图片抖动算法,用于黑白或者黑白红、黑白黄三色墨水屏
时间: 2024-01-28 16:05:47 浏览: 217
图片抖动算法是一种将彩色图像转换为黑白或其他几种色彩模式的算法。对于黑白或黑白红、黑白黄三色墨水屏,我们可以使用Floyd-Steinberg算法进行抖动处理。
Floyd-Steinberg算法是一种误差扩散算法,它将每个像素的误差分配给周围的像素,并将当前像素的值减去误差值,然后将结果四舍五入为最接近的颜色值。这种算法可以产生更好的黑白或其他几种色彩模式的效果,因为它可以保留更多的图像细节和灰度级别。
具体实现过程如下:
1. 将彩色图像转换为灰度图像
2. 对于每个像素,将其值与最近的黑白或其他几种颜色值进行比较,并将其四舍五入到最接近的颜色值
3. 将当前像素的误差分配给周围的像素,以便在下一次处理中进行补偿
4. 重复执行步骤2-3,直到所有像素都处理完毕
通过使用Floyd-Steinberg算法,我们可以生成适合黑白或其他几种色彩模式的图像,使其在黑白或其他几种颜色下仍然保留原始图像的细节和特征。
相关问题
用QT写出图片抖动算法,用于黑白或者黑白红、黑白黄三色墨水屏
以下是用QT实现Floyd-Steinberg算法的示例代码,用于将彩色图像转换为黑白或黑白红、黑白黄三色墨水屏:
```cpp
#include <QtGui>
#include <QtWidgets>
QImage ditherImage(const QImage& image, int colorMode)
{
QImage result(image.size(), QImage::Format_Mono);
int width = image.width();
int height = image.height();
int threshold = 128;
int red, green, blue, gray, newPixel;
int redThreshold, greenThreshold, blueThreshold;
int redValue, greenValue, blueValue;
// 判断颜色模式
switch (colorMode) {
case 1: // 黑白
threshold = 128;
break;
case 2: // 黑白红
threshold = 85;
break;
case 3: // 黑白黄
threshold = 170;
break;
default:
break;
}
// 遍历图像像素
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
QRgb pixel = image.pixel(x, y);
red = qRed(pixel);
green = qGreen(pixel);
blue = qBlue(pixel);
// 转换为灰度图像
gray = (qMax(qMax(red, green), blue) + qMin(qMin(red, green), blue)) / 2;
// 根据阈值进行二值化处理
if (gray > threshold) {
newPixel = 1;
} else {
newPixel = 0;
}
// 更新像素值
result.setPixel(x, y, newPixel);
// 计算误差扩散
if (newPixel == 0) {
redValue = red - 0;
greenValue = green - 0;
blueValue = blue - 0;
} else {
redValue = red - 255;
greenValue = green - 255;
blueValue = blue - 255;
}
// 分配误差值给周围的像素
if (x < width - 1) {
redThreshold = qRed(result.pixel(x + 1, y)) + redValue * 7 / 16;
greenThreshold = qGreen(result.pixel(x + 1, y)) + greenValue * 7 / 16;
blueThreshold = qBlue(result.pixel(x + 1, y)) + blueValue * 7 / 16;
result.setPixel(x + 1, y, qRgb(redThreshold, greenThreshold, blueThreshold));
}
if (x > 0 && y < height - 1) {
redThreshold = qRed(result.pixel(x - 1, y + 1)) + redValue * 3 / 16;
greenThreshold = qGreen(result.pixel(x - 1, y + 1)) + greenValue * 3 / 16;
blueThreshold = qBlue(result.pixel(x - 1, y + 1)) + blueValue * 3 / 16;
result.setPixel(x - 1, y + 1, qRgb(redThreshold, greenThreshold, blueThreshold));
}
if (y < height - 1) {
redThreshold = qRed(result.pixel(x, y + 1)) + redValue * 5 / 16;
greenThreshold = qGreen(result.pixel(x, y + 1)) + greenValue * 5 / 16;
blueThreshold = qBlue(result.pixel(x, y + 1)) + blueValue * 5 / 16;
result.setPixel(x, y + 1, qRgb(redThreshold, greenThreshold, blueThreshold));
}
if (x < width - 1 && y < height - 1) {
redThreshold = qRed(result.pixel(x + 1, y + 1)) + redValue * 1 / 16;
greenThreshold = qGreen(result.pixel(x + 1, y + 1)) + greenValue * 1 / 16;
blueThreshold = qBlue(result.pixel(x + 1, y + 1)) + blueValue * 1 / 16;
result.setPixel(x + 1, y + 1, qRgb(redThreshold, greenThreshold, blueThreshold));
}
}
}
return result;
}
```
在使用时,可以通过调用`ditherImage()`函数将彩色图像转换为黑白或黑白红、黑白黄三色墨水屏。其中,`image`参数是输入图像,`colorMode`参数指定颜色模式(1代表黑白,2代表黑白红,3代表黑白黄),返回值是输出图像。
用QT写出图片抖动算法,用于黑白或者黑白红、黑白黄三色墨水屏,并且适用于任何图片
好的,我可以给您提供一个简单的图片抖动算法实现,使用QT编写。您可以根据需要进一步优化算法。
算法步骤如下:
1. 读取待处理的图片。
2. 将彩色图像转换为灰度图像,可以使用以下公式:
gray = 0.299 * red + 0.587 * green + 0.114 * blue
其中,red、green、blue 分别表示红、绿、蓝三个通道的像素值,gray 表示灰度值。
3. 对每个像素点进行处理,将其灰度值四舍五入为 0 或 255。
4. 计算当前像素点的误差值,即当前像素点的灰度值与四舍五入后的灰度值之差。
5. 将误差值按照一定的比例分配到周围的像素点上,以达到抖动效果。
6. 将处理后的像素值保存为新的图片。
以下是实现代码:
```c++
#include <QImage>
void dithering(QImage& image)
{
int width = image.width();
int height = image.height();
// 转换为灰度图像
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
QColor color = image.pixelColor(x, y);
int gray = 0.299 * color.red() + 0.587 * color.green() + 0.114 * color.blue();
image.setPixelColor(x, y, QColor(gray, gray, gray));
}
}
// 处理每个像素点
for (int y = 0; y < height; y++) {
for (int x = 0; x < width; x++) {
QColor color = image.pixelColor(x, y);
int gray = color.red();
// 四舍五入为 0 或 255
int value = gray < 128 ? 0 : 255;
image.setPixelColor(x, y, QColor(value, value, value));
// 计算误差值
int error = gray - value;
// 分配误差值到周围的像素点
if (x < width - 1) {
QColor color = image.pixelColor(x + 1, y);
color.setRed(color.red() + error * 7 / 16);
image.setPixelColor(x + 1, y, color);
}
if (x > 0 && y < height - 1) {
QColor color = image.pixelColor(x - 1, y + 1);
color.setRed(color.red() + error * 3 / 16);
image.setPixelColor(x - 1, y + 1, color);
}
if (y < height - 1) {
QColor color = image.pixelColor(x, y + 1);
color.setRed(color.red() + error * 5 / 16);
image.setPixelColor(x, y + 1, color);
}
if (x < width - 1 && y < height - 1) {
QColor color = image.pixelColor(x + 1, y + 1);
color.setRed(color.red() + error * 1 / 16);
image.setPixelColor(x + 1, y + 1, color);
}
}
}
}
```
您可以将此函数添加到您的项目中,并在需要的地方调用它来进行图片抖动处理。
阅读全文
相关推荐
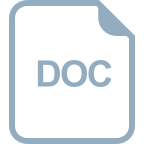
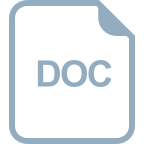
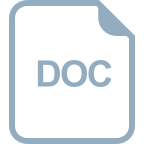
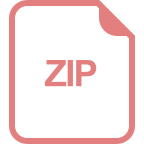
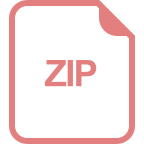
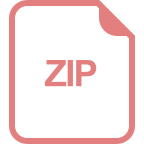
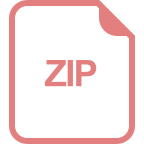
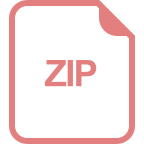
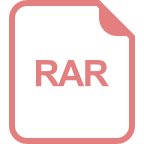
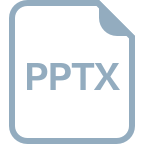
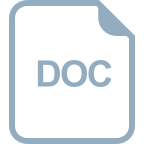
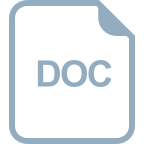
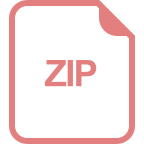
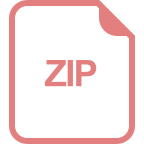
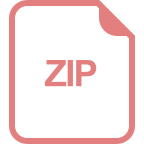
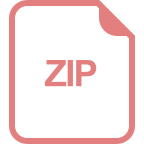
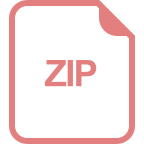