用java写一个下载ftp服务器文件的代码
时间: 2024-01-22 17:21:19 浏览: 82
可以使用 Apache Commons Net 库来实现FTP文件下载。以下是一个简单的示例代码,仅供参考:
```java
import org.apache.commons.net.ftp.FTP;
import org.apache.commons.net.ftp.FTPClient;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
public class FTPDownloadExample {
public static void main(String[] args) {
String server = "ftp.example.com";
int port = 21;
String user = "username";
String password = "password";
String remoteFilePath = "/path/to/file.txt";
String localFilePath = "/path/to/save/file.txt";
FTPClient ftpClient = new FTPClient();
try {
ftpClient.connect(server, port);
ftpClient.login(user, password);
ftpClient.enterLocalPassiveMode();
ftpClient.setFileType(FTP.BINARY_FILE_TYPE);
OutputStream outputStream = new FileOutputStream(localFilePath);
boolean success = ftpClient.retrieveFile(remoteFilePath, outputStream);
outputStream.close();
if (success) {
System.out.println("File downloaded successfully.");
} else {
System.out.println("Failed to download file.");
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (ftpClient.isConnected()) {
ftpClient.logout();
ftpClient.disconnect();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
在本示例中,我们使用 `FTPClient` 连接到FTP服务器,登录并进入被动模式。然后,我们将文件类型设置为二进制,从远程FTP服务器下载文件,将其保存到本地文件系统上,最后关闭连接。请注意,如果文件下载成功,`retrieveFile` 方法将返回 `true`,否则返回 `false`。
阅读全文
相关推荐

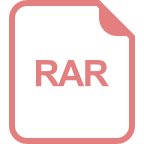












