Java写一个蓝牙工具类代码,获取蓝牙设备的数据
时间: 2024-02-09 22:10:59 浏览: 29
以下是一个基本的Java蓝牙工具类,可以用于获取蓝牙设备的数据:
```java
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.UUID;
import javax.bluetooth.BluetoothDevice;
import javax.bluetooth.BluetoothSocket;
public class BluetoothUtil {
private BluetoothDevice device;
private BluetoothSocket socket;
private InputStream inputStream;
private OutputStream outputStream;
public BluetoothUtil(BluetoothDevice device) {
this.device = device;
}
public boolean connect() {
try {
socket = device.createRfcommSocketToServiceRecord(UUID.fromString("00001101-0000-1000-8000-00805F9B34FB"));
socket.connect();
inputStream = socket.getInputStream();
outputStream = socket.getOutputStream();
return true;
} catch (IOException e) {
e.printStackTrace();
return false;
}
}
public void disconnect() {
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public byte[] read() {
byte[] buffer = new byte[1024];
int bytes;
try {
bytes = inputStream.read(buffer);
byte[] data = new byte[bytes];
System.arraycopy(buffer, 0, data, 0, bytes);
return data;
} catch (IOException e) {
e.printStackTrace();
return null;
}
}
public void write(byte[] data) {
try {
outputStream.write(data);
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这个工具类包括了连接蓝牙设备、断开连接、读取数据以及写入数据等基本操作。在使用时,可以先实例化一个BluetoothUtil对象,然后调用connect()方法连接蓝牙设备,调用read()方法读取数据,调用write()方法写入数据,最后调用disconnect()方法断开连接。需要注意的是,这个工具类只是一个基本的示例,具体的实现可能需要根据具体的需求进行修改。
相关推荐
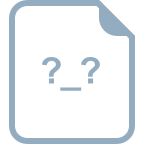














