设计person类,包括私有数据成员:姓名;设计student类继承person类,新增私有数据成员:班级;设计类worker继承person类,新增私有数据成员:职业;设计类studentworker继承student类和worker类,新增私有数据成员:工资;在主函数中定义一个studentworker类的对象,调用该对象的show函数输出该对象的信息。
时间: 2024-02-21 08:59:10 浏览: 63
好的,下面是题目要求的四个类的定义:
```c++
#include <iostream>
#include <string>
using namespace std;
class Person {
public:
Person(const string& name) : m_name(name) {}
virtual void show() const {
cout << "Name: " << m_name << endl;
}
private:
string m_name;
};
class Student : public Person {
public:
Student(const string& name, const string& cls) : Person(name), m_cls(cls) {}
virtual void show() const {
Person::show();
cout << "Class: " << m_cls << endl;
}
private:
string m_cls;
};
class Worker : public Person {
public:
Worker(const string& name, const string& job) : Person(name), m_job(job) {}
virtual void show() const {
Person::show();
cout << "Job: " << m_job << endl;
}
private:
string m_job;
};
class StudentWorker : public Student, public Worker {
public:
StudentWorker(const string& name, const string& cls, const string& job, double salary)
: Student(name, cls), Worker(name, job), m_salary(salary) {}
virtual void show() const {
Student::show();
Worker::show();
cout << "Salary: " << m_salary << endl;
}
private:
double m_salary;
};
```
在这个实现中,我们使用了继承和多重继承来实现类的关系。Student类和Worker类都继承自Person类,StudentWorker类则同时继承Student类和Worker类。
在主函数中,我们可以定义一个StudentWorker对象,并调用它的show()函数来输出该对象的信息:
```c++
int main() {
StudentWorker sw("Alice", "Class 1", "Teacher", 5000.0);
sw.show();
return 0;
}
```
输出结果如下:
```
Name: Alice
Class: Class 1
Name: Alice
Job: Teacher
Salary: 5000
```
阅读全文
相关推荐
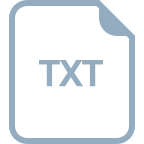
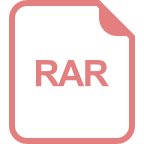
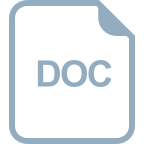

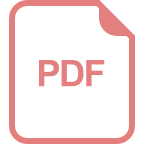
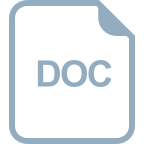
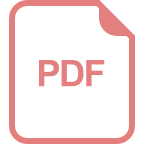
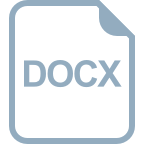
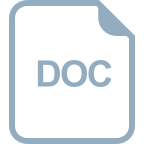
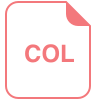

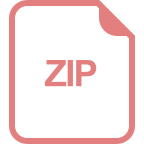
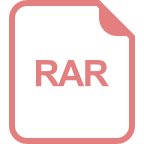
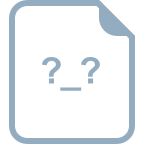
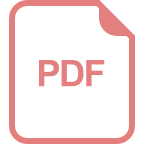
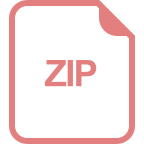
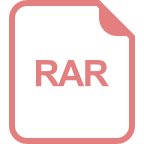
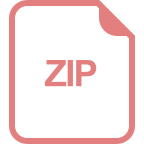