用python编写爬虫代码
时间: 2024-03-11 10:37:26 浏览: 17
好的,我可以给你一个简单的Python爬虫代码,它可以爬取一个网站的所有文章标题和链接。请确保你已经安装了Python和BeautifulSoup库。
```
import requests
from bs4 import BeautifulSoup
url = "https://www.example.com"
response = requests.get(url)
soup = BeautifulSoup(response.content, "html.parser")
articles = soup.find_all("article")
for article in articles:
title = article.find("h2").text.strip()
link = article.find("a")["href"]
print(title)
print(link)
```
这段代码中,我们首先使用 requests 库获取网站的 HTML 内容,然后使用 BeautifulSoup 库解析 HTML。我们使用 find_all() 方法查找所有文章,并使用 find() 方法查找标题和链接。最后,我们打印出所有文章的标题和链接。
相关问题
python编写爬虫代码
在 Python 中编写爬虫代码,需要使用到一些 Python 的库和模块,如 requests 库和 BeautifulSoup 模块。
步骤如下:
1. 导入所需的库和模块。
2. 使用 requests 库发送 HTTP 请求,并获取网页的源代码。
3. 使用 BeautifulSoup 解析网页源代码,并提取所需的信息。
4. 将提取到的信息存储到本地或者数据库中。
例如,以下是一个简单的 Python 爬虫代码,它可以提取网页的标题:
```python
import requests
from bs4 import BeautifulSoup
# 发送 HTTP 请求并获取网页源代码
response = requests.get('http://www.example.com')
html = response.text
# 使用 BeautifulSoup 解析网页源代码
soup = BeautifulSoup(html, 'html.parser')
# 提取标题
title = soup.title.string
print(title)
```
在这个例子中,我们使用了 requests 库来发送 HTTP 请求并获取网页的源代码,然后使用 BeautifulSoup 解析网页源代码,最后提取标题并打印出来。
用Python编写一个爬虫代码
### 回答1:
我可以为您提供一些有关如何使用Python编写爬虫代码的指导。首先,需要使用Python的urllib和BeautifulSoup库来获取网页内容。然后,可以使用正则表达式来提取特定的内容。最后,可以使用requests库发送HTTP请求,来获取页面内容。
### 回答2:
爬虫是指通过网络爬取并提取数据的程序。Python是一门强大的编程语言,可以很方便地编写爬虫代码。
下面是一个简单的用Python编写的爬虫代码示例:
```python
import requests
from bs4 import BeautifulSoup
# 设置请求头信息
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/89.0.4389.82 Safari/537.36'
}
# 发送请求并获取网页内容
def get_html(url):
response = requests.get(url, headers=headers)
if response.status_code == 200:
return response.text
else:
return None
# 解析网页内容并提取数据
def parse_html(html):
soup = BeautifulSoup(html, 'lxml')
# 这里以提取网页中的标题为例
titles = soup.select('h3') # 使用CSS选择器提取所有的h3标签
for title in titles:
print(title.text)
# 主函数
def main():
url = 'https://www.example.com/' # 设置待爬取的网页地址
html = get_html(url)
if html:
parse_html(html)
if __name__ == '__main__':
main()
```
上面的示例代码使用了第三方库`requests`发送HTTP请求,使用了`BeautifulSoup`库解析网页内容。爬虫的基本步骤是先发送请求获取网页内容,然后解析网页内容提取需要的数据。
这只是一个简单的爬虫示例,实际的爬虫代码可能会更复杂,需要根据具体的需求进行设计和调整。另外,编写爬虫代码时需要遵守网站的爬虫规则,尊重网站的合法权益,并避免对网站造成不必要的负载和干扰。
### 回答3:
爬虫(Spider)是指通过网络自动获取网页数据的程序。用Python编写爬虫代码可以使用requests库和BeautifulSoup库来实现。
首先,我们需要安装requests库和BeautifulSoup库,可以使用pip指令在命令行中输入以下两条指令进行安装:
pip install requests
pip install beautifulsoup4
接下来,我们编写一个简单的爬虫代码来爬取一个网页上的数据。以爬取豆瓣电影页面为例:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://movie.douban.com/top250'
# 发送请求
response = requests.get(url)
# 解析网页内容
soup = BeautifulSoup(response.text, 'html.parser')
# 定位电影名称所在的标签
movie_tags = soup.find_all('div', class_='hd')
# 打印电影名称
for movie_tag in movie_tags:
movie_name = movie_tag.a.span.text
print(movie_name)
```
上述代码首先使用requests库发送了一个GET请求获取网页内容,然后用BeautifulSoup库对网页内容进行解析。使用soup.find_all方法定位包含电影名称的标签,并通过遍历打印出电影名称。
这只是一个简单的爬虫代码例子,实际中可能需要考虑更多的情况,比如网页动态加载、反爬机制等。另外,爬取网页数据时需要遵守相关网站的爬取规则,不得进行非法的抓取和滥用。
相关推荐
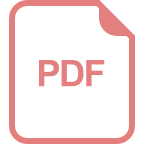
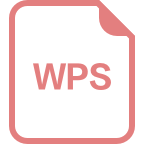












