现在有个需求 用到的语言是java 技术框架是mybatis 现在遇到的难点是实体类里面的属性都是对应不同表里面的列应该怎么映射
时间: 2023-03-21 13:01:27 浏览: 70
在MyBatis中,可以使用XML映射文件或注解方式来配置实体类属性和数据库表列之间的映射关系。以下是两种方式的示例:
1. 使用XML映射文件
(1)在实体类中定义属性,例如:
```java
public class User {
private Long id;
private String username;
private String password;
private String email;
// getter and setter methods
}
```
(2)在XML映射文件中定义SQL语句和参数映射关系,例如:
```xml
<mapper namespace="com.example.UserMapper">
<resultMap id="userResultMap" type="com.example.User">
<id column="id" property="id"/>
<result column="username" property="username"/>
<result column="password" property="password"/>
<result column="email" property="email"/>
</resultMap>
<select id="selectUserById" resultMap="userResultMap">
select id, username, password, email
from user
where id = #{id}
</select>
</mapper>
```
在这个XML映射文件中,使用`<resultMap>`标签来定义实体类和数据库表之间的映射关系,其中`id`标签用于定义主键列和实体类属性之间的映射关系,`result`标签用于定义非主键列和实体类属性之间的映射关系。
2. 使用注解方式
(1)在实体类中定义属性,例如:
```java
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "username")
private String username;
@Column(name = "password")
private String password;
@Column(name = "email")
private String email;
// getter and setter methods
}
```
(2)使用`@Table`、`@Column`、`@Id`、`@GeneratedValue`等注解来标记实体类和数据库表之间的映射关系,例如:
```java
@Table(name = "user")
public interface UserMapper {
@Select("select * from user where id = #{id}")
User selectUserById(@Param("id") Long id);
}
```
在这个示例中,使用`@Table`注解来指定实体类对应的数据库表,使用`@Column`注解来指定实体类属性对应的数据库表列,使用`@Id`和`@GeneratedValue`注解来指定实体类主键对应的数据库表列和生成方式,使用`@Select`注解来定义SQL语句,其中`#{id}`表示查询语句中的参数占位符,`@Param("id")`表示将参数名称为`id`的值绑定到SQL语句中的`#{id}`占位符上。
无论是使用XML映射文件还是注解方式,都需要确保实体类属性和数据库表列之间的映射关系正确,否则会导致数据读写错误或异常。
相关推荐
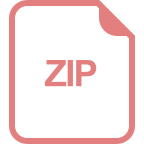














