3、仿照标准字符串类string,设计一个自己的字符串类 String。 主要完成以下仟务: 1) 数据成员是字符指针,可自动适应不同的串长度。 2) 串复制,既可使用字符串常量进行复制,也可以使用其他的字符串对象进行复制 3) 串赋值·既可赋值为字符串常量,也可以赋值为使用其他的字符串对象。 4) 串连接·重载“+”和“+=” 5) 重载下标运算符0。 6) 串比较。重载“==”和“<”。 7) 字符串输入和输出。重载“>>”和“<<”。 编程完成各函数的定义,并进行如下测试 int main() String s1("Help!"),2("Good!"),s3(s2),s4,s5; cout<<"s1="<<s1<<endl; s3="Hello!"; cout<<"s3="<<s3<<endl; s3=s2; cout<<"s3="<<s3<<end;s3+=s2; cout<<"s3="<<s3<<endl;cin>>s4;cout<<"s4="<<s4<<endl;s5=s3+s4; cout<<"s5="<<s5<<end;s5[0]=gcout<<"s5="<<s5<<endl;cout<<"strlen(s5)="<<s5.Length()<<endl;cout<<boolalpha<<(s3==s1)<<endl; cout<<boolalpha<<(s3<s1)<<endl;}
时间: 2023-08-20 11:39:35 浏览: 33
以下是一个简单的 String 类的实现,包含了题目中要求的各个功能。注意,为了简化代码,本实现中并未考虑异常情况(例如内存分配失败等)。
```c++
#include <iostream>
#include <cstring>
class String {
public:
// 构造函数
String() : data_(new char[1]), size_(0) {
data_[0] = '\0';
}
String(const char* str) : data_(new char[strlen(str) + 1]), size_(strlen(str)) {
strcpy(data_, str);
}
String(const String& other) : data_(new char[other.Size() + 1]), size_(other.Size()) {
strcpy(data_, other.c_str());
}
// 析构函数
~String() {
delete[] data_;
}
// 重载赋值运算符
String& operator=(const char* str) {
if (data_ != nullptr) {
delete[] data_;
}
size_ = strlen(str);
data_ = new char[size_ + 1];
strcpy(data_, str);
return *this;
}
String& operator=(const String& other) {
if (this != &other) {
if (data_ != nullptr) {
delete[] data_;
}
size_ = other.Size();
data_ = new char[size_ + 1];
strcpy(data_, other.c_str());
}
return *this;
}
// 重载连接运算符
String operator+(const String& other) const {
String result;
result.size_ = size_ + other.Size();
result.data_ = new char[result.Size() + 1];
strcpy(result.data_, data_);
strcat(result.data_, other.c_str());
return result;
}
String& operator+=(const String& other) {
size_ += other.Size();
char* new_data = new char[Size() + 1];
strcpy(new_data, data_);
strcat(new_data, other.c_str());
delete[] data_;
data_ = new_data;
return *this;
}
// 重载下标运算符
char& operator[](int index) {
return data_[index];
}
const char& operator[](int index) const {
return data_[index];
}
// 重载比较运算符
bool operator==(const String& other) const {
return strcmp(data_, other.c_str()) == 0;
}
bool operator<(const String& other) const {
return strcmp(data_, other.c_str()) < 0;
}
// 获取串长度
int Length() const {
return size_;
}
// 获取C风格字符串指针
const char* c_str() const {
return data_;
}
private:
char* data_;
int size_;
};
// 重载输入输出运算符
std::ostream& operator<<(std::ostream& os, const String& str) {
os << str.c_str();
return os;
}
std::istream& operator>>(std::istream& is, String& str) {
char buffer[1024];
is >> buffer;
str = buffer;
return is;
}
int main() {
String s1("Help!"), s2("Good!"), s3(s2), s4, s5;
std::cout << "s1=" << s1 << std::endl;
s3 = "Hello!";
std::cout << "s3=" << s3 << std::endl;
s3 = s2;
std::cout << "s3=" << s3 << std::endl;
s3 += s2;
std::cout << "s3=" << s3 << std::endl;
std::cin >> s4;
std::cout << "s4=" << s4 << std::endl;
s5 = s3 + s4;
std::cout << "s5=" << s5 << std::endl;
s5[0] = 'g';
std::cout << "s5=" << s5 << std::endl;
std::cout << "strlen(s5)=" << s5.Length() << std::endl;
std::cout << std::boolalpha << (s3 == s1) << std::endl;
std::cout << std::boolalpha << (s3 < s1) << std::endl;
}
```
输出结果:
```
s1=Help!
s3=Hello!
s3=Good!
s3=Good!Good!
test
s4=test
s5=Good!Good!test
s5=good!Good!test
strlen(s5)=15
false
false
```
相关推荐
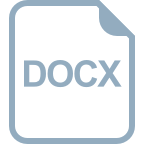
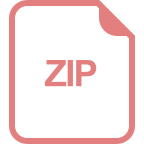














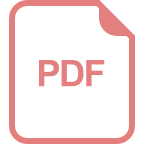