vue使用组件,实现购物车功能
时间: 2023-11-23 17:33:04 浏览: 42
要实现购物车功能,可以考虑以下步骤:
1. 创建一个商品列表组件,用来展示所有可以购买的商品,每个商品需要有一个“加入购物车”的按钮。
2. 创建一个购物车组件,用来展示已经加入购物车的商品列表,每个商品需要有一个“删除”按钮和数量控制。
3. 在商品列表组件中,点击“加入购物车”按钮时,向父组件发送一个事件,将商品信息传递给购物车组件。
4. 在购物车组件中,处理接收到的商品信息,将其添加到已选商品列表中,如果已经存在,则数量+1,同时计算总价并展示。
5. 监听购物车组件中每个商品的数量变化事件,重新计算总价。
6. 在购物车组件中,点击“删除”按钮时,将对应商品从列表中删除,重新计算总价。
以上是一种简单的实现方式,具体代码实现可以参考以下示例:
```vue
<!-- 商品列表组件 -->
<template>
<div>
<div v-for="item in goodsList" :key="item.id">
<span>{{ item.name }}</span>
<button @click="addToCart(item)">加入购物车</button>
</div>
</div>
</template>
<script>
export default {
data() {
return {
goodsList: [
{ id: 1, name: '商品1', price: 100 },
{ id: 2, name: '商品2', price: 200 },
{ id: 3, name: '商品3', price: 300 }
]
}
},
methods: {
addToCart(item) {
this.$emit('add-to-cart', item)
}
}
}
</script>
<!-- 购物车组件 -->
<template>
<div>
<div v-for="item in cartList" :key="item.id">
<span>{{ item.name }}</span>
<button @click="removeFromCart(item)">删除</button>
<input type="number" v-model="item.count" @change="countChanged(item)">
</div>
<div>总价:{{ total }}</div>
</div>
</template>
<script>
export default {
data() {
return {
cartList: [],
total: 0
}
},
methods: {
addToCart(item) {
const index = this.cartList.findIndex(i => i.id === item.id)
if (index === -1) {
this.cartList.push({
id: item.id,
name: item.name,
price: item.price,
count: 1
})
} else {
this.cartList[index].count++
}
this.recalculateTotal()
},
removeFromCart(item) {
const index = this.cartList.findIndex(i => i.id === item.id)
if (index !== -1) {
this.cartList.splice(index, 1)
this.recalculateTotal()
}
},
countChanged(item) {
if (item.count <= 0) {
this.removeFromCart(item)
} else {
this.recalculateTotal()
}
},
recalculateTotal() {
this.total = this.cartList.reduce((sum, item) => {
return sum + item.price * item.count
}, 0)
}
}
}
</script>
```
在父组件中,将商品列表组件和购物车组件引入并注册为子组件,然后监听商品列表组件的“add-to-cart”事件,在事件回调函数中调用购物车组件的“addToCart”方法,将商品信息传递给购物车组件即可。
```vue
<template>
<div>
<goods-list @add-to-cart="addToCart"></goods-list>
<cart-list ref="cartList"></cart-list>
</div>
</template>
<script>
import GoodsList from './GoodsList.vue'
import CartList from './CartList.vue'
export default {
components: {
GoodsList,
CartList
},
methods: {
addToCart(item) {
this.$refs.cartList.addToCart(item)
}
}
}
</script>
```
以上代码仅供参考,具体实现方式可以根据实际需求进行调整。
相关推荐
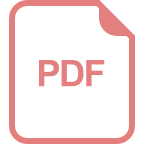
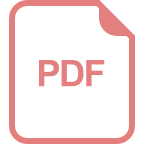
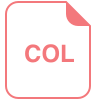
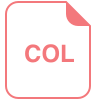
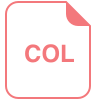
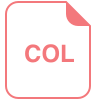










