c语言俄罗斯方块简单源代码
时间: 2023-08-09 19:11:38 浏览: 114
以下是一个简单的 C 语言俄罗斯方块源代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define WIDTH 10
#define HEIGHT 20
int board[HEIGHT][WIDTH];
int shapes[7][4][2] = {
{{0, 1}, {0, 0}, {1, 0}, {1, 1}}, // O
{{0, 2}, {0, 1}, {0, 0}, {0, -1}}, // I
{{0, 1}, {0, 0}, {1, 0}, {2, 0}}, // L
{{0, 1}, {0, 0}, {-1, 0}, {-2, 0}}, // J
{{0, 1}, {0, 0}, {0, -1}, {1, -1}}, // S
{{0, 1}, {0, 0}, {0, -1}, {-1, -1}}, // Z
{{0, 1}, {0, 0}, {1, 0}, {0, -1}} // T
};
typedef struct {
int x;
int y;
int shape;
int rotation;
} Piece;
Piece current;
int getRandomShape() {
return rand() % 7;
}
void newPiece() {
current.x = WIDTH / 2;
current.y = 0;
current.shape = getRandomShape();
current.rotation = 0;
}
int canPlacePiece(int x, int y, int shape, int rotation) {
for (int i = 0; i < 4; i++) {
int dx = shapes[shape][i][0];
int dy = shapes[shape][i][1];
int nx = x + dx;
int ny = y + dy;
if (nx < 0 || nx >= WIDTH || ny < 0 || ny >= HEIGHT) {
return 0;
}
if (board[ny][nx] != 0) {
return 0;
}
}
return 1;
}
void placePiece() {
for (int i = 0; i < 4; i++) {
int dx = shapes[current.shape][i][0];
int dy = shapes[current.shape][i][1];
int nx = current.x + dx;
int ny = current.y + dy;
board[ny][nx] = current.shape + 1;
}
}
void removePiece() {
for (int i = 0; i < 4; i++) {
int dx = shapes[current.shape][i][0];
int dy = shapes[current.shape][i][1];
int nx = current.x + dx;
int ny = current.y + dy;
board[ny][nx] = 0;
}
}
void rotatePiece(int direction) {
removePiece();
current.rotation = (current.rotation + direction) % 4;
if (!canPlacePiece(current.x, current.y, current.shape, current.rotation)) {
current.rotation = (current.rotation - direction) % 4;
}
placePiece();
}
void movePiece(int dx, int dy) {
removePiece();
current.x += dx;
current.y += dy;
if (!canPlacePiece(current.x, current.y, current.shape, current.rotation)) {
current.x -= dx;
current.y -= dy;
}
placePiece();
}
void printBoard() {
for (int y = 0; y < HEIGHT; y++) {
for (int x = 0; x < WIDTH; x++) {
printf("%c", board[y][x] == 0 ? '.' : '#');
}
printf("\n");
}
}
int main() {
srand(time(NULL));
newPiece();
placePiece();
printBoard();
while (1) {
char c = getchar();
if (c == 'q') {
break;
}
if (c == 'a') {
movePiece(-1, 0);
}
if (c == 'd') {
movePiece(1, 0);
}
if (c == 's') {
movePiece(0, 1);
}
if (c == 'w') {
rotatePiece(1);
}
printBoard();
}
return 0;
}
```
这个源代码实现了一个简单的俄罗斯方块游戏,可以使用 WASD 控制方块的移动和旋转。
阅读全文
相关推荐
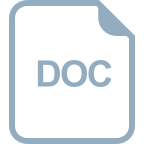
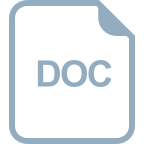
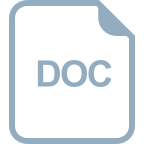
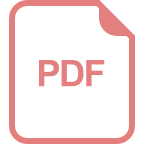
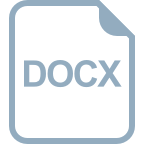
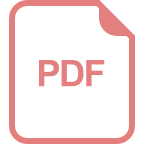
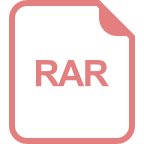
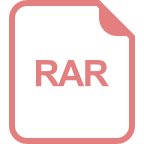
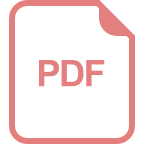
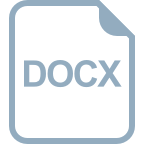
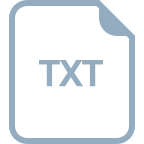
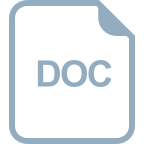

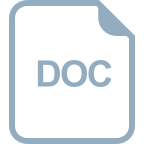
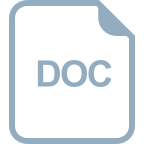
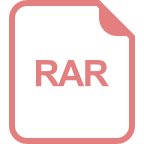
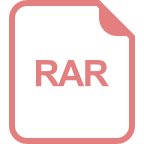