springboot hibernate jsp
时间: 2023-10-26 21:20:21 浏览: 141
Spring Boot 是一个用于创建独立运行的Spring应用程序的框架。Hibernate 是一个流行的Java ORM框架,用于将Java对象映射到关系数据库中。JSP(JavaServer Pages)是一种用于创建动态Web页面的Java技术。
在使用Spring Boot和Hibernate时,可以通过注解来轻松配置和使用Hibernate。同时,Spring Boot也提供了对JSP的支持,可以使用Thymeleaf、Freemarker等模板引擎来创建动态页面。
以下是一个基于Spring Boot、Hibernate和JSP的示例项目:
1. 配置pom.xml文件,添加相关依赖
```xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.apache.tomcat.embed</groupId>
<artifactId>tomcat-embed-jasper</artifactId>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
</dependency>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-validator</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-validation</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
```
2. 创建实体类User,使用Hibernate注解进行映射
```java
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@NotBlank
@Size(min = 3, max = 50)
private String username;
@NotBlank
@Size(max = 120)
private String password;
// getters and setters
}
```
3. 创建数据访问层Repository,继承自JpaRepository
```java
public interface UserRepository extends JpaRepository<User, Long> {
Optional<User> findByUsername(String username);
}
```
4. 创建控制层Controller,使用Spring MVC注解进行配置
```java
@Controller
@RequestMapping("/users")
public class UserController {
@Autowired
private UserRepository userRepository;
@GetMapping
public String list(Model model) {
model.addAttribute("users", userRepository.findAll());
return "users/list";
}
@GetMapping("/{id}")
public String view(@PathVariable("id") Long id, Model model) {
User user = userRepository.findById(id)
.orElseThrow(() -> new IllegalArgumentException("Invalid user Id:" + id));
model.addAttribute("user", user);
return "users/view";
}
@GetMapping("/new")
public String createForm(Model model) {
model.addAttribute("user", new User());
return "users/create";
}
@PostMapping
public String create(@Valid User user, BindingResult result) {
if (result.hasErrors()) {
return "users/create";
}
userRepository.save(user);
return "redirect:/users";
}
@GetMapping("/{id}/edit")
public String updateForm(@PathVariable("id") Long id, Model model) {
User user = userRepository.findById(id)
.orElseThrow(() -> new IllegalArgumentException("Invalid user Id:" + id));
model.addAttribute("user", user);
return "users/update";
}
@PostMapping("/{id}")
public String update(@PathVariable("id") Long id, @Valid User user, BindingResult result) {
if (result.hasErrors()) {
user.setId(id);
return "users/update";
}
userRepository.save(user);
return "redirect:/users";
}
@DeleteMapping("/{id}")
public String delete(@PathVariable("id") Long id) {
userRepository.deleteById(id);
return "redirect:/users";
}
}
```
5. 创建视图页面,使用JSP模板引擎进行渲染
```html
<!-- /src/main/resources/templates/users/list.jsp -->
<table>
<thead>
<tr>
<th>Username</th>
<th></th>
</tr>
</thead>
<tbody>
<c:forEach items="${users}" var="user">
<tr>
<td>${user.username}</td>
<td>
<a href="<c:url value='/users/${user.id}'/>">View</a>
<a href="<c:url value='/users/${user.id}/edit'/>">Edit</a>
<form action="<c:url value='/users/${user.id}'/>" method="post">
<input type="hidden" name="_method" value="delete"/>
<input type="submit" value="Delete"/>
</form>
</td>
</tr>
</c:forEach>
</tbody>
</table>
<a href="<c:url value='/users/new'/>">New User</a>
<!-- /src/main/resources/templates/users/view.jsp -->
<h1>${user.username}</h1>
<a href="<c:url value='/users/${user.id}/edit'/>">Edit</a>
<form action="<c:url value='/users/${user.id}'/>" method="post">
<input type="hidden" name="_method" value="delete"/>
<input type="submit" value="Delete"/>
</form>
<!-- /src/main/resources/templates/users/create.jsp -->
<h1>Create User</h1>
<form action="<c:url value='/users'/>" method="post">
<div>
<label for="username">Username:</label>
<input type="text" id="username" name="username" value="${user.username}" required/>
<span th:if="${#fields.hasErrors('username')}" th:errors="*{username}"></span>
</div>
<div>
<label for="password">Password:</label>
<input type="password" id="password" name="password" value="${user.password}" required/>
<span th:if="${#fields.hasErrors('password')}" th:errors="*{password}"></span>
</div>
<div>
<button type="submit">Create</button>
</div>
</form>
<!-- /src/main/resources/templates/users/update.jsp -->
<h1>Edit User</h1>
<form action="<c:url value='/users/${user.id}'/>" method="post">
<input type="hidden" name="_method" value="put"/>
<div>
<label for="username">Username:</label>
<input type="text" id="username" name="username" value="${user.username}" required/>
<span th:if="${#fields.hasErrors('username')}" th:errors="*{username}"></span>
</div>
<div>
<label for="password">Password:</label>
<input type="password" id="password" name="password" value="${user.password}" required/>
<span th:if="${#fields.hasErrors('password')}" th:errors="*{password}"></span>
</div>
<div>
<button type="submit">Update</button>
</div>
</form>
```
这就是一个基于Spring Boot、Hibernate和JSP的示例项目。通过使用注解配置,可以轻松地创建一个简单的Web应用程序。同时,使用Thymeleaf、Freemarker等模板引擎也可以很容易地替换JSP。
阅读全文
相关推荐
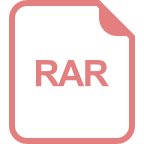
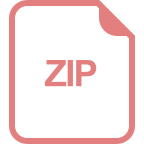
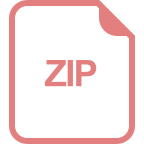











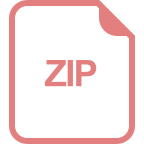
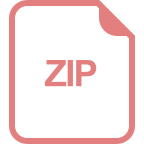
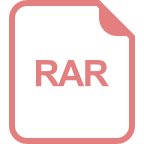
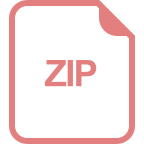
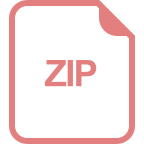