写一个c语言增删改查的链表
时间: 2023-09-11 13:10:31 浏览: 100
好的,以下是一个C语言实现增删改查的链表的代码:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
struct Node* head = NULL;
// 向链表中插入一个节点
void insert(int data) {
struct Node* newNode = (struct Node*) malloc(sizeof(struct Node));
newNode->data = data;
newNode->next = NULL;
if (head == NULL) {
head = newNode;
return;
}
struct Node* temp = head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
// 删除一个节点
void delete(int data) {
struct Node* temp = head;
struct Node* prev = NULL;
if (temp != NULL && temp->data == data) {
head = temp->next;
free(temp);
return;
}
while (temp != NULL && temp->data != data) {
prev = temp;
temp = temp->next;
}
if (temp == NULL) {
printf("Node with data %d not found\n", data);
return;
}
prev->next = temp->next;
free(temp);
}
// 修改一个节点的值
void modify(int oldData, int newData) {
struct Node* temp = head;
while (temp != NULL && temp->data != oldData) {
temp = temp->next;
}
if (temp == NULL) {
printf("Node with data %d not found\n", oldData);
return;
}
temp->data = newData;
}
// 查找链表中是否存在指定的节点
void search(int data) {
struct Node* temp = head;
while (temp != NULL && temp->data != data) {
temp = temp->next;
}
if (temp == NULL) {
printf("Node with data %d not found\n", data);
return;
}
printf("Node with data %d found\n", data);
}
// 打印链表的所有元素
void printList() {
struct Node* temp = head;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
}
int main() {
// 向链表插入5个节点,值分别为1, 2, 3, 4, 5
insert(1);
insert(2);
insert(3);
insert(4);
insert(5);
printf("链表的初始状态:\n");
printList();
// 通过调用各自的函数对节点进行删除、修改、查找等操作
delete(3);
printf("\n删除了值为3的节点:\n");
printList();
modify(4, 6);
printf("\n修改了值为4的节点,将其值改为6:\n");
printList();
search(2);
search(7);
return 0;
}
```
以上是一个基本的增删改查链表的实现,可以供开发者参考和学习。
阅读全文
相关推荐
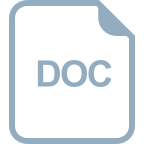
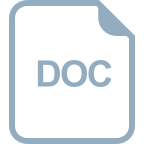
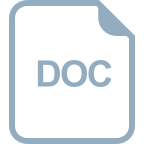




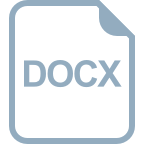
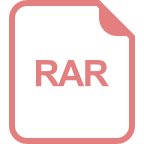
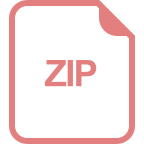
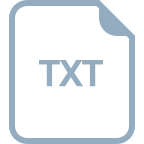
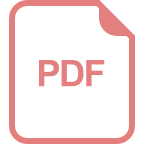






