用c语言写顺序链表的增删改查
时间: 2024-10-13 20:16:20 浏览: 32
在C语言中,我们可以使用结构体和指针来创建顺序链表。以下是基本的链表操作——插入、删除、查找和修改:
1. **插入操作(添加元素到链表末尾)**:
```c
struct Node {
int data;
struct Node* next; // 指向下一个节点的指针
};
void insert(struct Node** head, int value) {
if (*head == NULL) { // 如果链表为空
*head = (struct Node*)malloc(sizeof(struct Node));
(*head)->data = value;
(*head)->next = NULL;
} else {
struct Node* new_node = (struct Node*)malloc(sizeof(struct Node));
new_node->data = value;
new_node->next = (*head)->next;
(*head)->next = new_node;
}
}
```
2. **删除操作(删除特定值的节点)**:
```c
void deleteNode(struct Node** head, int value) {
struct Node* temp = *head, *prev = NULL;
if (temp != NULL && temp->data == value) {
*head = temp->next; // 如果头节点就是要删除的
free(temp); // 释放内存
return;
}
while (temp != NULL && temp->data != value) {
prev = temp;
temp = temp->next;
}
if (temp == NULL) return; // 没找到要删除的节点
prev->next = temp->next;
free(temp);
}
```
3. **查找操作(查找指定值的节点)**:
```c
struct Node* search(struct Node* head, int value) {
struct Node* current = head;
while (current != NULL) {
if (current->data == value)
return current;
current = current->next;
}
return NULL; // 如果未找到,返回NULL
}
```
4. **修改操作(更新节点数据)**:
```c
void updateNode(struct Node** head, int old_value, int new_value) {
struct Node* node = search(*head, old_value);
if (node != NULL) {
node->data = new_value;
} else {
printf("Element not found in the list.\n");
}
}
```
阅读全文
相关推荐
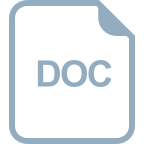
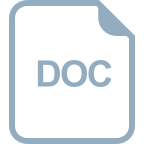
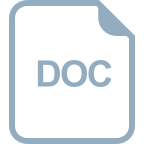



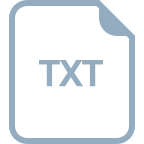
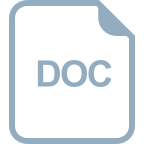



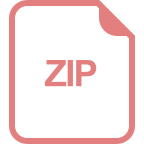
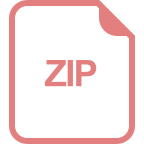
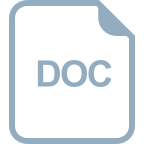



