写一个单向链表存放员工数据,可以进行增删改查,给出完整代码
时间: 2024-03-10 20:45:41 浏览: 60
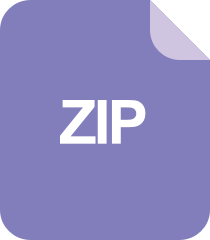
有关单向链表的增删改查
好的,以下是一个简单的单向链表存放员工数据的完整代码示例,包含增加、删除、修改、查询等操作:
```c++
#include <iostream>
using namespace std;
// 定义员工结构体
struct Employee {
int id;
string name;
int age;
string department;
Employee* next;
};
// 定义链表类
class EmployeeList {
public:
EmployeeList(); // 构造函数
~EmployeeList(); // 析构函数
void addEmployee(int id, string name, int age, string department); // 添加员工
void removeEmployee(int id); // 删除员工
void updateEmployee(int id, string name, int age, string department); // 修改员工信息
void findEmployee(int id); // 查找员工信息
void printEmployeeList(); // 打印员工信息列表
private:
Employee* head; // 头节点指针
};
// 构造函数
EmployeeList::EmployeeList() {
head = new Employee;
head->next = NULL;
}
// 析构函数
EmployeeList::~EmployeeList() {
Employee* p = head;
while (p != NULL) {
Employee* q = p;
p = p->next;
delete q;
}
}
// 添加员工
void EmployeeList::addEmployee(int id, string name, int age, string department) {
// 创建新员工结点
Employee* newEmployee = new Employee;
newEmployee->id = id;
newEmployee->name = name;
newEmployee->age = age;
newEmployee->department = department;
newEmployee->next = NULL;
// 找到链表末尾并插入新结点
Employee* p = head;
while (p->next != NULL) {
p = p->next;
}
p->next = newEmployee;
}
// 删除员工
void EmployeeList::removeEmployee(int id) {
// 找到要删除的员工结点并删除
Employee* p = head;
while (p->next != NULL) {
if (p->next->id == id) {
Employee* q = p->next;
p->next = q->next;
delete q;
cout << "员工 " << id << " 已删除" << endl;
return;
}
p = p->next;
}
cout << "找不到员工 " << id << endl;
}
// 修改员工信息
void EmployeeList::updateEmployee(int id, string name, int age, string department) {
// 找到要修改的员工结点并修改信息
Employee* p = head;
while (p->next != NULL) {
if (p->next->id == id) {
p->next->name = name;
p->next->age = age;
p->next->department = department;
cout << "员工 " << id << " 信息已更新" << endl;
return;
}
p = p->next;
}
cout << "找不到员工 " << id << endl;
}
// 查找员工信息
void EmployeeList::findEmployee(int id) {
// 找到要查找的员工结点并输出信息
Employee* p = head;
while (p->next != NULL) {
if (p->next->id == id) {
cout << "员工 " << id << " 的信息:" << endl;
cout << "姓名:" << p->next->name << endl;
cout << "年龄:" << p->next->age << endl;
cout << "部门:" << p->next->department << endl;
return;
}
p = p->next;
}
cout << "找不到员工 " << id << endl;
}
// 打印员工信息列表
void EmployeeList::printEmployeeList() {
cout << "员工信息列表:" << endl;
Employee* p = head->next;
while (p != NULL) {
cout << "ID:" << p->id << ",姓名:" << p->name << ",年龄:" << p->age << ",部门:" << p->department << endl;
p = p->next;
}
}
int main() {
// 创建员工链表
EmployeeList employeeList;
// 添加员工
employeeList.addEmployee(1001, "张三", 30, "销售部");
employeeList.addEmployee(1002, "李四", 25, "研发部");
employeeList.addEmployee(1003, "王五", 28, "财务部");
// 打印员工信息列表
employeeList.printEmployeeList();
// 删除员工
employeeList.removeEmployee(1002);
// 修改员工信息
employeeList.updateEmployee(1003, "赵六", 32, "人事部");
// 查找员工信息
employeeList.findEmployee(1001);
// 打印员工信息列表
employeeList.printEmployeeList();
return 0;
}
```
以上代码仅供参考,实际应用中可能需要根据具体需求进行适当修改。
阅读全文
相关推荐




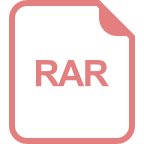
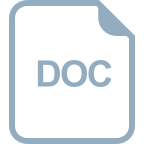
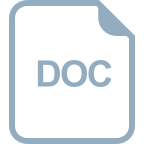
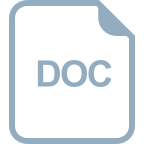
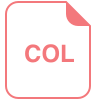
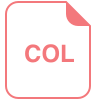
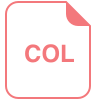
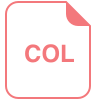
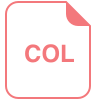
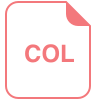