c语言的链表程序,增删改查
时间: 2023-08-10 07:43:45 浏览: 124
好的,下面是一个 C 语言链表程序示例,包含了链表的增删改查功能:
```c
#include <stdio.h>
#include <stdlib.h>
/* 定义链表节点结构体 */
typedef struct Node {
int data; // 数据域
struct Node* next; // 指针域,指向下一个节点
} Node;
/* 创建链表 */
Node* createList(int n) {
Node* head = NULL; // 头指针
Node* tail = NULL; // 尾指针
for (int i = 0; i < n; i++) {
Node* newNode = (Node*)malloc(sizeof(Node)); // 创建一个新节点
if (newNode == NULL) {
printf("Error: memory allocation failed!\n");
exit(1);
}
printf("Please input the data of node %d: ", i + 1);
scanf("%d", &newNode->data);
newNode->next = NULL;
if (i == 0) { // 第一个节点
head = newNode;
tail = newNode;
} else { // 后续节点
tail->next = newNode;
tail = newNode;
}
}
return head;
}
/* 打印链表 */
void printList(Node* head) {
printf("List: ");
for (Node* p = head; p != NULL; p = p->next) {
printf("%d ", p->data);
}
printf("\n");
}
/* 向链表中插入节点 */
Node* insertNode(Node* head, int index, int data) {
if (index < 0) {
printf("Error: invalid index!\n");
return head;
}
Node* newNode = (Node*)malloc(sizeof(Node)); // 创建一个新节点
if (newNode == NULL) {
printf("Error: memory allocation failed!\n");
exit(1);
}
newNode->data = data;
newNode->next = NULL;
if (index == 0) { // 在链表头部插入节点
newNode->next = head;
return newNode;
}
Node* p = head;
for (int i = 0; i < index - 1; i++) { // 找到待插入位置的前一个节点
if (p == NULL) {
printf("Error: invalid index!\n");
return head;
}
p = p->next;
}
newNode->next = p->next;
p->next = newNode;
return head;
}
/* 删除链表中的节点 */
Node* deleteNode(Node* head, int index) {
if (index < 0) {
printf("Error: invalid index!\n");
return head;
}
if (index == 0) { // 删除链表头部节点
Node* p = head;
head = head->next;
free(p);
return head;
}
Node* p = head;
for (int i = 0; i < index - 1; i++) { // 找到待删除节点的前一个节点
if (p == NULL || p->next == NULL) {
printf("Error: invalid index!\n");
return head;
}
p = p->next;
}
Node* q = p->next;
p->next = q->next;
free(q);
return head;
}
/* 修改链表中的节点 */
void modifyNode(Node* head, int index, int data) {
if (index < 0) {
printf("Error: invalid index!\n");
return;
}
Node* p = head;
for (int i = 0; i < index; i++) { // 找到待修改节点
if (p == NULL) {
printf("Error: invalid index!\n");
return;
}
p = p->next;
}
p->data = data;
}
/* 查找链表中的节点 */
int searchNode(Node* head, int data) {
int index = 0;
for (Node* p = head; p != NULL; p = p->next) {
if (p->data == data) {
return index;
}
index++;
}
return -1;
}
/* 主函数 */
int main() {
int n;
printf("Please input the length of the list: ");
scanf("%d", &n);
Node* head = createList(n);
printList(head);
// 插入节点
head = insertNode(head, 2, 100);
printList(head);
// 删除节点
head = deleteNode(head, 4);
printList(head);
// 修改节点
modifyNode(head, 1, 200);
printList(head);
// 查找节点
int index = searchNode(head, 200);
if (index != -1) {
printf("Node 200 is found at index %d.\n", index);
} else {
printf("Node 200 is not found in the list.\n");
}
return 0;
}
```
这个程序实现了链表的创建、打印、插入、删除、修改和查找功能。在程序中,我们定义了一个链表节点结构体,包含一个数据域和一个指针域,指向下一个节点。通过遍历链表,我们可以依次访问每个节点的数据域。在主函数中,我们首先输入链表的长度,然后调用 `createList()` 函数创建链表,并调用 `printList()` 函数打印链表。接着,我们演示了如何向链表中插入节点、删除节点、修改节点和查找节点。
阅读全文
相关推荐
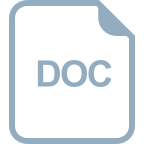
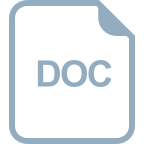
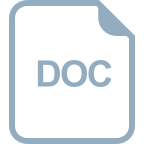
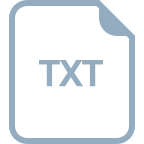
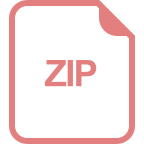




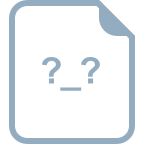
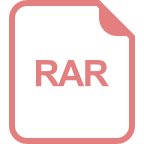
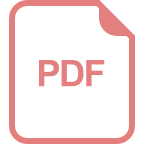
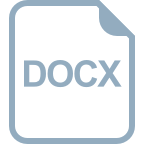
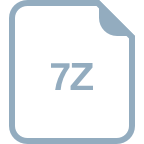
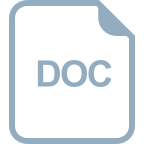
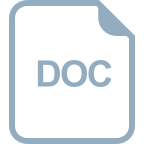

