分析需求o BlinkMorseCode: function to take a capital letter as input and control the P_LD1 to blink the corresponding Morse-code. Debug your code for the letters ‘H’, ‘O’, and ‘X’. o ReadChar: A function that reads the received character from UART and checks if it is a capital letter. Debug your code by entering different characters in the serial terminal and checking the value of X and making sure it is the same. o AddtoCharBuff: A function to add X to CharBuff and updates the tail. Debug by checking the head and tail and values in CharBuff after adding X when CharBuff is full, almost full, half full, empty. o ReadfromCharBuff: A function to read and return the oldest value in CharBuff. Debug by checking that the correct character is returned and tail and head have the correct values after reading from a full, almost full, half full, or empty CharBuff.
时间: 2024-04-26 20:20:53 浏览: 8
1. BlinkMorseCode:这个函数需要接受一个大写字母作为输入,并控制 P_LD1 以闪烁对应的莫尔斯码。我们需要对字母 ‘H’,‘O’和‘X’ 进行调试,确保它们能正确地显示对应的莫尔斯码。
2. ReadChar:这个函数需要从 UART 中读取接收到的字符,并检查它是否为大写字母。我们需要通过在串行终端中输入不同的字符并检查 X 的值,确保它与实际值相同。
3. AddtoCharBuff:这个函数需要向 CharBuff 中添加 X,并更新尾部。我们需要在 CharBuff 满、几乎满、一半满和空的情况下检查头、尾和 CharBuff 中的值,以调试这个函数。
4. ReadfromCharBuff:这个函数需要读取并返回 CharBuff 中最旧的值。我们需要在 CharBuff 满、几乎满、一半满和空的情况下检查返回的字符是否正确,并检查读取后头和尾的值是否正确。
相关问题
You are required to write a C program to: • Initialize GPIO peripherals • Initialise UART peripheral for receiving ASCII characters ‘A’ to ‘Z’ at baud 9600 • Initialise an internal array to hold 10 characters with head and tail: CharBuff • Repeat the following:o When data is received on the serial communication port, read ASCII character X, o If received character X is a capital letter add it to CharBuff, else ignore. o While CharBuff is not empty, transmit the morse code of the oldest stored character by blinking the LED (code provided for you). o When CharBuff is full, disable UART RX. o If UART RX is disabled, pushing the button P_B1 will activate it; otherwise, pushing the button does not affect your programme. You are recommended to use interrupt to control UART receiving data and coordinate the operation between CharBuff and P_LD2. 其中,启用uart代码位uart_enable();
以下是一个简单的示例程序,演示了如何实现上述要求:
```c
#include <stdio.h>
#include <stdlib.h>
#include "stm32f4xx.h"
#define BUF_SIZE 10
// 初始化 GPIO
void init_gpio() {
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_5; // LD2
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0; // P_B1
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
}
// 初始化 UART
void init_uart() {
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART2, ENABLE);
USART_InitTypeDef USART_InitStructure;
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx;
USART_Init(USART2, &USART_InitStructure);
NVIC_InitTypeDef NVIC_InitStructure;
NVIC_InitStructure.NVIC_IRQChannel = USART2_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
USART_ITConfig(USART2, USART_IT_RXNE, ENABLE);
USART_Cmd(USART2, ENABLE);
}
// 定义 morse 码表
const char* morse_table[] = {
".-", "-...", "-.-.", "-..", ".", "..-.", "--.", "....", "..", ".---", "-.-",
".-..", "--", "-.", "---", ".--.", "--.-", ".-.", "...", "-", "..-", "...-",
".--", "-..-", "-.--", "--.."
};
// 定义字符缓冲区
char char_buff[BUF_SIZE];
int head = 0;
int tail = 0;
int count = 0;
int uart_enabled = 1;
// 将一个大写字母转换为 morse 码
void blink_morse(char c) {
if (c >= 'A' && c <= 'Z') {
const char* morse = morse_table[c - 'A'];
for (int i = 0; morse[i] != '\0'; i++) {
if (morse[i] == '.') {
GPIO_SetBits(GPIOA, GPIO_Pin_5); // LD2 on
delay(100);
GPIO_ResetBits(GPIOA, GPIO_Pin_5); // LD2 off
delay(100);
} else if (morse[i] == '-') {
GPIO_SetBits(GPIOA, GPIO_Pin_5); // LD2 on
delay(300);
GPIO_ResetBits(GPIOA, GPIO_Pin_5); // LD2 off
delay(100);
}
}
delay(200);
}
}
// 中断处理函数,当有数据接收时调用
void USART2_IRQHandler(void) {
if (USART_GetITStatus(USART2, USART_IT_RXNE) != RESET) {
char c = USART_ReceiveData(USART2);
if (c >= 'A' && c <= 'Z') {
if (count < BUF_SIZE) {
char_buff[tail] = c;
tail = (tail + 1) % BUF_SIZE;
count++;
if (count == BUF_SIZE) {
uart_enabled = 0;
USART_ITConfig(USART2, USART_IT_RXNE, DISABLE);
}
}
}
}
}
int main(void) {
init_gpio();
init_uart();
while (1) {
if (!uart_enabled && GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_0) == RESET) {
// 如果 UART RX 被禁用并且 P_B1 被按下,则重新启用 UART RX
head = 0;
tail = 0;
count = 0;
uart_enabled = 1;
USART_ITConfig(USART2, USART_IT_RXNE, ENABLE);
}
if (count > 0) {
blink_morse(char_buff[head]);
head = (head + 1) % BUF_SIZE;
count--;
}
}
return 0;
}
```
这段代码使用 STM32F4 系列芯片作为开发板,通过 GPIO 控制板载 LED(LD2)的闪烁来输出 Morse 电码,通过 UART 串口接收来自外部设备的 ASCII 字符,将收到的大写字母存储在内部缓冲区(CharBuff)中,并在收到每个字符后立即将其转换为 Morse 电码输出。如果缓冲区已满,则禁用 UART 接收,并在按下按钮后重新启用它。
这是什么问题main.c(40): error C267: 'led_blink_off': requires ANSI-style prototype
这个错误是因为函数 `led_blink_off` 没有在之前声明时指定参数类型,在使用该函数时编译器无法确定参数类型。要解决这个问题,可以在函数 `led_blink_off` 的前面加上函数声明,指定参数类型,例如:
```c
void led_blink_off(int led_pin);
```
如果该函数有多个参数,则应该按顺序列出参数类型,例如:
```c
void led_blink_off(int led_pin, int blink_times);
```
在声明函数时,也可以给参数命名,这样更容易阅读和理解代码:
```c
void led_blink_off(int led_pin, int blink_times);
```
无论哪种方式,都可以帮助编译器正确地解析函数调用,并避免编译错误。
相关推荐
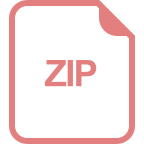
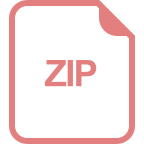












