python实现tps插值
时间: 2023-06-29 12:19:21 浏览: 78
以下是一个基于Python的TPS插值示例代码,使用scipy库:
```python
import numpy as np
from scipy.spatial.distance import cdist
from scipy.linalg import solve
def tps_fit(X, Y, lmbda=0.):
"""
TPS拟合函数
:param X: 输入点,shape为(N, 2)
:param Y: 输出点,shape为(N, C)
:param lmbda: 正则化强度
:return: TPS拟合函数
"""
N, d = X.shape
K = rbf_kernel_matrix(X)
P = np.hstack((np.ones((N, 1)), X))
L = np.vstack((np.hstack((K + lmbda * np.eye(N), P)), np.hstack((P.T, np.zeros((d + 1, d + 1))))))
v = np.zeros((N + d + 1, Y.shape[1]))
v[:N] = Y
# 解线性方程组,求解参数
w = solve(L, v)
return lambda x: tps_predict(x, X, w)
def tps_predict(Xnew, X, w):
"""
TPS预测函数
:param Xnew: 待预测点,shape为(M, 2)
:param X: 输入点,shape为(N, 2)
:param w: TPS拟合函数的参数
:return: 预测值,shape为(M, C)
"""
M, d = Xnew.shape
K = rbf_kernel_matrix(Xnew, X)
P = np.hstack((np.ones((M, 1)), Xnew))
return np.dot(np.hstack((K, P)), w)
def rbf_kernel_matrix(X, Y=None, gamma=1.0):
"""
计算RBF核矩阵
:param X: 第一个输入矩阵,shape为(N, d)
:param Y: 第二个输入矩阵,shape为(M, d),如果为None,则默认为X
:param gamma: RBF核函数的gamma参数
:return: RBF核矩阵,shape为(N, M)
"""
Y = X if Y is None else Y
dist2 = cdist(X / gamma, Y / gamma, metric='sqeuclidean')
return np.exp(-dist2)
# 测试代码
import matplotlib.pyplot as plt
# 生成测试数据
X = np.random.rand(20, 2) * 2 - 1
Y = np.sin(X[:, 0] * np.pi) * np.cos(X[:, 1] * np.pi)
# TPS拟合
f_tps = tps_fit(X, Y)
# 生成测试点
Xtest = np.mgrid[-1:1:50j, -1:1:50j].reshape(2, -1).T
# TPS预测
Ytest = f_tps(Xtest)
# 绘制结果
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(10, 5))
ax1.scatter(X[:, 0], X[:, 1], c=Y, cmap=plt.cm.RdBu_r)
ax1.set_title('Data')
ax2.scatter(Xtest[:, 0], Xtest[:, 1], c=Ytest, cmap=plt.cm.RdBu_r)
ax2.set_title('TPS interpolation')
plt.show()
```
在上面的代码中,首先生成了一组测试数据,然后使用`tps_fit`函数对数据进行了TPS拟合。接着,生成了一些测试点,并使用`tps_predict`函数对测试点进行了预测。最后,将原始数据和插值结果绘制在了同一张图中,以便于比较。
在TPS插值中,需要注意的是正则化强度的选择。如果正则化强度设置得过小,可能会导致过拟合,而过大则会导致欠拟合。因此,需要通过交叉验证等方法来确定合适的正则化强度。
相关推荐
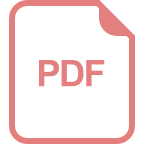
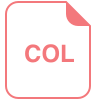
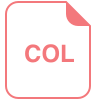
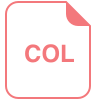
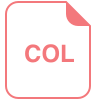
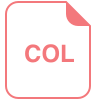









