写一个单向链表存放员工name,age,gender,要求增删改查的源码
时间: 2023-04-03 12:00:31 浏览: 60
以下是单向链表存放员工信息的源码,包括增删改查功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义员工结构体
typedef struct Employee {
char name[20];
int age;
char gender[10];
struct Employee *next;
} Employee;
// 创建新员工节点
Employee *createEmployee(char *name, int age, char *gender) {
Employee *newEmployee = (Employee *)malloc(sizeof(Employee));
strcpy(newEmployee->name, name);
newEmployee->age = age;
strcpy(newEmployee->gender, gender);
newEmployee->next = NULL;
return newEmployee;
}
// 在链表末尾添加新员工
void addEmployee(Employee **head, Employee *newEmployee) {
if (*head == NULL) {
*head = newEmployee;
} else {
Employee *current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newEmployee;
}
}
// 根据员工姓名查找员工
Employee *findEmployeeByName(Employee *head, char *name) {
Employee *current = head;
while (current != NULL) {
if (strcmp(current->name, name) == ) {
return current;
}
current = current->next;
}
return NULL;
}
// 根据员工姓名删除员工
void deleteEmployeeByName(Employee **head, char *name) {
Employee *current = *head;
Employee *previous = NULL;
while (current != NULL) {
if (strcmp(current->name, name) == ) {
if (previous == NULL) {
*head = current->next;
} else {
previous->next = current->next;
}
free(current);
return;
}
previous = current;
current = current->next;
}
}
// 根据员工姓名修改员工信息
void updateEmployeeByName(Employee *head, char *name, int age, char *gender) {
Employee *employee = findEmployeeByName(head, name);
if (employee != NULL) {
employee->age = age;
strcpy(employee->gender, gender);
}
}
// 打印所有员工信息
void printAllEmployees(Employee *head) {
Employee *current = head;
while (current != NULL) {
printf("Name: %s, Age: %d, Gender: %s\n", current->name, current->age, current->gender);
current = current->next;
}
}
int main() {
Employee *head = NULL;
addEmployee(&head, createEmployee("Tom", 25, "Male"));
addEmployee(&head, createEmployee("Mary", 30, "Female"));
addEmployee(&head, createEmployee("John", 28, "Male"));
printf("All employees:\n");
printAllEmployees(head);
printf("\n");
printf("Find employee by name:\n");
Employee *employee = findEmployeeByName(head, "Mary");
if (employee != NULL) {
printf("Name: %s, Age: %d, Gender: %s\n", employee->name, employee->age, employee->gender);
} else {
printf("Employee not found.\n");
}
printf("\n");
printf("Delete employee by name:\n");
deleteEmployeeByName(&head, "Mary");
printAllEmployees(head);
printf("\n");
printf("Update employee by name:\n");
updateEmployeeByName(head, "John", 29, "Male");
printAllEmployees(head);
return ;
}
```
以上是单向链表存放员工信息的源码,包括增删改查功能。
相关推荐
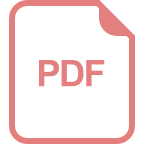













