利用SQLite库进行数据库操作,程序应有数据库录入功能,按字段名查询功能,记录的修改和删除功能,要求写在一个文件,并在控制台有输入提醒
时间: 2024-03-20 07:42:05 浏览: 18
好的,下面是一个示例代码,实现了数据库录入、查询、修改和删除功能,并在控制台有输入提醒:
```python
import sqlite3
# 打开数据库连接
conn = sqlite3.connect('example.db')
# 创建一个表
conn.execute('''CREATE TABLE IF NOT EXISTS COMPANY
(ID INT PRIMARY KEY NOT NULL,
NAME TEXT NOT NULL,
AGE INT NOT NULL,
ADDRESS CHAR(50),
SALARY REAL);''')
while True:
print("请选择要进行的操作:")
print("1. 录入数据")
print("2. 按字段名查询数据")
print("3. 修改数据")
print("4. 删除数据")
print("5. 退出程序")
choice = input("请输入数字选择操作:")
if choice == '1':
id = input("请输入ID:")
name = input("请输入姓名:")
age = input("请输入年龄:")
address = input("请输入地址:")
salary = input("请输入工资:")
# 插入数据
conn.execute("INSERT INTO COMPANY (ID, NAME, AGE, ADDRESS, SALARY) \
VALUES (?, ?, ?, ?, ?)", (id, name, age, address, salary))
# 提交事务
conn.commit()
print("数据已成功录入!")
elif choice == '2':
field = input("请输入要查询的字段名:")
value = input("请输入要查询的值:")
# 查询数据
cursor = conn.execute("SELECT * FROM COMPANY WHERE {} = ?".format(field), (value,))
rows = cursor.fetchall()
if len(rows) == 0:
print("没有找到符合条件的记录!")
else:
for row in rows:
print("ID = ", row[0])
print("NAME = ", row[1])
print("AGE = ", row[2])
print("ADDRESS = ", row[3])
print("SALARY = ", row[4], "\n")
elif choice == '3':
id = input("请输入要修改的记录的ID:")
field = input("请输入要修改的字段名:")
value = input("请输入修改后的值:")
# 更新数据
conn.execute("UPDATE COMPANY SET {} = ? WHERE ID = ?".format(field), (value, id))
conn.commit()
print("数据已成功修改!")
elif choice == '4':
id = input("请输入要删除的记录的ID:")
# 删除数据
conn.execute("DELETE FROM COMPANY WHERE ID = ?", (id,))
conn.commit()
print("数据已成功删除!")
elif choice == '5':
break
else:
print("输入有误,请重新输入!")
# 关闭连接
conn.close()
```
在控制台中执行这个程序,可以按照提示进行数据库操作。
相关推荐
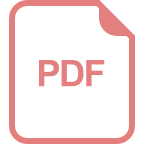
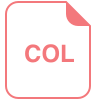
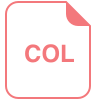
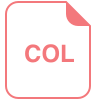
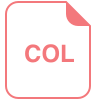
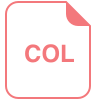









