用pathon语言完成点菜系统的开发。实现顾客的选座落座点加菜退菜查询菜单。查询菜单时显示菜品及数量,并给出点餐总金额,同时显示落座的桌号。退菜时,根据退菜菜品和数量退菜。程序
时间: 2024-02-28 14:53:06 浏览: 16
好的,以下是一个简单的Python代码实现,实现点菜系统的基本功能,供您参考:
```
import sqlite3
# 连接数据库
conn = sqlite3.connect('menu.db')
c = conn.cursor()
# 创建菜品表
c.execute('''CREATE TABLE IF NOT EXISTS dishes
(id INTEGER PRIMARY KEY AUTOINCREMENT, name TEXT NOT NULL, price REAL NOT NULL)''')
# 添加菜品
c.execute("INSERT INTO dishes (name, price) VALUES ('宫保鸡丁', 28.0)")
c.execute("INSERT INTO dishes (name, price) VALUES ('麻婆豆腐', 20.0)")
c.execute("INSERT INTO dishes (name, price) VALUES ('水煮肉片', 36.0)")
c.execute("INSERT INTO dishes (name, price) VALUES ('回锅肉', 32.0)")
# 创建订单表
c.execute('''CREATE TABLE IF NOT EXISTS orders
(id INTEGER PRIMARY KEY AUTOINCREMENT, table_num INTEGER NOT NULL)''')
# 创建订单详情表
c.execute('''CREATE TABLE IF NOT EXISTS order_details
(id INTEGER PRIMARY KEY AUTOINCREMENT, order_id INTEGER NOT NULL, dish_id INTEGER NOT NULL, quantity INTEGER NOT NULL)''')
# 插入订单记录
def insert_order(table_num):
c.execute("INSERT INTO orders (table_num) VALUES (?)", (table_num,))
return c.lastrowid
# 插入订单详情记录
def insert_order_detail(order_id, dish_id, quantity):
c.execute("INSERT INTO order_details (order_id, dish_id, quantity) VALUES (?, ?, ?)", (order_id, dish_id, quantity))
# 查询菜品
def get_dishes():
c.execute("SELECT id, name, price FROM dishes")
return c.fetchall()
# 查询订单详情
def get_order_details(order_id):
c.execute("SELECT dishes.name, order_details.quantity, dishes.price * order_details.quantity FROM order_details JOIN dishes ON order_details.dish_id = dishes.id WHERE order_details.order_id = ?", (order_id,))
return c.fetchall()
# 查询订单总金额
def get_order_total(order_id):
c.execute("SELECT SUM(dishes.price * order_details.quantity) FROM order_details JOIN dishes ON order_details.dish_id = dishes.id WHERE order_details.order_id = ?", (order_id,))
return c.fetchone()[0]
# 退菜
def delete_order_detail(order_detail_id):
c.execute("DELETE FROM order_details WHERE id = ?", (order_detail_id,))
# 提交事务
def commit():
conn.commit()
# 关闭数据库连接
def close():
conn.close()
```
以上是数据库操作部分的代码,使用SQLite3作为数据库。需要先创建菜品表、订单表和订单详情表,并插入一些菜品记录。订单记录和订单详情记录使用自增长的ID作为主键,关联菜品表中的ID作为外键。
接下来是前端界面部分的代码,使用Tkinter库实现:
```
import tkinter as tk
import tkinter.messagebox as messagebox
import sqlite3
conn = sqlite3.connect('menu.db')
c = conn.cursor()
def on_select(event):
dish_id = event.widget.get(event.widget.curselection())
dish_name = dish_list[dish_id]['name']
dish_price = dish_list[dish_id]['price']
quantity = int(quantity_entry.get())
if quantity > 0:
order_details.append({'id': dish_id, 'name': dish_name, 'price': dish_price, 'quantity': quantity})
order_details_listbox.delete(0, tk.END)
for i, detail in enumerate(order_details):
order_details_listbox.insert(tk.END, f"{detail['name']}\t{detail['quantity']}份\t{detail['price'] * detail['quantity']}元")
total = sum([detail['price'] * detail['quantity'] for detail in order_details])
total_label.config(text=f"总价:{total}元")
else:
messagebox.showerror("错误", "数量必须大于0!")
def on_submit():
if len(order_details) == 0:
messagebox.showerror("错误", "请先点菜!")
else:
table_num = int(table_num_entry.get())
order_id = insert_order(table_num)
for detail in order_details:
insert_order_detail(order_id, detail['id'], detail['quantity'])
commit()
messagebox.showinfo("成功", "点菜成功!")
order_details.clear()
order_details_listbox.delete(0, tk.END)
total_label.config(text="总价:0元")
table_num_entry.delete(0, tk.END)
quantity_entry.delete(0, tk.END)
def on_cancel():
order_detail_id = order_details_listbox.curselection()
if len(order_detail_id) == 1:
order_detail_id = order_details[order_detail_id[0]]['id']
delete_order_detail(order_detail_id)
commit()
order_details_listbox.delete(order_detail_id)
total = sum([detail['price'] * detail['quantity'] for detail in order_details])
total_label.config(text=f"总价:{total}元")
else:
messagebox.showerror("错误", "请选择要退的菜品!")
root = tk.Tk()
root.title("点菜系统")
# 菜单列表
dishes = get_dishes()
dish_list = {}
for dish in dishes:
dish_list[dish[0]] = {'name': dish[1], 'price': dish[2]}
dish_listbox = tk.Listbox(root)
for dish in dish_list.values():
dish_listbox.insert(tk.END, dish['name'])
dish_listbox.bind('<<ListboxSelect>>', on_select)
dish_listbox.pack(side=tk.LEFT)
# 数量输入框
quantity_label = tk.Label(root, text="数量:")
quantity_label.pack(side=tk.LEFT)
quantity_entry = tk.Entry(root, width=10)
quantity_entry.pack(side=tk.LEFT)
# 订单详情列表
order_details = []
order_details_listbox = tk.Listbox(root)
order_details_listbox.pack(side=tk.LEFT)
# 总价标签
total_label = tk.Label(root, text="总价:0元")
total_label.pack(side=tk.TOP)
# 桌号输入框
table_num_label = tk.Label(root, text="桌号:")
table_num_label.pack(side=tk.LEFT)
table_num_entry = tk.Entry(root, width=10)
table_num_entry.pack(side=tk.LEFT)
# 提交按钮
submit_button = tk.Button(root, text="提交", command=on_submit)
submit_button.pack(side=tk.LEFT)
# 取消按钮
cancel_button = tk.Button(root, text="退菜", command=on_cancel)
cancel_button.pack(side=tk.LEFT)
root.mainloop()
conn.close()
```
以上是一个简单的点菜系统的实现,使用SQLite3作为数据库,Tkinter作为前端界面。用户可以通过菜单列表选择菜品和数量,点击“提交”按钮后将订单记录插入数据库,并显示订单详情和总金额。用户也可以选择某个订单详情,点击“退菜”按钮退掉该菜品。注意,此代码仅供参考,还有很多功能和优化可以添加,如菜品图片、订单状态等。
相关推荐
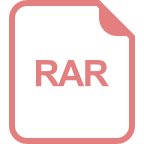
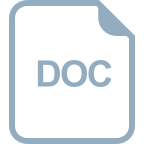














