java安卓开发写一个蓝牙工具类代码,获取蓝牙设备的数据
时间: 2024-02-09 22:11:00 浏览: 214
以下是一个基本的Java安卓蓝牙工具类,可以用于获取蓝牙设备的数据:
```java
import android.bluetooth.BluetoothAdapter;
import android.bluetooth.BluetoothDevice;
import android.bluetooth.BluetoothSocket;
import android.os.Handler;
import android.os.Looper;
import android.util.Log;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.UUID;
public class BluetoothUtil {
private static final String TAG = "BluetoothUtil";
private static final UUID MY_UUID = UUID.fromString("00001101-0000-1000-8000-00805F9B34FB");
private static final int BUFFER_SIZE = 1024;
private BluetoothAdapter bluetoothAdapter;
private BluetoothDevice device;
private BluetoothSocket socket;
private InputStream inputStream;
private OutputStream outputStream;
private byte[] buffer;
private Handler handler;
private OnDataReceivedListener onDataReceivedListener;
public BluetoothUtil(BluetoothAdapter bluetoothAdapter, BluetoothDevice device) {
this.bluetoothAdapter = bluetoothAdapter;
this.device = device;
this.handler = new Handler(Looper.getMainLooper());
this.buffer = new byte[BUFFER_SIZE];
}
public void setOnDataReceivedListener(OnDataReceivedListener onDataReceivedListener) {
this.onDataReceivedListener = onDataReceivedListener;
}
public boolean connect() {
try {
socket = device.createRfcommSocketToServiceRecord(MY_UUID);
socket.connect();
inputStream = socket.getInputStream();
outputStream = socket.getOutputStream();
startReading();
return true;
} catch (IOException e) {
Log.e(TAG, "connect error: " + e.getMessage());
return false;
}
}
public void disconnect() {
try {
socket.close();
} catch (IOException e) {
Log.e(TAG, "disconnect error: " + e.getMessage());
}
}
public void write(byte[] data) {
try {
outputStream.write(data);
} catch (IOException e) {
Log.e(TAG, "write error: " + e.getMessage());
}
}
private void startReading() {
new Thread(new Runnable() {
@Override
public void run() {
while (true) {
try {
int bytes = inputStream.read(buffer);
final byte[] data = new byte[bytes];
System.arraycopy(buffer, 0, data, 0, bytes);
handler.post(new Runnable() {
@Override
public void run() {
if (onDataReceivedListener != null) {
onDataReceivedListener.onDataReceived(data);
}
}
});
} catch (IOException e) {
Log.e(TAG, "read error: " + e.getMessage());
break;
}
}
}
}).start();
}
public interface OnDataReceivedListener {
void onDataReceived(byte[] data);
}
}
```
这个工具类包括了连接蓝牙设备、断开连接、读取数据以及写入数据等基本操作。在使用时,可以先实例化一个BluetoothUtil对象,然后调用connect()方法连接蓝牙设备,调用write()方法写入数据,实现OnDataReceivedListener接口并设置监听器,即可在onDataReceived()方法中获取蓝牙设备返回的数据,最后调用disconnect()方法断开连接。
需要注意的是,这个工具类只是一个基本的示例,具体的实现可能需要根据具体的需求进行修改。另外,由于读取数据是在一个新线程中进行的,需要通过Handler将数据切换到主线程中进行处理。
阅读全文
相关推荐
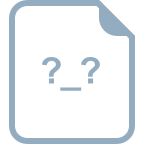
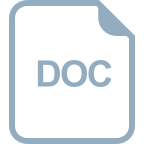
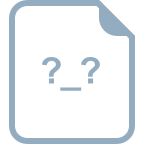
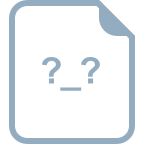
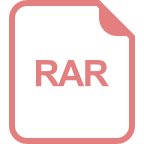
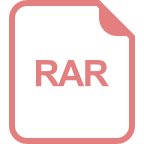
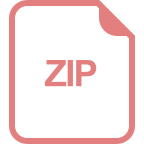
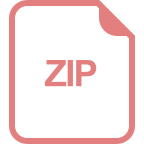
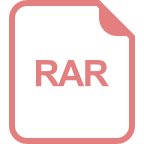
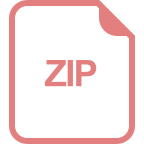
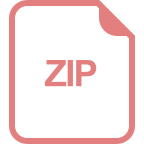
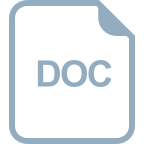
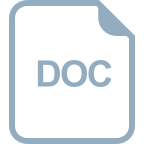
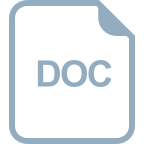
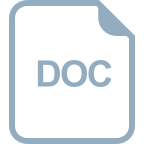