python使用mysql
时间: 2023-05-27 09:03:10 浏览: 88
Python可以使用MySQL数据库进行数据存储和查询。下面是Python操作MySQL的简单示例:
1. 首先需要安装MySQL官方提供的驱动程序pymysql。
```
pip install pymysql
```
2. 连接MySQL数据库:
```python
import pymysql
# 连接MySQL数据库
db = pymysql.connect(host='localhost', user='root', password='root', port=3306, db='test')
cursor = db.cursor()
```
3. 创建表:
```python
# 创建表
sql = '''
create table if not exists students (
id int not null auto_increment,
name varchar(20) not null,
age int not null,
primary key (id)
);
'''
cursor.execute(sql)
```
4. 插入数据:
```python
# 插入数据
sql = "insert into students(name, age) values(%s, %s)"
cursor.execute(sql, ('Tom', 18))
cursor.execute(sql, ('Jerry', 20))
db.commit()
```
5. 查询数据:
```python
# 查询数据
sql = "select * from students where age >= %s"
cursor.execute(sql, 18)
results = cursor.fetchall()
for row in results:
print(row)
```
6. 关闭连接:
```python
# 关闭连接
cursor.close()
db.close()
```
阅读全文
相关推荐
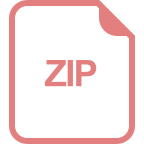















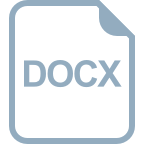