用c语言实现链表的查找,插入,删除操作
时间: 2024-10-22 19:17:31 浏览: 26
在C语言中,链表是一种常用的数据结构,可以动态地存储数据并支持高效的插入、删除操作。以下是链表的基本操作:
1. **查找操作** (查找元素)
- 定义查找函数,如`find_node`,它接受链表头指针和目标值作为参数。
```c
struct Node* find_node(struct Node* head, int data) {
struct Node* current = head;
while (current != NULL && current->data != data) {
current = current->next;
}
return current;
}
```
函数遍历链表,如果找到匹配的节点则返回该节点,否则返回NULL。
2. **插入操作** (在特定位置插入新节点)
- 分别有三种插入方式:在头部、尾部和指定位置。
- 插入头部:`insert_at_head`
- 插入尾部:`insert_at_tail`
- 插入指定位置:需要先找到目标位置的前一个节点,然后插入
```c
void insert_at_head(struct Node** head, int data) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = data;
newNode->next = *head;
*head = newNode;
}
// 插入尾部类似,只需将新节点的next指向NULL
void insert_at_tail(struct Node** head, int data) {
if (*head == NULL) {
insert_at_head(head, data);
} else {
struct Node* last = *head;
while (last->next != NULL) {
last = last->next;
}
last->next = (struct Node*)malloc(sizeof(struct Node));
last->next->data = data;
last->next->next = NULL;
}
}
// 插入指定位置的辅助函数
struct Node* search_for_insertion_point(struct Node* head, int data, int target_data) {
struct Node* current = head;
while (current != NULL && current->data < target_data) {
current = current->next;
}
return current;
}
// 然后在`search_for_insertion_point`返回的节点后插入
void insert_at_position(struct Node** head, int data, int target_data) {
struct Node* insertionPoint = search_for_insertion_point(*head, data, target_data);
// ...
}
```
3. **删除操作** (删除某个节点)
- 删除头部节点:直接更新头指针
- 删除其他位置:同样需找到待删除节点的前一个节点,将其next指向下一个节点
```c
void delete_node(struct Node** head, int data) {
if (*head == NULL) {
return;
}
if ((*head)->data == data) { // 如果是头部
struct Node* temp = *head;
*head = (*head)->next;
free(temp);
} else {
struct Node* current = *head;
while (current->next != NULL && current->next->data != data) {
current = current->next;
}
if (current->next != NULL) {
struct Node* temp = current->next;
current->next = temp->next;
free(temp);
}
}
}
```
阅读全文
相关推荐
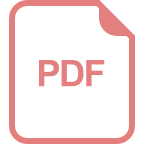
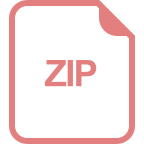
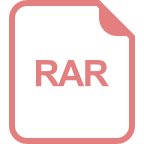
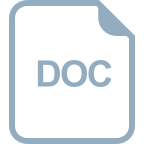
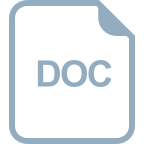

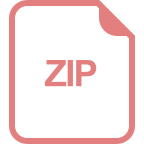
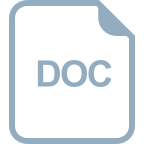
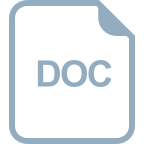
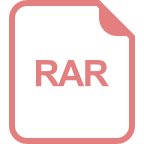
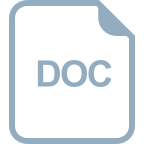
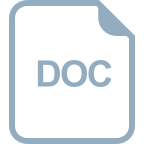
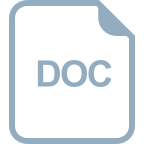
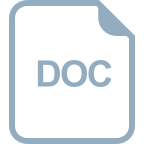
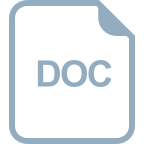
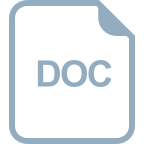
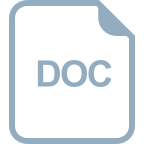
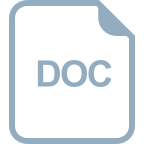
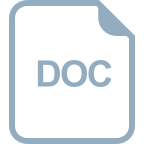