unordered_set.find()返回值
时间: 2023-12-10 15:00:26 浏览: 234
`unordered_set::find()` 返回一个迭代器,指向第一个找到的元素。如果没有找到元素,则返回 `unordered_set::end()` 迭代器。因此,我们通常使用以下方式来检查元素是否存在于 `unordered_set` 中:
```cpp
unordered_set<int> mySet = {1, 2, 3};
unordered_set<int>::iterator it = mySet.find(2);
if (it != mySet.end()) {
// 元素 2 存在于 mySet 中
} else {
// 元素 2 不存在于 mySet 中
}
```
在 C++11 中,可以使用 `auto` 关键字自动推导迭代器类型:
```cpp
unordered_set<int> mySet = {1, 2, 3};
auto it = mySet.find(2);
if (it != mySet.end()) {
// 元素 2 存在于 mySet 中
} else {
// 元素 2 不存在于 mySet 中
}
```
相关问题
unordered_set:find 条件查找 包含lambda表达式
unordered_set 的 find() 函数可以用于查找指定元素。如果元素存在,则返回该元素的迭代器;如果不存在,则返回 end()。
可以使用 lambda 表达式作为 find() 函数的参数,以实现条件查找。
例如,假设你有一个 unordered_set<string>,你想查找其中一个长度为 5 且以字母 'a' 开头的字符串:
```c++
#include <iostream>
#include <unordered_set>
#include <string>
int main()
{
std::unordered_set<std::string> myset = {"apple", "banana", "cherry", "date", "elderberry"};
auto it = myset.find([](const std::string& s) { return s.length() == 5 && s[0] == 'a'; });
if (it != myset.end()) {
std::cout << "Found: " << *it << std::endl;
} else {
std::cout << "Not found" << std::endl;
}
return 0;
}
```
上面的代码使用 lambda 表达式作为 find() 函数的参数,该 lambda 表达式接受一个 std::string 类型的参数 s,返回值为 bool 类型。如果 s 的长度为 5 且以字母 'a' 开头,则返回 true,否则返回 false。
请注意,lambda 表达式不是 unordered_set 的成员函数,而是作为参数传递给 find() 函数的。
unordered_set count 与find
unordered_set是C++标准库中的容器,它提供了一种存储唯一元素的方式,并且可以快速地进行查找、插入和删除操作。下面是对count和find两个成员函数的解释:
1. count(key)函数会返回unordered_set中等于给定键key的元素的个数。由于unordered_set中的元素是唯一的,所以返回值只能是0或1。
2. find(key)函数会返回一个迭代器,指向unordered_set中第一个等于给定键key的元素。如果不存在这样的元素,则返回一个指向unordered_set尾部的迭代器end()。
以下是一个示例代码,演示了如何使用count和find函数:
```cpp
#include <iostream>
#include <unordered_set>
int main() {
std::unordered_set<int> mySet = {1, 2, 3, 4, 5};
// 使用count函数查找元素
int count = mySet.count(3);
std::cout << "Count of 3: " << count << std::endl;
// 使用find函数查找元素
auto it = mySet.find(4);
if (it != mySet.end()) {
std::cout << "Element found: " << *it << std::endl;
} else {
std::cout << "Element not found" << std::endl;
}
return 0;
}
```
输出结果:
```
Count of 3: 1
Element found: 4
```
阅读全文
相关推荐
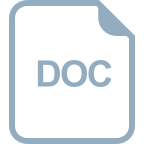
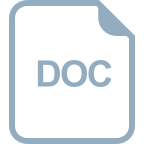
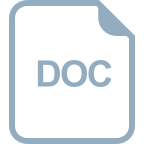
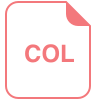
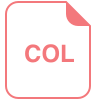
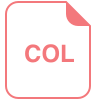
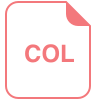




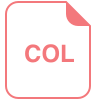

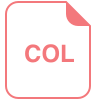
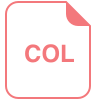
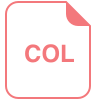
