请用C语言写出链表储存定义
时间: 2024-01-03 10:04:35 浏览: 22
Sure, here's an example C code for defining and storing data in a singly linked list:
```
// Define the structure for the linked list node
typedef struct Node {
int data; // data to be stored in the node
struct Node* next; // pointer to the next node in the list
} Node;
// Create a new node with given data and return a pointer to it
Node* createNode(int data) {
Node* newNode = (Node*)malloc(sizeof(Node)); // allocate memory dynamically for the new node
newNode->data = data; // set the data value
newNode->next = NULL; // set the next node pointer to NULL
return newNode;
}
// Example usage: create a linked list with 3 nodes storing values 1, 2, 3
int main() {
Node* head = createNode(1); // create the head node with value 1
head->next = createNode(2); // add a second node with value 2
head->next->next = createNode(3); // add a third node with value 3
return 0;
}
```
This is just a simple example, and there are many variations on how linked lists can be defined and used in C programs depending on requirements.
相关推荐
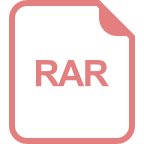
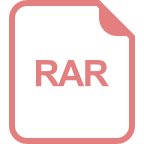
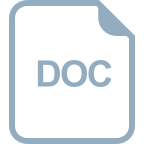














