怎么利用pyopencl来加快torch训练模型的速度
时间: 2024-05-10 10:21:01 浏览: 98
PyOpenCL 是一个用来访问 OpenCL 的 Python 模块,可以用来加速 Torch 训练模型的速度。以下是一些利用 PyOpenCL 加速 Torch 训练模型的方法:
1. 使用 PyTorch 的 PyOpenCL 后端
PyTorch 提供了一个名为 PyOpenCL 的后端,可以将模型转换为 OpenCL 核心,从而加速模型训练。要使用 PyOpenCL 后端,只需要在 PyTorch 中导入 PyOpenCL 并将模型放到 PyOpenCL 设备上即可。
```python
import torch
import pyopencl as cl
# Use PyOpenCL backend
torch.backends.opencl.is_available()
torch.backends.opencl.initialize()
# Create a PyTorch tensor on a PyOpenCL device
device = torch.device("opencl:0")
x = torch.randn(10, 10, device=device)
```
2. 使用 PyOpenCL 的并行计算能力
PyOpenCL 提供了一个名为 Context 的类,可以用来管理 OpenCL 设备和上下文。通过使用 Context,可以实现并行计算,从而加速模型训练。
```python
import pyopencl as cl
# Create a PyOpenCL context and queue
platform = cl.get_platforms()[0]
device = platform.get_devices()[0]
context = cl.Context([device])
queue = cl.CommandQueue(context)
# Create PyOpenCL buffers for input and output data
input_data = cl.Buffer(context, cl.mem_flags.READ_ONLY, size=input_size)
output_data = cl.Buffer(context, cl.mem_flags.WRITE_ONLY, size=output_size)
# Enqueue a PyOpenCL kernel
kernel = """
__kernel void my_kernel(__global float* input, __global float* output) {
int i = get_global_id(0);
output[i] = input[i] * 2;
}
"""
program = cl.Program(context, kernel).build()
program.my_kernel(queue, (num_elements,), None, input_data, output_data)
# Read the output data from the PyOpenCL buffer
output = np.empty(num_elements, dtype=np.float32)
cl.enqueue_copy(queue, output, output_data)
```
3. 使用 PyOpenCL 的内存管理功能
PyOpenCL 提供了一个名为 MemoryPool 的类,可以用来管理内存分配,从而减少内存分配和释放的开销。通过使用 MemoryPool,可以进一步加快模型训练的速度。
```python
import pyopencl as cl
import pyopencl.tools as cltools
# Create a PyOpenCL memory pool
mem_pool = cltools.MemoryPool()
# Create a PyOpenCL buffer using the memory pool
input_data = cl.Buffer(context, cl.mem_flags.READ_ONLY, size=input_size, allocator=mem_pool.allocate)
# Enqueue a PyOpenCL kernel using the memory pool
program.my_kernel(queue, (num_elements,), None, input_data, output_data, allocator=mem_pool.allocate)
# Release the PyOpenCL memory pool
mem_pool.release()
```
通过以上三种方法,可以利用 PyOpenCL 加速 Torch 训练模型的速度。需要注意的是,PyOpenCL 的使用需要一定的 OpenCL 知识,需要对 OpenCL 的编程模型和内存管理有一定的了解。同时,PyOpenCL 的性能也会受到硬件设备的限制,需要根据实际情况进行优化。
阅读全文
相关推荐
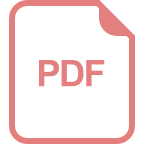
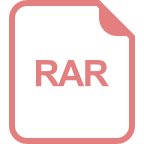
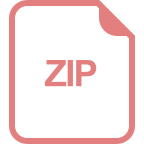
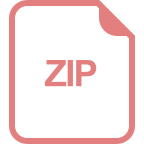
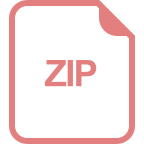
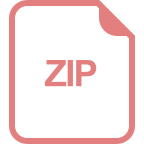
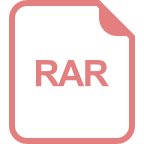
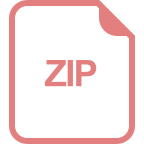
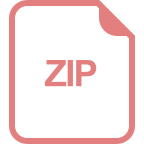
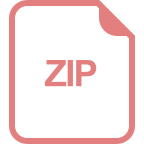
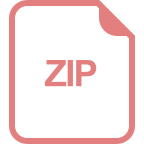
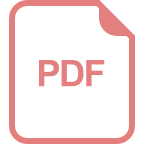
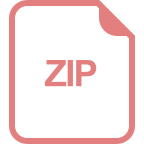
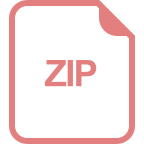
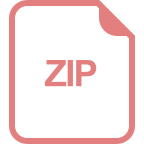
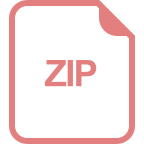
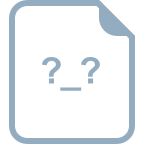