用C++语言直接编写代码不要复述文字,在主函数中,可以创建一个指向 Book 类的指针数组,然后动态地创建 Book 类的对象和 EBook 类的对象,将这些对象存储到指针数组中。然后,可以通过循环来调用每个对象的 print() 函数,实现多态输出。
时间: 2023-02-07 19:39:02 浏览: 65
你可以使用如下的代码来实现这个功能:
```
#include <iostream>
#include <string>
class Book {
public:
Book(std::string title, std::string author)
: m_title(title), m_author(author) {}
virtual void print() const {
std::cout << "Book: " << m_title << ", Author: " << m_author << std::endl;
}
private:
std::string m_title;
std::string m_author;
};
class EBook : public Book {
public:
EBook(std::string title, std::string author, std::string format)
: Book(title, author), m_format(format) {}
virtual void print() const {
std::cout << "EBook: " << m_title << ", Author: " << m_author << ", Format: " << m_format << std::endl;
}
private:
std::string m_format;
};
int main() {
// 创建指向 Book 类的指针数组
Book* books[5];
// 动态地创建 Book 类的对象和 EBook 类的对象
books[0] = new Book("Book 1", "Author 1");
books[1] = new Book("Book 2", "Author 2");
books[2] = new EBook("EBook 3", "Author 3", "PDF");
books[3] = new EBook("EBook 4", "Author 4", "ePub");
books[4] = new Book("Book 5", "Author 5");
// 通过循环来调用每个对象的 print() 函数,实现多态输出
for (int i = 0; i < 5; i++) {
books[i]->print();
}
// 释放内存
for (int i = 0; i < 5; i++) {
delete books[i];
}
return 0;
}
```
在这段代码中,我们使用了虚函数来实现多态,在父类中声明了一个虚函数 `print()`,然后在子类中重写了这个虚函数,最后在主函数中调用虚函数时,会根据对象的实际类型来调用对应的函数。
相关推荐
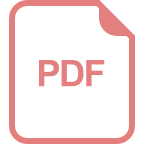
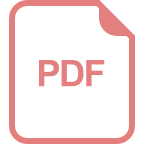
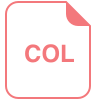
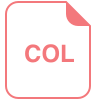
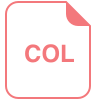
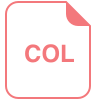




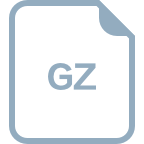
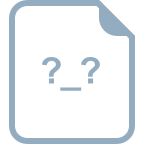