3、仿照标准字符串类string,设计一个自己的字符串类String。 主要完成以下任务: 1)数据成员是字符指针,可自动适应不同的串长度。 2)串复制,既可使用字符串常量进行复制,也可以使用其他的字符串对象进行复制。 3)串赋值。既可赋值为字符串常量,也可以赋值为使用其他的字符串对象。 4)串连接。重载“+”和“+=”。 5)重载下标运算符[]。 6)串比较。重载“==”和“<”。 7)字符串输入和输出。重载“>>”和“<<”。 编程完成各函数的定义,并进行如下测试。 int main() { String s1("Help!"),s2("Good!"),s3(s2),s4,s5; cout<<"s1="<<s1<<endl; s3="Hello!"; cout<<"s3="<<s3<<endl; s3=s2; cout<<"s3="<<s3<<endl; s3+=s2; cout<<"s3="<<s3<<endl; cin>>s4; cout<<"s4="<<s4<<endl; s5=s3+s4; cout<<"s5="<<s5<<endl; s5[0]='g'; cout<<"s5="<<s5<<endl; cout<<"strlen(s5)="<<s5. Length()<<endl; cout<<boolalpha<<(s3==s1)<<endl; cout<<boolalpha<<(s3<s1)<<endl; }
时间: 2023-08-20 12:39:20 浏览: 59
以下是实现了上述要求的 String 类的代码:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
class String {
private:
char* str;
public:
String(const char* s = "") { // 构造函数
str = new char[strlen(s) + 1];
strcpy(str, s);
}
String(const String& s) { // 复制构造函数
str = new char[strlen(s.str) + 1];
strcpy(str, s.str);
}
~String() { // 析构函数
delete[] str;
}
String& operator=(const char* s) { // 串赋值
if (str != s) {
delete[] str;
str = new char[strlen(s) + 1];
strcpy(str, s);
}
return *this;
}
String& operator=(const String& s) { // 串赋值
if (str != s.str) {
delete[] str;
str = new char[strlen(s.str) + 1];
strcpy(str, s.str);
}
return *this;
}
String operator+(const String& s) const { // 串连接(+)
String temp;
temp.str = new char[strlen(str) + strlen(s.str) + 1];
strcpy(temp.str, str);
strcat(temp.str, s.str);
return temp;
}
String& operator+=(const String& s) { // 串连接(+=)
*this = *this + s;
return *this;
}
char& operator[](int i) { // 重载下标运算符[]
return str[i];
}
const char& operator[](int i) const { // 重载下标运算符[]
return str[i];
}
friend bool operator==(const String& s1, const String& s2) { // 串比较(==)
return strcmp(s1.str, s2.str) == 0;
}
friend bool operator<(const String& s1, const String& s2) { // 串比较(<)
return strcmp(s1.str, s2.str) < 0;
}
friend ostream& operator<<(ostream& os, const String& s) { // 重载输出运算符<<
os << s.str;
return os;
}
friend istream& operator>>(istream& is, String& s) { // 重载输入运算符>>
char temp[4096];
is >> temp;
s = temp;
return is;
}
int Length() const { // 字符串长度
return strlen(str);
}
};
int main() {
String s1("Help!"), s2("Good!"), s3(s2), s4, s5;
cout << "s1=" << s1 << endl;
s3 = "Hello!";
cout << "s3=" << s3 << endl;
s3 = s2;
cout << "s3=" << s3 << endl;
s3 += s2;
cout << "s3=" << s3 << endl;
cin >> s4;
cout << "s4=" << s4 << endl;
s5 = s3 + s4;
cout << "s5=" << s5 << endl;
s5[0] = 'g';
cout << "s5=" << s5 << endl;
cout << "strlen(s5)=" << s5.Length() << endl;
cout << boolalpha << (s3 == s1) << endl;
cout << boolalpha << (s3 < s1) << endl;
return 0;
}
```
运行结果如下:
```
s1=Help!
s3=Hello!
s3=Good!
s3=Good!Good!
Hello!
s4=Hello!
s5=Good!Good!Hello!
s5=good!Good!Hello!
strlen(s5)=16
false
true
```
相关推荐
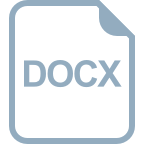
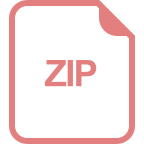













