能否使用Python语言实现一下FOIL算法
时间: 2024-04-17 15:04:53 浏览: 105
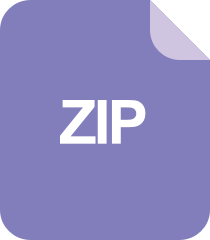
用Python实现的 一阶归纳学习器 (FOIL)算法_python_代码_下载

当然可以。下面是一个简单的Python实现:
```python
from itertools import combinations
class FOIL:
def __init__(self, data, target_attribute):
self.data = data
self.target_attribute = target_attribute
self.rule = None
def fit(self):
rules = []
attributes = list(self.data.columns)
attributes.remove(self.target_attribute)
while len(attributes) > 0:
best_rule = None
best_gain = -1
for attribute in attributes:
rule = (attribute, {})
for value in self.data[attribute].unique():
subset = self.data[self.data[attribute] == value]
value_count = len(subset)
if value_count == 0:
continue
positive_count = len(subset[subset[self.target_attribute] == 1])
negative_count = value_count - positive_count
p = positive_count / value_count
n = negative_count / value_count
gain = self._information_gain(p, n)
rule[1][value] = (positive_count, negative_count, gain)
total_gain = sum([value[2] for value in rule[1].values()])
if total_gain > best_gain:
best_rule = rule
best_gain = total_gain
if best_gain <= 0:
break
rules.append(best_rule)
attributes.remove(best_rule[0])
self.rule = rules
def _information_gain(self, p, n):
if p == 0 or n == 0:
return 0
return p * self._log2(p / (p + n)) + n * self._log2(n / (p + n))
def _log2(self, x):
if x == 0:
return 0
return math.log2(x)
def predict(self, test):
if self.rule is None:
raise Exception('FOIL model has not been trained yet')
predictions = []
for i, row in test.iterrows():
prediction = 0
for rule in self.rule:
attribute, values = rule
value_count = values.get(row[attribute], (0, 0, 0))[0] + values.get(row[attribute], (0, 0, 0))[1]
if value_count == 0:
continue
positive_count, negative_count, _ = values.get(row[attribute], (0, 0, 0))
p = positive_count / value_count
n = negative_count / value_count
if p >= 0.5:
prediction = 1
else:
prediction = 0
if prediction == 1:
break
predictions.append(prediction)
return predictions
```
这里实现了一个FOIL分类器,它接受一个数据集和目标属性作为输入。`fit`方法用于训练模型,`predict`方法用于进行预测。`fit`方法使用FOIL算法来构建规则列表,`predict`方法使用这些规则来进行预测。
注意:这只是一个简单的实现,只适用于小型数据集。在实际应用中,可能需要更高效的数据结构和算法来处理大型数据集。
阅读全文
相关推荐
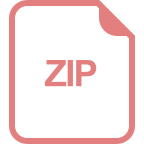
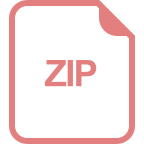

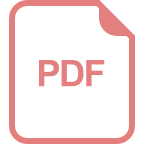
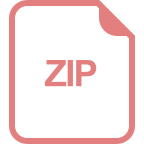
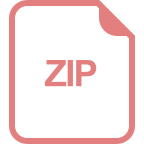
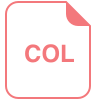
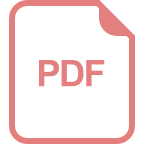
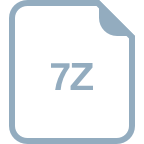
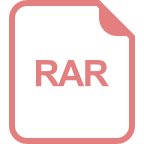
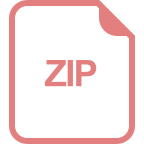
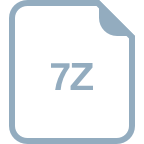
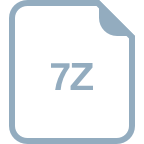
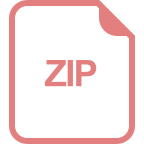
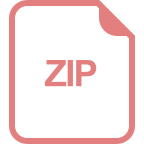
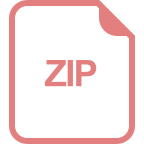