python如何检查controller 模块安装和路径设置是否正确
时间: 2023-05-20 18:05:13 浏览: 154
您可以使用以下命令检查Python中是否安装了controller模块:
```
pip freeze | grep controller
```
如果返回了controller模块的版本号,则表示已安装。如果没有返回任何内容,则表示未安装。
要检查路径设置是否正确,您可以使用以下代码:
```python
import sys
print(sys.path)
```
这将打印出Python解释器搜索模块的路径列表。确保您的controller模块所在的路径在其中。
相关问题
webots python nao机器人 A*算法 路径规划
在Webots中使用Python编写A*算法的路径规划可以通过以下步骤实现:
1. 在Webots中创建一个场景,将NAO机器人放置在其中。
2. 安装Python控制器插件,以便使用Python编写控制器程序。
3. 使用Python编写A*算法的路径规划代码,可以使用Python中的heapq模块实现最小堆数据结构,同时使用Webots提供的API实现机器人的运动控制。
4. 将编写好的Python路径规划代码与NAO机器人控制器程序结合使用,实现机器人的自主导航功能。
以下是一个简单的示例代码,演示如何在Webots中使用Python编写A*算法的路径规划:
```python
import heapq
from controller import Robot
robot = Robot()
# 定义机器人的运动控制函数
def move(x, y):
# 将机器人移动到指定位置
# ...
# 定义A*算法的路径规划函数
def A_star(start, end):
# 初始化路径规划所需的数据结构
# ...
# 将起点加入open列表
heapq.heappush(open, (0, start))
# 开始路径规划
while open:
# 从open列表中取出f值最小的节点
# ...
# 如果当前节点是终点,则返回路径
if current_node == end:
# 构造路径
# ...
# 将当前节点标记为已访问
# ...
# 遍历当前节点的所有邻居节点
# ...
# 对于未访问过的邻居节点,更新其f值并加入open列表
# ...
# 如果open列表为空,则无法到达终点,返回空路径
return []
# 定义起点和终点
start = (0, 0)
end = (10, 10)
# 使用A*算法进行路径规划
path = A_star(start, end)
# 依次移动机器人到路径上的每个节点
for node in path:
move(node[0], node[1])
```
需要注意的是,以上示例代码只是一个简单的演示,并没有完整实现机器人的自主导航功能。在实际应用中,还需要考虑机器人与障碍物的碰撞检测、路径跟踪精度等问题。
写一个文件导入的controller ,导入到指定的路径下
好的,这是一个比较简单的问题,以下是一个示例代码:
```python
import os
class FileImportController:
def __init__(self, file_path, destination_path):
self.file_path = file_path
self.destination_path = destination_path
def import_file(self):
try:
file_name = os.path.basename(self.file_path)
destination_file_path = os.path.join(self.destination_path, file_name)
with open(self.file_path, 'rb') as f:
with open(destination_file_path, 'wb') as destination_file:
destination_file.write(f.read())
print(f"File {file_name} imported successfully to {destination_file_path}")
except Exception as e:
print(f"Error importing file: {e}")
```
这是一个简单的文件导入控制器,它接受两个参数:文件路径和目标路径。它使用 Python 的内置 os 模块来获取文件名和目标文件路径,并使用 with 语句打开文件并将其复制到目标路径。如果导入成功,它将打印一条成功消息,否则将打印错误消息。
阅读全文
相关推荐




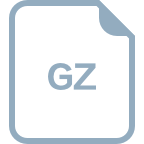










