qt tableview 添加复选框
时间: 2023-07-09 08:02:08 浏览: 194
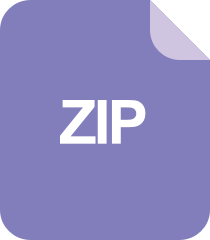
Qt之QTableView添加复选框

### 回答1:
在Qt中使用QTableView添加复选框可以通过自定义委托实现。首先,我们需要创建一个自定义的委托类来设置单元格的编辑控件为复选框。
```cpp
class CheckBoxDelegate : public QItemDelegate
{
public:
void paint(QPainter *painter, const QStyleOptionViewItem &option, const QModelIndex &index) const override
{
QStyleOptionButton checkboxStyle;
checkboxStyle.state |= QStyle::State_Enabled | QStyle::State_Active;
if (index.data(Qt::CheckStateRole).toBool())
checkboxStyle.state |= QStyle::State_On;
else
checkboxStyle.state |= QStyle::State_Off;
checkboxStyle.rect = option.rect.adjusted(0, 0, -1, -1);
QApplication::style()->drawControl(QStyle::CE_CheckBox, &checkboxStyle, painter);
}
QWidget *createEditor(QWidget *parent, const QStyleOptionViewItem &option, const QModelIndex &index) const override
{
QCheckBox *editor = new QCheckBox(parent);
editor->setAttribute(Qt::WA_TransparentForMouseEvents);
editor->setChecked(index.data(Qt::CheckStateRole).toBool());
connect(editor, SIGNAL(clicked(bool)), this, SLOT(commitAndCloseEditor()));
return editor;
}
void setEditorData(QWidget *editor, const QModelIndex &index) const override
{
QCheckBox *checkBox = static_cast<QCheckBox*>(editor);
checkBox->setChecked(index.data(Qt::CheckStateRole).toBool());
}
void setModelData(QWidget *editor, QAbstractItemModel *model, const QModelIndex &index) const override
{
QCheckBox *checkBox = static_cast<QCheckBox*>(editor);
model->setData(index, checkBox->isChecked(), Qt::CheckStateRole);
}
void updateEditorGeometry(QWidget *editor, const QStyleOptionViewItem &option, const QModelIndex &index) const override
{
editor->setGeometry(option.rect);
}
private slots:
void commitAndCloseEditor()
{
QCheckBox *editor = static_cast<QCheckBox*>(sender());
emit commitData(editor);
emit closeEditor(editor);
}
};
```
接下来,在创建QTableView并设置model之后,我们可以为需要添加复选框的列设置自定义委托。
```cpp
QTableView tableView;
QStandardItemModel model(10, 3); // 例如,创建一个10行3列的模型
tableView.setModel(&model);
CheckBoxDelegate delegate;
tableView.setItemDelegateForColumn(2, &delegate); // 为第3列设置自定义委托
```
通过这样的方式,我们可以在QTableView中的指定列上显示复选框,并实现相应的操作。
### 回答2:
Qt的TableView通过使用QCheckBox作为模型元素来添加复选框。可以通过实现自定义的委托类来启用这个功能。
首先,需要创建一个新的委托类,继承QItemDelegate。在这个委托类中,重写createEditor()和setEditorData()方法,并使用QCheckBox作为编辑器。
以下是一个示例:
```cpp
#include <QtWidgets>
class CheckBoxDelegate : public QItemDelegate
{
public:
CheckBoxDelegate(QObject *parent = nullptr)
: QItemDelegate(parent)
{
}
QWidget *createEditor(QWidget *parent, const QStyleOptionViewItem &option, const QModelIndex &index) const override
{
Q_UNUSED(option)
Q_UNUSED(index)
QCheckBox *editor = new QCheckBox(parent);
return editor;
}
void setEditorData(QWidget *editor, const QModelIndex &index) const override
{
QCheckBox *checkBox = qobject_cast<QCheckBox*>(editor);
if (checkBox) {
bool checked = index.model()->data(index, Qt::DisplayRole).toBool();
checkBox->setChecked(checked);
}
}
void setModelData(QWidget *editor, QAbstractItemModel *model, const QModelIndex &index) const override
{
QCheckBox *checkBox = qobject_cast<QCheckBox*>(editor);
if (checkBox) {
model->setData(index, checkBox->isChecked(), Qt::EditRole);
}
}
};
```
接下来,在TableView中使用这个自定义委托类。例如:
```cpp
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QStandardItemModel model(4, 2);
QTableView tableView;
CheckBoxDelegate checkBoxDelegate;
tableView.setItemDelegateForColumn(0, &checkBoxDelegate);
tableView.setModel(&model);
tableView.show();
return app.exec();
}
```
这样,TableView的第一列将显示复选框。您可以通过在模型中设置数据来更改复选框的状态,例如:
```cpp
QStandardItem *item = new QStandardItem();
item->setCheckable(true);
item->setData(Qt::Unchecked, Qt::CheckStateRole);
model->setItem(row, 0, item);
```
这样,当您运行应用程序时,您应该能够在TableView中看到具有复选框的行。
阅读全文
相关推荐

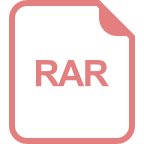

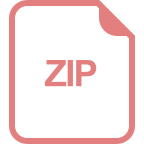
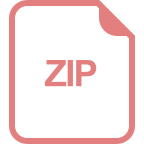






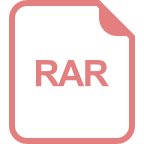
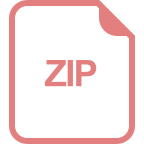
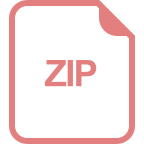

