用Java写一个ftp分片上传与合并的代码
时间: 2024-02-21 11:58:11 浏览: 28
以下是一个用Java实现FTP分片上传和合并的示例代码:
```
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.ArrayList;
import java.util.List;
import org.apache.commons.net.ftp.FTP;
import org.apache.commons.net.ftp.FTPClient;
import org.apache.commons.net.ftp.FTPReply;
public class FtpUpload {
public static void main(String[] args) {
String server = "ftp.example.com";
int port = 21;
String user = "username";
String password = "password";
String remotePath = "/upload/";
String localPath = "/path/to/local/file";
String fileName = "example.txt";
int bufferSize = 1024 * 1024;
int chunkSize = 1024 * 1024 * 10; // 10MB
try {
FTPClient ftp = new FTPClient();
ftp.connect(server, port);
int reply = ftp.getReplyCode();
if (!FTPReply.isPositiveCompletion(reply)) {
ftp.disconnect();
throw new IOException("FTP server refused connection.");
}
ftp.login(user, password);
ftp.setFileType(FTP.BINARY_FILE_TYPE);
ftp.enterLocalPassiveMode();
ftp.changeWorkingDirectory(remotePath);
File file = new File(localPath, fileName);
long fileSize = file.length();
int chunks = (int) Math.ceil((double) fileSize / chunkSize);
System.out.println("File size: " + fileSize + " bytes");
System.out.println("Chunk size: " + chunkSize + " bytes");
System.out.println("Number of chunks: " + chunks);
List<String> chunkFiles = new ArrayList<String>();
InputStream input = new FileInputStream(file);
byte[] buffer = new byte[bufferSize];
int bytesRead = 0;
int chunkNumber = 1;
while ((bytesRead = input.read(buffer)) != -1) {
String chunkFileName = fileName + ".part" + chunkNumber;
OutputStream output = new FileOutputStream(new File(localPath, chunkFileName));
output.write(buffer, 0, bytesRead);
output.close();
chunkFiles.add(chunkFileName);
chunkNumber++;
}
input.close();
for (int i = 0; i < chunks; i++) {
String chunkFileName = chunkFiles.get(i);
File chunkFile = new File(localPath, chunkFileName);
input = new FileInputStream(chunkFile);
ftp.storeFile(chunkFileName, input);
input.close();
chunkFile.delete();
System.out.println("Uploaded chunk " + (i + 1) + "/" + chunks);
}
ftp.disconnect();
System.out.println("All chunks uploaded.");
// merge chunks
OutputStream output = new FileOutputStream(new File(localPath, fileName));
for (int i = 0; i < chunks; i++) {
String chunkFileName = fileName + ".part" + (i + 1);
input = new FileInputStream(new File(localPath, chunkFileName));
buffer = new byte[bufferSize];
while ((bytesRead = input.read(buffer)) != -1) {
output.write(buffer, 0, bytesRead);
}
input.close();
}
output.close();
System.out.println("File merged.");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
该代码通过Apache Commons Net库实现FTP客户端连接和文件上传,将大文件分割成多个小文件进行上传,并在上传完成后将它们合并成一个完整的文件。
相关推荐
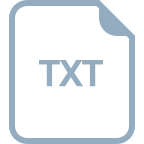














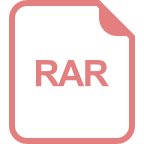