python决策树鸢尾花id3算法代码
时间: 2023-08-11 18:43:25 浏览: 101
以下是使用Python实现决策树鸢尾花ID3算法的示例代码:
```python
import pandas as pd
import numpy as np
# 定义节点的类
class Node:
def __init__(self, feature=None, label=None, sub_nodes=None):
self.feature = feature # 当前节点的特征
self.label = label # 当前节点的标签
self.sub_nodes = sub_nodes # 当前节点的子节点
# 定义决策树的类
class DecisionTree:
def __init__(self, epsilon=0.1):
self.epsilon = epsilon # 定义划分阈值
# 计算信息熵
def calc_entropy(self, data):
labels = data[:, -1]
label_count = np.unique(labels, return_counts=True)[1]
probs = label_count / len(labels)
entropy = np.sum(-probs * np.log2(probs))
return entropy
# 计算条件熵
def calc_condition_entropy(self, data, feature_idx):
feature_values = data[:, feature_idx]
unique_values = np.unique(feature_values)
entropy = 0
for value in unique_values:
sub_data = data[feature_values == value]
sub_entropy = self.calc_entropy(sub_data)
entropy += (len(sub_data) / len(data)) * sub_entropy
return entropy
# 选择最优划分特征
def choose_best_feature(self, data):
feature_count = data.shape[1] - 1
max_info_gain = 0
best_feature_idx = 0
base_entropy = self.calc_entropy(data)
for i in range(feature_count):
condition_entropy = self.calc_condition_entropy(data, i)
info_gain = base_entropy - condition_entropy
if info_gain > max_info_gain:
max_info_gain = info_gain
best_feature_idx = i
return best_feature_idx
# 构建决策树
def build_tree(self, data):
labels = data[:, -1]
if len(np.unique(labels)) == 1:
return Node(label=labels[0])
if data.shape[1] == 1:
return Node(label=np.argmax(np.bincount(labels)))
best_feature_idx = self.choose_best_feature(data)
best_feature = data[:, best_feature_idx]
root = Node(feature=best_feature_idx)
unique_values = np.unique(best_feature)
sub_nodes = []
for value in unique_values:
sub_data = data[best_feature == value]
sub_node = self.build_tree(sub_data)
sub_nodes.append(sub_node)
root.sub_nodes = sub_nodes
return root
# 预测单个样本的类别
def predict_sample(self, root, sample):
while root.sub_nodes:
feature_idx = root.feature
feature_value = sample[feature_idx]
sub_node = root.sub_nodes[int(feature_value)]
root = sub_node
return root.label
# 预测测试集的类别
def predict(self, root, test_data):
predictions = []
for sample in test_data:
prediction = self.predict_sample(root, sample)
predictions.append(prediction)
return np.array(predictions)
# 计算准确率
def accuracy(self, y_true, y_pred):
return np.sum(y_true == y_pred) / len(y_true)
# 读取数据集
data = pd.read_csv('iris.csv').values
np.random.shuffle(data)
train_data = data[:120]
test_data = data[120:]
# 构建决策树并预测测试集
dt = DecisionTree()
root = dt.build_tree(train_data)
y_true = test_data[:, -1]
y_pred = dt.predict(root, test_data[:, :-1])
print('Accuracy:', dt.accuracy(y_true, y_pred))
```
说明:
- 该代码使用了鸢尾花数据集,数据集文件名为`iris.csv`,可以自行更改为其他数据集。
- 在`DecisionTree`类的构造函数中,定义了划分阈值`epsilon`,默认值为`0.1`。
- `Node`类表示决策树的节点,包含特征、标签和子节点三个属性。
- `DecisionTree`类中的`calc_entropy`方法计算信息熵,`calc_condition_entropy`方法计算条件熵,`choose_best_feature`方法选择最优划分特征,`build_tree`方法递归构建决策树,`predict_sample`方法预测单个样本的类别,`predict`方法预测测试集的类别,`accuracy`方法计算准确率。
- 最后输出测试集的准确率。
阅读全文
相关推荐
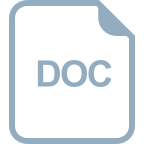
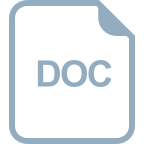
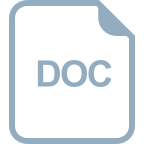
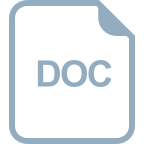








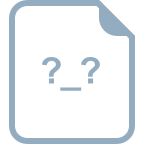
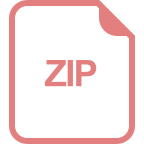
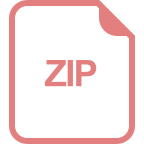
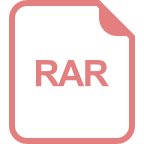


