C语言链表的增删改查
时间: 2023-09-22 07:11:24 浏览: 122
链表是一种常见的数据结构,用于存储和操作数据元素。下面是关于C语言链表的增删改查的一些基本操作:
1. 添加节点(增加):
- 创建一个新节点。
- 将新节点的数据赋值。
- 将新节点的next指针指向当前链表的头节点(或者指向NULL,如果链表为空)。
- 更新链表的头节点指针,使其指向新节点。
2. 删除节点(删除):
- 找到要删除的节点以及它的前一个节点。
- 将前一个节点的next指针指向要删除节点的下一个节点。
- 释放要删除节点的内存。
3. 修改节点(改变):
- 遍历链表,找到要修改的节点。
- 修改该节点的数据。
4. 查找节点(查询):
- 从链表的头节点开始遍历,直到找到包含所需数据的节点。
- 如果找到了,返回该节点;如果没有找到,返回NULL或者其他特定值。
下面是一个简单的示例代码,演示了链表的增删改查操作:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
void insertNode(struct Node** head, int data) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = data;
newNode->next = *head;
*head = newNode;
}
void deleteNode(struct Node** head, int data) {
struct Node* currentNode = *head;
struct Node* previousNode = NULL;
if (currentNode != NULL && currentNode->data == data) {
*head = currentNode->next;
free(currentNode);
return;
}
while (currentNode != NULL && currentNode->data != data) {
previousNode = currentNode;
currentNode = currentNode->next;
}
if (currentNode == NULL) {
printf("Node not found.\n");
return;
}
previousNode->next = currentNode->next;
free(currentNode);
}
void modifyNode(struct Node* head, int data, int newData) {
while (head != NULL) {
if (head->data == data) {
head->data = newData;
return;
}
head = head->next;
}
printf("Node not found.\n");
}
struct Node* searchNode(struct Node* head, int data) {
while (head != NULL) {
if (head->data == data) {
return head;
}
head = head->next;
}
return NULL;
}
void printList(struct Node* node) {
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
printf("\n");
}
int main() {
struct Node* head = NULL;
insertNode(&head, 3);
insertNode(&head, 5);
insertNode(&head, 7);
printf("Initial list: ");
printList(head);
deleteNode(&head, 5);
printf("Updated list after deletion: ");
printList(head);
modifyNode(head, 7, 9);
printf("Updated list after modification: ");
printList(head);
struct Node* searchedNode = searchNode(head, 9);
if (searchedNode != NULL) {
printf("Searched node found: %d\n", searchedNode->data);
} else {
printf("Searched node not found.\n");
}
return 0;
}
```
这个示例代码展示了链表的增删改查操作,你可以根据需要进行修改和扩展。希望对你有帮助!
相关推荐
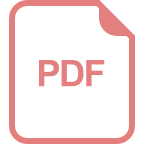














