C语言判断字符串中,的个数
时间: 2023-11-28 10:48:24 浏览: 82
可以使用循环遍历字符串中的每个字符,当遇到空格或其他分隔符时,计数器加 1,最后得到字符串中单词的个数。
以下是一个简单的例子:
```c
#include <stdio.h>
#include <string.h>
int countWords(char *str) {
int count = 0;
int len = strlen(str);
int i;
for (i = 0; i < len; i++) {
if (str[i] == ' ' || str[i] == '\t' || str[i] == '\n') {
count++;
}
}
return count + 1; // 最后一个单词后面没有空格,需要加 1
}
int main() {
char str[] = "hello world, this is a test";
int count = countWords(str);
printf("Number of words: %d\n", count);
return 0;
}
```
输出:
```
Number of words: 6
```
相关问题
c语言判断字符串中,的个数
可以使用循环遍历字符串中的每个字符,当遇到空格或其他分隔符时,计数器加 1,最后得到字符串中单词的个数。
以下是一个简单的例子:
```c
#include <stdio.h>
#include <string.h>
int countWords(char *str) {
int count = 0;
int len = strlen(str);
int i;
for (i = 0; i < len; i++) {
if (str[i] == ' ' || str[i] == '\t' || str[i] == '\n') {
count++;
}
}
return count + 1; // 最后一个单词后面没有空格,需要加 1
}
int main() {
char str[] = "hello world, this is a test";
int count = countWords(str);
printf("Number of words: %d\n", count);
return 0;
}
```
输出:
```
Number of words: 6
```
c语言怎么判断字符串的个数
在C语言中,要计算字符串的个数通常是指字符数组或字符串常量的数量,但这里你可能想问的是如何统计字符串组成的数组或向量的元素个数。如果你有一个字符数组,比如`char strArray[] = {"str1", "str2", "str3"}`,你可以通过计数每个字符串结束符`\0`来确定字符串的个数。
下面是一个简单的示例:
```c
#include <stdio.h>
#include <string.h>
int count_strings(char *strArray[], int size) {
int count = 0;
for (int i = 0; i < size; i++) {
if (strArray[i][strlen(strArray[i])] == '\0') // 检查字符串是否以'\0'结尾
count++;
}
return count;
}
int main() {
char strings[] = {"hello", "", "world"};
int stringCount = count_strings(strings, sizeof(strings) / sizeof(strings[0]));
printf("字符串个数: %d\n", stringCount);
return 0;
}
```
在这个例子中,`count_strings`函数遍历数组并检查每个元素是否以空字符`\0`结束。如果是,就增加计数器。注意这里假设数组中每个元素都是有效的字符串,如果不是,这个简单的方法可能无法正确处理。
阅读全文
相关推荐
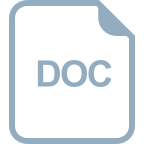
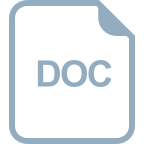
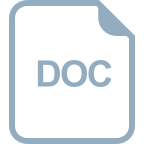













