ID3鸢尾花python
时间: 2023-09-11 21:05:02 浏览: 95
ID3决策树是一种常用的分类算法,可以用于鸢尾花数据集的分类。下面是使用Python实现ID3算法对鸢尾花数据集进行分类的示例代码:
```python
import pandas as pd
import numpy as np
from math import log2
# 加载鸢尾花数据集
iris_data = pd.read_csv('iris.csv')
# 定义ID3决策树算法
def id3(data, target):
# 如果数据集中只有一种类别,直接返回该类别
if len(set(data[target])) == 1:
return data[target].iloc[0]
# 如果特征集为空,返回数据集中出现最多的类别
if len(data.columns) == 1:
return data[target].value_counts().idxmax()
# 计算每个特征的信息增益
ent = entropy(data[target])
gains = {}
for col in data.columns[:-1]:
gains[col] = ent - conditional_entropy(data, col, target)
# 选择信息增益最大的特征作为节点
node = max(gains, key=gains.get)
# 构建子树
tree = {node:{}}
for val in set(data[node]):
subset = data[data[node] == val].drop(columns=node)
tree[node][val] = id3(subset, target)
return tree
# 计算熵
def entropy(target):
n = len(target)
counts = target.value_counts()
probs = counts / n
return -(probs * np.log2(probs)).sum()
# 计算条件熵
def conditional_entropy(data, feature, target):
n = len(data)
ent = 0
for val in set(data[feature]):
subset = data[data[feature] == val]
ent += len(subset) / n * entropy(subset[target])
return ent
# 使用ID3算法构建决策树
tree = id3(iris_data, 'class')
# 打印决策树
print(tree)
```
以上代码中,通过读取鸢尾花数据集,定义了ID3算法函数和计算熵、条件熵的函数,最后使用ID3算法构建决策树并打印输出。在运行代码前,需要准备一份名为“iris.csv”的鸢尾花数据集文件。
阅读全文
相关推荐
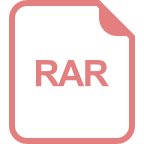







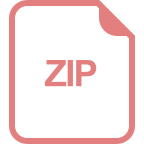
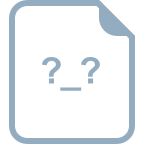
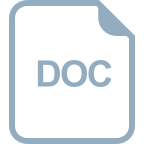





