You may notice that entering a non-numeric value and clicking the ‘Check!’ button will cause a run-time error on the console. Therefore, your second task is to improve the application developed in Task 1 to ensure the user is only allowed to supply valid input values, i.e. a 4/5 number between 1 and 10 (inclusive). The application must still function as specified in Task 1 although you may remove the ‘Check!’ button if you wish
时间: 2024-03-18 13:39:21 浏览: 15
Sure, I can help you with that.
To ensure that the user only inputs valid values, you can use input validation in your code. Here's a sample code snippet that demonstrates how you can implement input validation in your code:
```
import java.util.Scanner;
public class YourClassNameHere {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int inputNumber;
while (true) {
System.out.print("Please enter a 4/5 number between 1 and 10 (inclusive): ");
String input = scanner.nextLine();
try {
inputNumber = Integer.parseInt(input);
if (inputNumber == 4 || inputNumber == 5) {
break;
} else {
System.out.println("Invalid input! Please enter a valid 4/5 number between 1 and 10 (inclusive).");
}
} catch (NumberFormatException e) {
System.out.println("Invalid input! Please enter a valid 4/5 number between 1 and 10 (inclusive).");
}
}
// Perform calculations using valid input value inputNumber
}
}
```
In this code, we are using a while loop to prompt the user to enter a valid input until they do so. We are using the `Scanner` class to get user input. We are using a `try-catch` block to catch any `NumberFormatException` that might occur when parsing the user input.
We are also checking if the inputNumber is equal to 4 or 5 and breaking out of the loop if it is. If the inputNumber is not equal to 4 or 5, we are displaying an error message and prompting the user to enter a valid input again.
Once a valid input is entered, the code will proceed with the calculations as specified in Task 1.
相关推荐
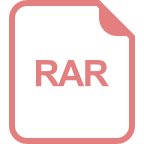
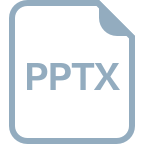














