static void blink_led(void) { /* If the addressable LED is enabled */ if (s_led_state) { /* Set the LED pixel using RGB from 0 (0%) to 255 (100%) for each color */ led_strip_set_pixel(led_strip, 0, 16, 16, 16); /* Refresh the strip to send data */ led_strip_refresh(led_strip); } else { /* Set all LED off to clear all pixels */ led_strip_clear(led_strip); } } static void configure_led(void) { ESP_LOGI(TAG, "Example configured to blink addressable LED!"); /* LED strip initialization with the GPIO and pixels number*/ led_strip_config_t strip_config = { .strip_gpio_num = BLINK_GPIO, .max_leds = 1, // at least one LED on board }; led_strip_rmt_config_t rmt_config = { .resolution_hz = 10 * 1000 * 1000, // 10MHz }; ESP_ERROR_CHECK(led_strip_new_rmt_device(&strip_config, &rmt_config, &led_strip)); /* Set all LED off to clear all pixels */ led_strip_clear(led_strip); } #elif CONFIG_BLINK_LED_GPIO static void blink_led(void) { /* Set the GPIO level according to the state (LOW or HIGH)*/ gpio_set_level(BLINK_GPIO, s_led_state); } static void configure_led(void) { ESP_LOGI(TAG, "Example configured to blink GPIO LED!"); gpio_reset_pin(BLINK_GPIO); /* Set the GPIO as a push/pull output */ gpio_set_direction(BLINK_GPIO, GPIO_MODE_OUTPUT); } #endif void app_main(void) { /* Configure the peripheral according to the LED type */ configure_led(); while (1) { ESP_LOGI(TAG, "Turning the LED %s!", s_led_state == true ? "ON" : "OFF"); blink_led(); /* Toggle the LED state */ s_led_state = !s_led_state; vTaskDelay(CONFIG_BLINK_PERIOD / portTICK_PERIOD_MS); } }
时间: 2024-01-09 15:03:56 浏览: 280
这段代码是一个 ESP32 的示例程序,用于控制 LED 灯进行闪烁。该程序通过宏定义来选择控制 LED 的方式,可以选择使用地址可寻址 LED 或者 GPIO 控制 LED。在主函数中,首先调用 configure_led() 函数来初始化 LED,并在一个无限循环中调用 blink_led() 函数来控制 LED 闪烁,并且通过 vTaskDelay() 函数来控制 LED 闪烁的频率。其中,s_led_state 变量用于记录 LED 的状态,当它为 true 时,LED 会亮起;当它为 false 时,LED 会熄灭。如果选择的是地址可寻址 LED 控制方式,则会调用 led_strip_set_pixel() 函数来设置 LED 的颜色,并通过 led_strip_refresh() 函数来将设置的颜色数据发送到 LED 上;如果选择的是 GPIO 控制方式,则会调用 gpio_set_level() 函数来设置 GPIO 引脚的电平来控制 LED 的亮灭。
相关问题
分析代码功能static void blink_led(void) { /* Set the GPIO level according to the state (LOW or HIGH)*/ gpio_set_level(BLINK_GPIO, s_led_state); } static void configure_led(void) { ESP_LOGI(TAG, "Example configured to blink GPIO LED!"); gpio_reset_pin(BLINK_GPIO); /* Set the GPIO as a push/pull output */ gpio_set_direction(BLINK_GPIO, GPIO_MODE_OUTPUT); } #endif void app_main(void) { /* Configure the peripheral according to the LED type */ configure_led(); while (1) { ESP_LOGI(TAG, "Turning the LED %s!", s_led_state == true ? "ON" : "OFF"); blink_led(); /* Toggle the LED state */ s_led_state = !s_led_state; vTaskDelay(CONFIG_BLINK_PERIOD / portTICK_PERIOD_MS); } }
这是一个 ESP32 芯片的示例代码,主要实现以下功能:
1. 定义了一个静态函数 blink_led(),用于控制 GPIO 引脚的电平状态,从而控制 LED 灯的亮灭。
2. 定义了一个静态函数 configure_led(),用于配置 GPIO 引脚的方向和模式,将其设置为输出模式,以便控制 LED 灯的亮灭。
3. 在 app_main() 函数中,首先调用 configure_led() 函数,对 GPIO 引脚进行配置。
4. 进入一个无限循环,不断地控制 LED 灯的亮灭状态。具体过程如下:
- 打印日志,指示当前 LED 灯的状态(ON 或 OFF)。
- 调用 blink_led() 函数,控制 GPIO 引脚的电平状态,从而控制 LED 灯的亮灭。
- 切换 LED 灯的状态(ON 或 OFF)。
- 延时一定时间,以控制 LED 灯的闪烁频率。
这段代码的作用是让 ESP32 上的 LED 灯不断地闪烁,以检验 GPIO 引脚的配置和控制是否正确。
while (1) { ESP_LOGI(TAG, "Turning the LED %s!", s_led_state == true ? "ON" : "OFF"); blink_led(); /* Toggle the LED state */ s_led_state = !s_led_state; vTaskDelay(CONFIG_BLINK_PERIOD / portTICK_PERIOD_MS); }
这段代码是一个无限循环的函数,其中包含了三个步骤:
1. 打印当前 LED 状态(开启或关闭)。
2. 闪烁 LED。
3. 切换 LED 状态,即从开启变为关闭,或从关闭变为开启。
在每次循环结束后,使用 vTaskDelay 函数暂停一段时间,以控制 LED 闪烁的频率。
这段代码可能是用于控制某个设备上的 LED 灯,让它以一定的频率闪烁,以提醒用户设备正在工作。
阅读全文
相关推荐
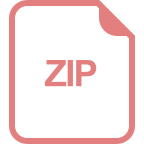








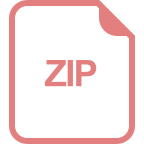
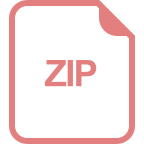
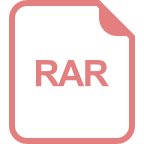
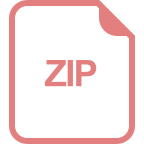
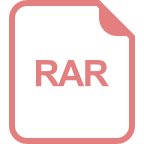
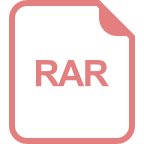
