使用java代码写一个ftp服务器下载文件功能,需要失败重新下载,返回结果
时间: 2023-08-31 08:44:03 浏览: 144
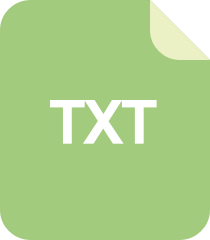
java下载ftp文件.txt
### 回答1:
好的,以下是使用Java代码编写FTP服务器下载文件功能,并包含失败重新下载和返回结果的示例:
```java
import org.apache.commons.net.ftp.FTP;
import org.apache.commons.net.ftp.FTPClient;
import java.io.*;
public class FTPDownloader {
public static void download(String server, int port, String user, String password,
String remoteFilePath, String localFilePath) throws IOException {
FTPClient ftpClient = new FTPClient();
ftpClient.connect(server, port);
ftpClient.login(user, password);
ftpClient.enterLocalPassiveMode();
ftpClient.setFileType(FTP.BINARY_FILE_TYPE);
File localFile = new File(localFilePath);
OutputStream outputStream = new BufferedOutputStream(new FileOutputStream(localFile));
boolean success = false;
while (!success) {
InputStream inputStream = ftpClient.retrieveFileStream(remoteFilePath);
if (inputStream != null) {
byte[] bytesArray = new byte[4096];
int bytesRead = -1;
while ((bytesRead = inputStream.read(bytesArray)) != -1) {
outputStream.write(bytesArray, 0, bytesRead);
}
success = ftpClient.completePendingCommand();
if (success) {
System.out.println("文件下载成功!");
}
}
if (!success) {
System.out.println("文件下载失败,将在5秒后尝试重新下载...");
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
outputStream.close();
ftpClient.logout();
ftpClient.disconnect();
}
public static void main(String[] args) {
String server = "ftp.example.com";
int port = 21;
String user = "username";
String password = "password";
String remoteFilePath = "/path/to/remote/file";
String localFilePath = "/path/to/local/file";
try {
download(server, port, user, password, remoteFilePath, localFilePath);
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这个示例代码使用了Apache Commons Net库来实现FTP文件下载。在while循环中,每次下载文件失败后都会等待5秒后再尝试重新下载,直到下载成功为止。成功下载后会打印出一条成功信息。最后,关闭输出流和FTP连接,并返回结果。
### 回答2:
使用Java代码编写一个FTP服务器下载文件功能,需要实现失败重新下载并返回结果的功能,可以参考以下步骤:
1. 导入相关的Java FTP库(例如commons-net)。
2. 创建一个FTP客户端对象,并连接到FTP服务器。
```java
FTPClient ftpClient = new FTPClient();
ftpClient.connect(server, port);
ftpClient.login(username, password);
```
3. 设置FTP连接的模式和传输类型。
```java
ftpClient.enterLocalPassiveMode();
ftpClient.setFileType(FTP.BINARY_FILE_TYPE);
```
4. 切换到FTP服务器上的工作目录。
```java
ftpClient.changeWorkingDirectory(remoteDirectory);
```
5. 定义本地文件的路径和文件名,以及在服务器上的文件路径和文件名。
```java
String localFile = "本地文件路径";
String remoteFile = "服务器文件路径";
```
6. 尝试从服务器下载文件。如果下载失败,则进行重新下载。
```java
boolean success = false;
while (!success) {
OutputStream outputStream = new BufferedOutputStream(new FileOutputStream(localFile));
if (ftpClient.retrieveFile(remoteFile, outputStream)) {
success = true;
} else {
// 下载失败,等待一段时间后重新下载
Thread.sleep(5000); // 等待5秒
}
outputStream.close();
}
```
7. 根据下载是否成功进行相应的处理。
```java
if (success) {
System.out.println("文件下载成功");
// 其他处理逻辑
} else {
System.out.println("文件下载失败");
// 其他处理逻辑
}
```
8. 最后关闭FTP连接。
```java
ftpClient.logout();
ftpClient.disconnect();
```
通过以上步骤,可以实现一个FTP服务器下载文件功能,并在下载失败时进行重新下载,并返回相应的结果。
### 回答3:
要通过Java代码实现一个FTP服务器下载文件的功能,需要按照以下步骤进行操作:
1. 导入FTP客户端库,如Apache Commons Net库。
2. 创建一个FTPClient对象,并设置服务器地址、端口、用户名和密码。
3. 连接到FTP服务器,使用`connect()`方法。
4. 登录FTP服务器,使用`login()`方法,传入用户名和密码。
5. 设置被动模式,使用`enterLocalPassiveMode()`方法,以便能够下载文件。
6. 检查本地目标路径是否存在,如果不存在则创建该路径。
7. 进入到服务器上文件所在的目录,使用`changeWorkingDirectory()`方法。
8. 检查远程文件是否存在,使用`listFiles()`方法,通过文件名来检索特定文件。
9. 如果文件存在,使用`retrieveFile()`方法下载文件到本地路径,并设置下载进度的回调接口。
10. 检查下载是否成功,如果成功则返回下载结果,否则重新下载。
11. 关闭FTP连接,使用`disconnect()`方法。
下面是一个简单的示例代码:
```java
import org.apache.commons.net.ftp.FTPClient;
import org.apache.commons.net.ftp.FTPFile;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class FTPDownloader {
public static void main(String[] args) {
String server = "ftp.example.com";
int port = 21;
String user = "ftpuser";
String password = "ftppassword";
String remoteFilePath = "/path/to/remote/file";
String localDirPath = "/path/to/local/directory";
FTPClient ftpClient = new FTPClient();
try {
ftpClient.connect(server, port);
ftpClient.login(user, password);
ftpClient.enterLocalPassiveMode();
Path localDir = Paths.get(localDirPath);
if (!Files.exists(localDir)) {
Files.createDirectories(localDir);
}
ftpClient.changeWorkingDirectory(remoteFilePath);
FTPFile[] files = ftpClient.listFiles();
for (FTPFile file : files) {
if (file.isFile()) {
String remoteFileName = file.getName();
String localFilePath = localDirPath + "/" + remoteFileName;
Path localFile = Paths.get(localFilePath);
// 如果本地文件已存在,跳过下载
if (!Files.exists(localFile)) {
try (OutputStream outputStream = new FileOutputStream(localFilePath)) {
ftpClient.retrieveFile(remoteFileName, outputStream);
}
}
// 检查下载是否成功
if (Files.exists(localFile)) {
System.out.println("文件下载成功:" + localFilePath);
} else {
System.out.println("文件下载失败:" + localFilePath);
}
}
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
ftpClient.disconnect();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
此代码将连接到FTP服务器,遍历指定目录中的文件,并在本地目录中下载这些文件。如果文件已经存在,则跳过下载。如果下载成功,则打印相应的结果。如果下载失败,则打印相应的错误信息。
阅读全文
相关推荐
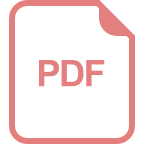
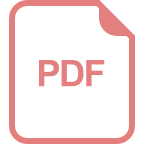















