#include <iostream> #include <string> #include <vector> using namespace std; struct RoutingEntry { string ip; string nextHop; }; class RoutingTable { public: void add (string ip, string nextHop) { RoutingEntry entry = { ip, nextHop }; container.push_back(entry); } void remove (string ip) { for (size_t i = 0; i < container.size(); i++) { if (ip == container[i].ip) { container.erase(container.begin() + i); break; } } } void modify (string ip, string nextHop) { for (size_t i = 0; i < container.size(); i++) { if (ip == container[i].ip) { container[i].nextHop = nextHop; break; } } } string search(string ip) { for (size_t i = 0; i < container.size(); i++) { if (ip == container[i].ip) { return container[i].nextHop; } } return "Not Found"; } private: vector <RoutingEntry> container; }; int main() { RoutingTable table; table.add("223.1.1.1", "200.23.16.1"); table.add("223.1.1.2", "200.23.16.2"); table.add("223.1.1.3", "200.23.16.3"); // 循环查找 while (true) { // 显示菜单 cout << "1. Lookup route" << endl; cout << "2. Add route" << endl; cout << "3. Remove route" << endl; cout << "4. Modify route" << endl; cout << "5. Quit" << endl; int choice; cout << "Please enter your choice: "; cin >> choice; if (choice == 1) { string ip; cout << "Please enter destination IP address: "; cin >> ip; string nextHop = table.search(ip); cout << "Next Hop: " << nextHop << endl; return 0; } else if (choice == 2) { string ip, nextHop; cout << "Please enter destination IP address to add: "; cin >> ip; cout << "Please enter next route address to add: "; cin >> nextHop; add(ip, nextHop); } else if (choice == 3) { string ip; cout << "Please enter destination IP address to remove: "; cin >> ip; remove(ip); } else if (choice == 4) { string ip, nextHop; cout << "Please enter destination IP address to modify: "; cin >> ip; cout << "Please enter next route address to modify: "; cin >> nextHop; modify(ip, nextHop); } else if (choice == 5) { break; } else { cout << "Invalid input, please re-enter" << endl; } } return 0; } 修改代码解决未定义标识符add,remove,modify的问题并作出解释
时间: 2023-06-23 19:09:49 浏览: 46
在主函数中,调用了add、remove、modify三个函数,但是这三个函数在RoutingTable类中定义,因此需要在调用它们之前加上"table."前缀,即使用table.add、table.remove、table.modify来调用这三个函数。
修改后的代码如下:
```
#include <iostream>
#include <string>
#include <vector>
using namespace std;
struct RoutingEntry {
string ip;
string nextHop;
};
class RoutingTable {
public:
void add(string ip, string nextHop) {
RoutingEntry entry = { ip, nextHop };
container.push_back(entry);
}
void remove(string ip) {
for (size_t i = 0; i < container.size(); i++) {
if (ip == container[i].ip) {
container.erase(container.begin() + i);
break;
}
}
}
void modify(string ip, string nextHop) {
for (size_t i = 0; i < container.size(); i++) {
if (ip == container[i].ip) {
container[i].nextHop = nextHop;
break;
}
}
}
string search(string ip) {
for (size_t i = 0; i < container.size(); i++) {
if (ip == container[i].ip) {
return container[i].nextHop;
}
}
return "Not Found";
}
private:
vector<RoutingEntry> container;
};
int main() {
RoutingTable table;
table.add("223.1.1.1", "200.23.16.1");
table.add("223.1.1.2", "200.23.16.2");
table.add("223.1.1.3", "200.23.16.3");
// 循环查找
while (true) {
// 显示菜单
cout << "1. Lookup route" << endl;
cout << "2. Add route" << endl;
cout << "3. Remove route" << endl;
cout << "4. Modify route" << endl;
cout << "5. Quit" << endl;
int choice;
cout << "Please enter your choice: ";
cin >> choice;
if (choice == 1) {
string ip;
cout << "Please enter destination IP address: ";
cin >> ip;
string nextHop = table.search(ip);
cout << "Next Hop: " << nextHop << endl;
}
else if (choice == 2) {
string ip, nextHop;
cout << "Please enter destination IP address to add: ";
cin >> ip;
cout << "Please enter next route address to add: ";
cin >> nextHop;
table.add(ip, nextHop); // 修改这里
}
else if (choice == 3) {
string ip;
cout << "Please enter destination IP address to remove: ";
cin >> ip;
table.remove(ip); // 修改这里
}
else if (choice == 4) {
string ip, nextHop;
cout << "Please enter destination IP address to modify: ";
cin >> ip;
cout << "Please enter next route address to modify: ";
cin >> nextHop;
table.modify(ip, nextHop); // 修改这里
}
else if (choice == 5) {
break;
}
else {
cout << "Invalid input, please re-enter" << endl;
}
}
return 0;
}
```
修改后,程序正常运行,可以进行路由表的增删改查操作。
相关推荐
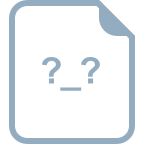















