用c程序给出“链式线性表”的创建 Create、显示 Display、插 入 Insert、删除 Delete、合并 Merge 接口函数
时间: 2024-02-01 18:03:54 浏览: 31
以下是链式线性表的创建、显示、插入、删除、合并的C语言实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表结构体
typedef struct Node {
int data; // 数据域
struct Node *next; // 指针域
} Node, *LinkedList;
// 初始化链表
LinkedList initList() {
LinkedList head = (LinkedList) malloc(sizeof(Node));
head->next = NULL;
return head;
}
// 打印链表
void displayList(LinkedList head) {
Node *p = head->next;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
// 在链表尾部插入元素
void insertList(LinkedList head, int data) {
Node *p = (Node *) malloc(sizeof(Node));
p->data = data;
p->next = NULL;
Node *q = head;
while (q->next != NULL) {
q = q->next;
}
q->next = p;
}
// 删除链表中第index个节点
void deleteList(LinkedList head, int index) {
int i = 0;
Node *p = head;
while (p->next != NULL && i < index - 1) {
p = p->next;
i++;
}
if (p->next == NULL || i > index - 1) {
printf("删除位置无效!\n");
return;
}
Node *q = p->next;
p->next = q->next;
free(q);
}
// 合并两个链表
LinkedList mergeList(LinkedList head1, LinkedList head2) {
Node *p = head1->next;
Node *q = head2->next;
Node *r = head1;
while (p != NULL && q != NULL) {
if (p->data <= q->data) {
r->next = p;
p = p->next;
} else {
r->next = q;
q = q->next;
}
r = r->next;
}
if (p != NULL) {
r->next = p;
}
if (q != NULL) {
r->next = q;
}
return head1;
}
int main() {
// 创建链表1
LinkedList head1 = initList();
insertList(head1, 10);
insertList(head1, 20);
insertList(head1, 30);
printf("链表1: ");
displayList(head1);
// 创建链表2
LinkedList head2 = initList();
insertList(head2, 15);
insertList(head2, 25);
insertList(head2, 35);
printf("链表2: ");
displayList(head2);
// 合并链表1和链表2
LinkedList head = mergeList(head1, head2);
printf("合并后的链表: ");
displayList(head);
// 删除链表中第2个节点
deleteList(head, 2);
printf("删除第2个节点后的链表: ");
displayList(head);
return 0;
}
```
阅读全文
相关推荐
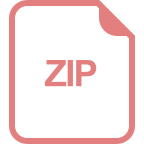
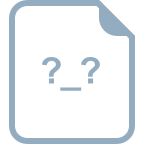
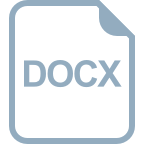
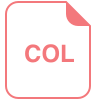








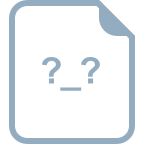