vue div中使用v-for循环,显示每个对象的姓名,类似item.name,给item.name所在的div绑定click事件,点击item.name如何准确的定位鼠标所在位置
时间: 2024-02-18 22:00:48 浏览: 22
在Vue的v-for循环中,可以通过v-on指令来绑定click事件,并且使用$event对象来获取鼠标点击的位置。具体的代码如下:
```html
<template>
<div>
<div v-for="(item, index) in itemList" :key="index" @click="handleClick($event)">
{{ item.name }}
</div>
</div>
</template>
<script>
export default {
data() {
return {
itemList: [
{ name: '张三' },
{ name: '李四' },
{ name: '王五' }
]
}
},
methods: {
handleClick(event) {
const x = event.clientX
const y = event.clientY
console.log(`鼠标点击的位置:(${x}, ${y})`)
}
}
}
</script>
```
在上面的代码中,我们使用v-for循环来显示每个对象的姓名,并且给每个div绑定了click事件。在click事件中,我们通过$event对象获取鼠标点击的位置,并且输出到控制台中。
相关问题
如何在VUE v-for循环中每个item节点动态绑定不同函数
你可以在v-for循环中,使用v-on指令动态绑定不同的函数。具体实现可以参考以下代码:
```html
<template>
<div>
<ul>
<li v-for="(item, index) in itemList" :key="index">
<button v-on:click="getFunction(index)">{{ item.name }}</button>
</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
itemList: [
{ name: '函数1' },
{ name: '函数2' },
{ name: '函数3' }
]
}
},
methods: {
getFunction(index) {
switch (index) {
case 0:
this.function1()
break
case 1:
this.function2()
break
case 2:
this.function3()
break
default:
break
}
},
function1() {
console.log('执行函数1')
},
function2() {
console.log('执行函数2')
},
function3() {
console.log('执行函数3')
}
}
}
</script>
```
在上述代码中,我们使用v-for指令循环渲染出一个列表,每个列表项绑定了一个点击事件,事件名是动态生成的。在点击事件中,我们使用了switch语句来判断点击的是哪个列表项,然后调用对应的函数。这样就可以在v-for循环中每个item节点动态绑定不同函数了。
VUE v-for循环数组arr,div中显示对象中的某一值item.name,div横向排列,超出设定的长度时,div则自动换行,点击某一item.name时如何确定点击的元素是第几行的
要实现这个功能,你可以使用 Vue 的计算属性来计算每个 item 在数组中的索引以及所在的行数。具体操作步骤如下:
1. 在模板中使用 v-for 循环数组 arr,将每个 item 显示在一个 div 中,并绑定一个 click 事件。
```html
<template>
<div>
<div v-for="(item, index) in arr" :key="item.id" @click="handleClick(item)">
{{ item.name }}
</div>
</div>
</template>
```
2. 在计算属性中计算每个 item 在数组中的索引以及所在的行数。首先,需要知道每行能容纳的 item 的数量,可以根据容器的宽度和每个 item 的宽度来计算。然后,可以根据每个 item 在数组中的索引和每行能容纳的 item 的数量来计算该 item 所在的行数。
```javascript
computed: {
itemsPerRow() {
// 计算每行能容纳的 item 的数量
const containerWidth = this.$refs.container.clientWidth;
const itemWidth = this.$refs.item[0].clientWidth;
return Math.floor(containerWidth / itemWidth);
},
indexedArr() {
// 给每个 item 添加索引和行数属性
return this.arr.map((item, index) => {
const rowIndex = Math.floor(index / this.itemsPerRow);
return {
...item,
index,
rowIndex
};
});
}
}
```
3. 在 handleClick 方法中,可以通过事件对象的 target 属性获取点击的元素,然后遍历 indexedArr 数组,找到该元素对应的 item,并获取该 item 的 rowIndex 属性即可。
```javascript
methods: {
handleClick(item) {
const clickedElement = event.target;
const index = Array.from(this.$refs.item).indexOf(clickedElement);
const clickedItem = this.indexedArr[index];
console.log(clickedItem.rowIndex);
}
}
```
注意,这里需要使用 Array.from 将类数组对象转换为数组,以便使用 indexOf 方法来查找点击的元素在数组中的索引。同时,需要在每个 div 中添加一个 ref 属性,以便在计算属性中获取每个 item 的宽度。
相关推荐
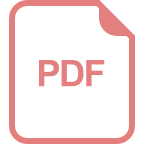
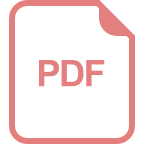












