model.eval() with torch.no_grad(): # test total_correct = 0 total_num = 0 for x, label in cifar_test: # [b, 3, 32, 32] # [b] x, label = x.to(device), label.to(device) # [b, 10] logits = model(x) # [b] pred = logits.argmax(dim=1) # [b] vs [b] => scalar tensor correct = torch.eq(pred, label).float().sum().item() total_correct += correct total_num += x.size(0) # print(correct) acc = total_correct / total_num print(epoch, 'test acc:', acc) ———————————————— 逐行解释
这段代码用于在训练过程中对模型进行测试,并打印出测试准确率。以下是对代码逐行的解释:
2. `model.eval()`
将模型设置为评估模式,这将冻结模型中的dropout和batch normalization等操作。
3. `with torch.no_grad():`
使用torch.no_grad()上下文管理器,禁用梯度计算。在测试阶段,我们不需要计算梯度,只需要进行前向传播和评估。
6-19. 进行测试:
- `total_correct = 0` 初始化正确分类的样本数量。
- `total_num = 0` 初始化总样本数量。
- `for x, label in cifar_test:` 对测试集数据进行迭代,每次迭代获取一个批次的输入x和对应的标签label。
- `x, label = x.to(device), label.to(device)` 将输入x和标签label移动到指定的设备(GPU或CPU)上。
- `logits = model(x)` 将输入x传递给模型,得到模型的输出logits。
- `pred = logits.argmax(dim=1)` 获取logits中每个样本预测结果的最大值所对应的索引,即预测的类别。
- `correct = torch.eq(pred, label).float().sum().item()` 计算预测正确的样本数量,将预测结果和真实标签进行比较,并将结果转换为浮点数。
- `total_correct += correct` 累加正确分类的样本数量。
- `total_num += x.size(0)` 累加总样本数量,x.size(0)表示批次中的样本数量。
- `acc = total_correct / total_num` 计算测试准确率,即正确分类的样本数量除以总样本数量。
- `print(epoch, 'test acc:', acc)` 打印出当前训练轮数epoch和测试准确率。
这段代码的主要目的是在训练过程中定期对模型进行测试,并打印出测试准确率。通过计算模型在测试集上的准确率可以评估模型的性能。使用torch.no_grad()上下文管理器可以提高代码的运行效率,同时禁用梯度计算以减少内存占用。
阅读全文
相关推荐
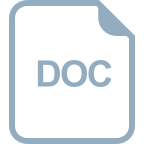
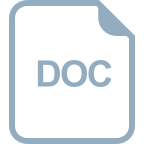
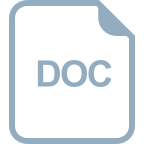













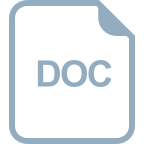