C动态链表指定节点位置插入
时间: 2023-07-11 20:20:29 浏览: 85
在C语言中,动态链表指定节点位置插入的操作可以分为以下几个步骤:
1. 定义一个新节点,并为其分配内存空间
```
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
```
2. 给新节点赋值
```
newNode->data = data;
```
3. 找到待插入节点的前一个节点
```
struct Node* prevNode = head;
for (int i = 1; i < pos - 1; i++) {
prevNode = prevNode->next;
}
```
这里假设链表头节点为`head`,待插入位置为`pos`。
4. 将新节点插入到链表中
```
newNode->next = prevNode->next;
prevNode->next = newNode;
```
完整代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
void insert(struct Node** head, int data, int pos) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = data;
newNode->next = NULL;
if (pos == 1) {
newNode->next = *head;
*head = newNode;
return;
}
struct Node* prevNode = *head;
for (int i = 1; i < pos - 1; i++) {
prevNode = prevNode->next;
}
newNode->next = prevNode->next;
prevNode->next = newNode;
}
void printList(struct Node* node) {
while (node) {
printf("%d ", node->data);
node = node->next;
}
printf("\n");
}
int main() {
struct Node* head = NULL;
insert(&head, 1, 1);
insert(&head, 2, 2);
insert(&head, 4, 3);
insert(&head, 3, 3);
printList(head);
return 0;
}
```
输出结果为:
```
1 2 3 4
```
阅读全文
相关推荐

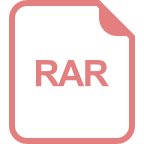
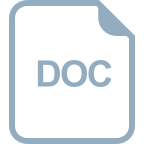
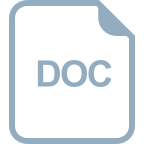
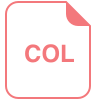









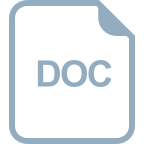
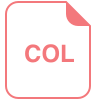