能帮我用c语言写一个链表的增删改查
时间: 2023-09-22 19:05:43 浏览: 114
### 回答1:
当然可以,链表是 C 语言中常用的数据结构之一,可以使用结构体和指针来实现。以下是一个简单的链表增删改查的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建节点
Node* createNode(int data) {
Node* node = (Node*)malloc(sizeof(Node));
node->data = data;
node->next = NULL;
return node;
}
// 插入节点
void insertNode(Node** head, int data) {
Node* node = createNode(data);
if (*head == NULL) {
*head = node;
} else {
Node* curr = *head;
while (curr->next != NULL) {
curr = curr->next;
}
curr->next = node;
}
}
// 删除节点
void deleteNode(Node** head, int data) {
Node* curr = *head;
Node* prev = NULL;
while (curr != NULL) {
if (curr->data == data) {
if (prev == NULL) {
*head = curr->next;
} else {
prev->next = curr->next;
}
free(curr);
return;
}
prev = curr;
curr = curr->next;
}
}
// 修改节点
void modifyNode(Node* head, int oldData, int newData) {
Node* curr = head;
while (curr != NULL) {
if (curr->data == oldData) {
curr->data = newData;
return;
}
curr = curr->next;
}
}
// 查找节点
Node* findNode(Node* head, int data) {
Node* curr = head;
while (curr != NULL) {
if (curr->data == data) {
return curr;
}
curr = curr->next;
}
return NULL;
}
// 打印链表
void printList(Node* head) {
Node* curr = head;
while (curr != NULL) {
printf("%d ", curr->data);
curr = curr->next;
}
printf("\n");
}
int main() {
Node* head = NULL;
insertNode(&head, 1);
insertNode(&head, 2);
insertNode(&head, 3);
printList(head); // 输出: 1 2 3
deleteNode(&head, 2);
printList(head); // 输出: 1 3
modifyNode(head, 1, 4);
printList(head); // 输出: 4 3
Node* node = findNode(head, 3);
if (node != NULL) {
printf("找到了节点: %d\n", node->data); // 输出: 找到了节点: 3
} else {
printf("未找到节点\n");
}
return ;
}
```
### 回答2:
当然可以帮您用C语言写一个简单的链表的增删改查代码。下面是一个示例:
```C
#include<stdio.h>
#include<stdlib.h>
// 定义链表节点结构
struct Node {
int data;
struct Node* next;
};
// 创建一个空链表
struct Node* createLinkedList()
{
return NULL;
}
// 在链表末尾插入节点
struct Node* insertNode(struct Node* head, int data)
{
// 创建新节点
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = data;
newNode->next = NULL;
if (head == NULL) {
return newNode; // 链表为空,直接返回新节点
}
struct Node* current = head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode; // 将新节点插入到链表末尾
return head;
}
// 在指定位置插入节点
struct Node* insertNodeAt(struct Node* head, int data, int position)
{
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = data;
if (head == NULL || position <= 1) {
newNode->next = head;
return newNode;
}
struct Node* current = head;
int count = 1;
while (count < position - 1 && current->next != NULL) {
current = current->next;
count++;
}
newNode->next = current->next;
current->next = newNode;
return head;
}
// 删除链表中指定数据的节点
struct Node* deleteNode(struct Node* head, int data)
{
if (head == NULL) {
return head;
}
struct Node* current = head;
struct Node* previous = NULL;
while (current != NULL && current->data != data) {
previous = current;
current = current->next;
}
if (current == NULL) {
return head; // 未找到指定数据的节点
}
if (previous == NULL) {
head = head->next; // 删除头节点
} else {
previous->next = current->next; // 删除非头节点
}
free(current); // 释放内存
return head;
}
// 查找链表中指定数据的节点
int searchNode(struct Node* head, int data)
{
struct Node* current = head;
int position = 1;
while (current != NULL && current->data != data) {
current = current->next;
position++;
}
if (current == NULL) {
return -1; // 未找到指定数据的节点
} else {
return position;
}
}
// 打印链表所有节点的数据
void printLinkedList(struct Node* head)
{
struct Node* current = head;
if (current == NULL) {
printf("链表为空。\n");
return;
}
printf("链表的节点数据为: ");
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
int main()
{
struct Node* linkedList = createLinkedList();
linkedList = insertNode(linkedList, 10);
linkedList = insertNode(linkedList, 20);
linkedList = insertNode(linkedList, 30);
linkedList = insertNode(linkedList, 40);
printLinkedList(linkedList);
int searchData = 30;
int position = searchNode(linkedList, searchData);
if (position != -1) {
printf("找到节点%d,位置为%d。\n", searchData, position);
} else {
printf("未找到节点%d。\n", searchData);
}
int deleteData = 20;
linkedList = deleteNode(linkedList, deleteData);
printf("删除节点%d后的链表: ", deleteData);
printLinkedList(linkedList);
int insertData = 50;
int insertPosition = 2;
linkedList = insertNodeAt(linkedList, insertData, insertPosition);
printf("在位置%d插入节点%d后的链表: ", insertPosition, insertData);
printLinkedList(linkedList);
return 0;
}
```
这段代码实现了链表的初始化、增删改查等基本操作。在`main`函数中,首先创建一个空链表,并且按顺序插入了4个节点。然后,通过调用`searchNode`函数查找链表中是否存在节点数据为30的节点,通过调用`deleteNode`函数删除掉节点数据为20的节点,通过调用`insertNodeAt`函数在位置2插入了节点数据为50的节点。
最后运行程序时,会输出所做的操作后的链表结果。
### 回答3:
当然可以!下面是一个基本的链表增删改查的C语言示例:
```C
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建一个新节点
Node* createNode(int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// 在链表末尾插入节点
Node* insertNode(Node* head, int data) {
Node* newNode = createNode(data);
if (head == NULL) {
head = newNode;
} else {
Node* temp = head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
return head;
}
// 在指定位置插入节点
Node* insertNodeAtIndex(Node* head, int data, int position) {
if (head == NULL || position <= 0) {
return insertNode(head, data);
}
Node* newNode = createNode(data);
if (position == 1) {
newNode->next = head;
return newNode;
}
Node* temp = head;
int i;
for (i = 2; i < position; i++) {
if (temp->next == NULL) {
break;
}
temp = temp->next;
}
newNode->next = temp->next;
temp->next = newNode;
return head;
}
// 删除指定位置的节点
Node* deleteNodeAtIndex(Node* head, int position) {
if (head == NULL || position <= 0) {
return head;
}
if (position == 1) {
Node* temp = head->next;
free(head);
return temp;
}
Node* temp = head;
int i;
for (i = 2; i < position; i++) {
if (temp->next == NULL) {
break;
}
temp = temp->next;
}
if (temp->next != NULL) {
Node* deletedNode = temp->next;
temp->next = deletedNode->next;
free(deletedNode);
}
return head;
}
// 在链表中查找指定值的节点位置
int findNodePosition(Node* head, int data) {
int position = 1;
Node* temp = head;
while (temp != NULL) {
if (temp->data == data) {
return position;
}
temp = temp->next;
position++;
}
return -1; // 表示未找到
}
// 打印链表
void printLinkedList(Node* head) {
Node* temp = head;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
int main() {
Node* head = NULL;
// 在链表末尾插入节点
head = insertNode(head, 1);
head = insertNode(head, 2);
head = insertNode(head, 3);
// 打印链表
printf("链表内容:");
printLinkedList(head);
// 在指定位置插入节点
head = insertNodeAtIndex(head, 4, 2);
// 打印链表
printf("链表内容:");
printLinkedList(head);
// 删除指定位置的节点
head = deleteNodeAtIndex(head, 1);
// 打印链表
printf("链表内容:");
printLinkedList(head);
// 在链表中查找节点位置
int position = findNodePosition(head, 4);
if (position != -1) {
printf("节点 4 的位置:%d\n", position);
} else {
printf("节点 4 未找到\n");
}
return 0;
}
```
这个示例提供了链表的基本操作,包括在链表末尾插入节点、在指定位置插入节点、删除指定位置的节点以及在链表中查找指定值的节点位置。你可以根据实际需要进行修改和扩展。
阅读全文
相关推荐
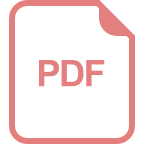




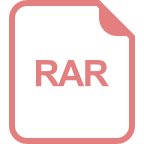
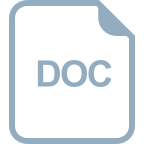
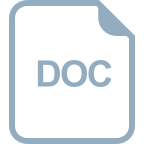
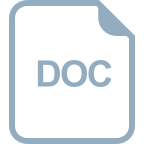






