C++实现DPM算法
时间: 2023-07-11 09:08:48 浏览: 157
DPM (Deformable Part-based Models) 算法是一种目标检测算法,它采用了分层的结构来描述目标,并通过学习模型参数来实现目标的快速检测。
以下是一个简单的 C++ 实现 DPM 算法的示例代码:
```cpp
#include <opencv2/opencv.hpp>
#include <opencv2/core/core.hpp>
#include <opencv2/highgui/highgui.hpp>
#include <opencv2/imgproc/imgproc.hpp>
#include <iostream>
#include <fstream>
#include <vector>
using namespace cv;
using namespace std;
struct Feature {
Mat image;
int x, y, w, h;
};
struct Model {
vector<Feature> features;
float score;
};
vector<Model> models;
void load_model(string filename) {
ifstream file(filename);
if (!file.is_open()) {
cerr << "Failed to open file: " << filename << endl;
exit(1);
}
string line;
while (getline(file, line)) {
Model model;
model.score = stof(line);
while (getline(file, line)) {
if (line.empty()) break;
Feature feature;
stringstream ss(line);
ss >> feature.x >> feature.y >> feature.w >> feature.h;
Mat image(feature.h, feature.w, CV_8UC1);
for (int y = 0; y < feature.h; y++) {
for (int x = 0; x < feature.w; x++) {
int pixel;
ss >> pixel;
image.at<uchar>(y, x) = pixel;
}
}
feature.image = image;
model.features.push_back(feature);
}
models.push_back(model);
}
}
float match_feature(const Feature& feature, const Mat& image, int x, int y) {
float sum = 0;
for (int j = 0; j < feature.h; j++) {
for (int i = 0; i < feature.w; i++) {
int pixel = image.at<uchar>(y + j, x + i);
sum += pixel * feature.image.at<uchar>(j, i);
}
}
return sum;
}
float match_model(const Model& model, const Mat& image, int x, int y) {
float sum = model.score;
for (const auto& feature : model.features) {
sum += match_feature(feature, image, x + feature.x, y + feature.y);
}
return sum;
}
vector<Rect> detect_objects(const Mat& image) {
vector<Rect> objects;
for (int y = 0; y < image.rows - 64; y += 8) {
for (int x = 0; x < image.cols - 64; x += 8) {
float max_score = -INFINITY;
for (const auto& model : models) {
float score = match_model(model, image, x, y);
if (score > max_score) {
max_score = score;
}
}
if (max_score > 0.5) {
objects.push_back(Rect(x, y, 64, 64));
}
}
}
return objects;
}
int main() {
load_model("model.txt");
Mat image = imread("image.jpg", IMREAD_GRAYSCALE);
vector<Rect> objects = detect_objects(image);
Mat result;
cvtColor(image, result, COLOR_GRAY2BGR);
for (const auto& object : objects) {
rectangle(result, object, Scalar(0, 0, 255), 2);
}
imshow("Result", result);
waitKey(0);
return 0;
}
```
上述代码中,我们首先定义了 `Feature` 和 `Model` 结构体,分别表示特征和模型。然后,我们通过 `load_model` 函数从文件中加载模型,并将其存储在 `models` 变量中。
接着,我们实现了 `match_feature` 和 `match_model` 函数,用于计算特征和模型之间的相似度得分。最后,我们实现了 `detect_objects` 函数,用于在图像中检测目标。
在 `main` 函数中,我们首先调用 `load_model` 函数加载模型,然后读取待检测的图像,并调用 `detect_objects` 函数来检测目标。最后,我们在图像上绘制检测结果,并显示出来。
需要注意的是,上述代码只是一个简单的示例,实际应用中还需要进行许多优化和改进,以提高检测的准确率和效率。
阅读全文
相关推荐
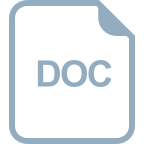
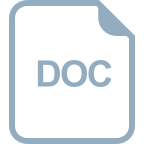
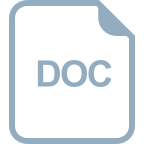
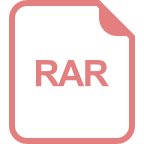
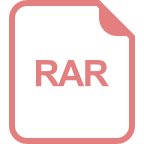
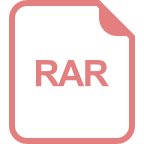
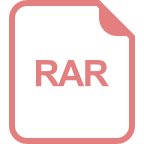
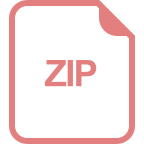
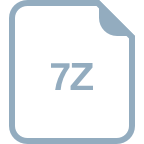
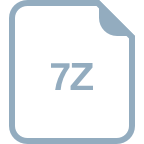
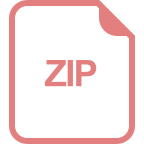
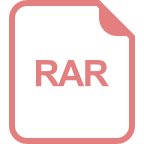
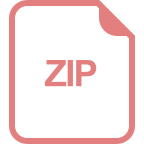
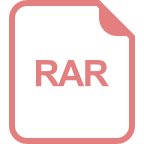
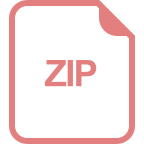
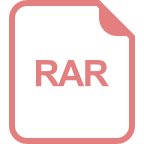
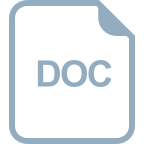

