为某公司编写一个工资支付系统,用于计算某一类员工的月薪。该公司共有四类员工:领固定月薪的(SalariedEmployee);计时取酬的(HourlyEmployee,如果一月工时超过160小时,则还需对额外的工时支付加班费);按销售额提成(CommissionEmployee)的和带底薪并按销售额提成的(BasePlusCommissionEmployee),其继承层次结构如下所示。已知每类员工均有表示员工工号、姓名和出生年月的属性,和用于计算员工月薪的方法。创建一个Employee变量数组,保存Employee类层次结构中每个具体类对象的引用,对每个Employee显示其工号、姓名、出生年月和月收入,如果当月是Employee的生日所在的月份,则还另发给他100月作为红包。用Java写出源码
时间: 2024-02-17 17:05:32 浏览: 71
下面是用Java实现的工资支付系统代码,包括Employee类及其子类SalariedEmployee、HourlyEmployee、CommissionEmployee和BasePlusCommissionEmployee:
```java
import java.util.Date;
public abstract class Employee {
private String empId;
private String empName;
private Date birthDate;
public Employee(String empId, String empName, Date birthDate) {
this.empId = empId;
this.empName = empName;
this.birthDate = birthDate;
}
public abstract double getMonthlySalary();
public String getEmpId() {
return empId;
}
public void setEmpId(String empId) {
this.empId = empId;
}
public String getEmpName() {
return empName;
}
public void setEmpName(String empName) {
this.empName = empName;
}
public Date getBirthDate() {
return birthDate;
}
public void setBirthDate(Date birthDate) {
this.birthDate = birthDate;
}
public boolean isBirthday() {
Date today = new Date();
return (birthDate.getMonth() == today.getMonth());
}
public double getBirthdayBonus() {
if (isBirthday()) {
return 100;
} else {
return 0;
}
}
}
class SalariedEmployee extends Employee {
private double monthlySalary;
public SalariedEmployee(String empId, String empName, Date birthDate, double monthlySalary) {
super(empId, empName, birthDate);
this.monthlySalary = monthlySalary;
}
public double getMonthlySalary() {
return monthlySalary + getBirthdayBonus();
}
public double getMonthlySalaryWithoutBonus() {
return monthlySalary;
}
}
class HourlyEmployee extends Employee {
private double hourlyWage;
private double hoursWorked;
public HourlyEmployee(String empId, String empName, Date birthDate, double hourlyWage, double hoursWorked) {
super(empId, empName, birthDate);
this.hourlyWage = hourlyWage;
this.hoursWorked = hoursWorked;
}
public double getMonthlySalary() {
double overtimePay = 0;
if (hoursWorked > 160) {
overtimePay = (hoursWorked - 160) * hourlyWage * 1.5;
hoursWorked = 160;
}
double monthlySalary = hourlyWage * hoursWorked + overtimePay;
return monthlySalary + getBirthdayBonus();
}
public double getMonthlySalaryWithoutBonus() {
double overtimePay = 0;
if (hoursWorked > 160) {
overtimePay = (hoursWorked - 160) * hourlyWage * 1.5;
hoursWorked = 160;
}
double monthlySalary = hourlyWage * hoursWorked + overtimePay;
return monthlySalary;
}
}
class CommissionEmployee extends Employee {
private double commissionRate;
private double grossSales;
public CommissionEmployee(String empId, String empName, Date birthDate, double commissionRate, double grossSales) {
super(empId, empName, birthDate);
this.commissionRate = commissionRate;
this.grossSales = grossSales;
}
public double getMonthlySalary() {
double monthlySalary = commissionRate * grossSales;
return monthlySalary + getBirthdayBonus();
}
public double getMonthlySalaryWithoutBonus() {
double monthlySalary = commissionRate * grossSales;
return monthlySalary;
}
}
class BasePlusCommissionEmployee extends CommissionEmployee {
private double baseSalary;
public BasePlusCommissionEmployee(String empId, String empName, Date birthDate, double commissionRate, double grossSales, double baseSalary) {
super(empId, empName, birthDate, commissionRate, grossSales);
this.baseSalary = baseSalary;
}
public double getMonthlySalary() {
double monthlySalary = super.getMonthlySalary() + baseSalary;
return monthlySalary + getBirthdayBonus();
}
public double getMonthlySalaryWithoutBonus() {
double monthlySalary = super.getMonthlySalary() + baseSalary;
return monthlySalary;
}
}
public class PayrollSystem {
public static void main(String[] args) {
Date birthDate1 = new Date(2000, 1, 1);
Employee employee1 = new SalariedEmployee("001", "John", birthDate1, 5000);
Date birthDate2 = new Date(1995, 2, 1);
Employee employee2 = new HourlyEmployee("002", "Mary", birthDate2, 20, 180);
Date birthDate3 = new Date(1990, 3, 1);
Employee employee3 = new CommissionEmployee("003", "Tom", birthDate3, 0.1, 20000);
Date birthDate4 = new Date(1985, 4, 1);
Employee employee4 = new BasePlusCommissionEmployee("004", "Lisa", birthDate4, 0.1, 30000, 5000);
Employee[] employees = new Employee[]{employee1, employee2, employee3, employee4};
for (Employee employee : employees) {
System.out.println("Employee ID: " + employee.getEmpId());
System.out.println("Employee Name: " + employee.getEmpName());
System.out.println("Birth Date: " + employee.getBirthDate().toString());
System.out.println("Monthly Salary: " + employee.getMonthlySalary());
System.out.println();
}
}
}
```
在这个工资支付系统中,Employee类为抽象类,其中包含表示员工工号、姓名和出生年月的属性,以及用于计算员工月薪的抽象方法getMonthlySalary()。该类还包含isBirthday()和getBirthdayBonus()方法,用于判断当月是否是员工的生日所在的月份,并返回生日红包的金额。
SalariedEmployee、HourlyEmployee、CommissionEmployee和BasePlusCommissionEmployee类是Employee类的子类,它们分别表示领固定月薪、计时取酬、按销售额提成和带底薪并按销售额提成的员工。这些子类实现了getMonthlySalary()方法,根据不同的计算公式返回员工的月薪,同时调用父类的getBirthdayBonus()方法计算生日红包。
PayrollSystem类是一个测试类,其中创建了四个具体的员工对象,并将它们保存在一个Employee类型的数组中。然后,遍历该数组,对每个员工显示其工号、姓名、出生年月和月收入,其中如果当月是该员工的生日所在的月份,则还另发给他100元作为红包。
阅读全文
相关推荐














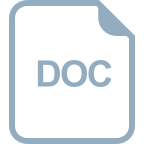
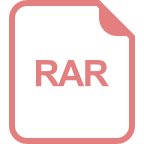
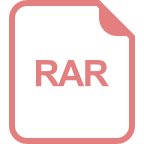

