jpa JpaRepository <S extends T> List<S> findAll(Example<S> var1); 条件查询同时分页查询具体怎么写?写个例子
时间: 2023-06-03 16:06:49 浏览: 119
您好!关于您的问题,您可以使用Spring Data JPA提供的Specification来实现条件查询,同时使用Pageable来实现分页查询,具体的实现可以参考以下代码示例:
```java
import org.springframework.data.domain.Example;
import org.springframework.data.domain.ExampleMatcher;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.PageRequest;
import org.springframework.data.jpa.domain.Specification;
import org.springframework.stereotype.Service;
import javax.persistence.criteria.CriteriaBuilder;
import javax.persistence.criteria.CriteriaQuery;
import javax.persistence.criteria.Predicate;
import javax.persistence.criteria.Root;
import java.util.ArrayList;
import java.util.List;
@Service
public interface YourRepository extends JpaRepository<S, Long> {
default Page<S> search(S example, int pageNum, int pageSize) {
ExampleMatcher matcher = ExampleMatcher.matching()
.withMatcher("field1", ExampleMatcher.GenericPropertyMatchers.contains())
.withMatcher("field2", ExampleMatcher.GenericPropertyMatchers.contains())
.withIgnorePaths("id", "field3"); // ignore id and field3
Example<S> exampleFilter = Example.of(example, matcher);
Specification<S> spec = new Specification<S>() {
@Override
public Predicate toPredicate(Root<S> root, CriteriaQuery<?> query, CriteriaBuilder cb) {
List<Predicate> predicates = new ArrayList<>();
/* add your filters here */
predicates.add(cb.equal(root.get("field4"), "value1"));
predicates.add(cb.like(root.get("field5"), "value2%"));
return cb.and(predicates.toArray(new Predicate[predicates.size()]));
}
};
PageRequest pageRequest = PageRequest.of(pageNum, pageSize);
return findAll(spec.and(exampleFilter), pageRequest);
}
}
```
其中,`S`代表实体类型,`field1`到`field5`代表实体中的属性名,`value1`和`value2`代表你要匹配的值,`pageNum`和`pageSize`分别代表页码和每页显示的记录数。
希望这可以帮到您!如果您还有任何问题,请随时联系我。
阅读全文
相关推荐







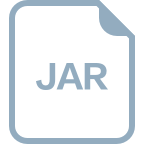







