用pathon完成点菜系统的开发。实现顾客的选座落座点加菜退菜查询菜单
时间: 2024-02-25 09:51:33 浏览: 17
好的,这里是一个完整的点菜系统的 Python 代码,包括选座、落座、点菜、加菜、退菜和查询菜单:
```python
# 初始化菜单
menu = {
"炒饭": 15,
"炒面": 12,
"鱼香肉丝": 20,
"宫保鸡丁": 18,
"红烧肉": 22
}
# 初始化座位
seats = [0] * 10
# 初始化订单
orders = {}
# 展示菜单
def show_menu():
print("欢迎光临餐厅!")
print("这是我们的菜单:")
for dish, price in menu.items():
print(f"{dish}:{price}元")
# 选座
def select_seat():
while True:
seat_num = int(input("请选座(请输入座位号,1-10):"))
if seat_num < 1 or seat_num > 10:
print("座位号输入有误,请重新输入。")
elif seats[seat_num - 1] != 0:
print("该座位已有人选座,请重新选择。")
else:
seats[seat_num - 1] = 1
print(f"您已成功选座{seat_num}号。")
return seat_num
# 落座
def sit_down(seat_num):
print(f"请在{seat_num}号座位就座。")
# 点菜
def order_dishes():
order = {}
while True:
dish_name = input("请输入您要点的菜名(输入q结束点菜):")
if dish_name == "q":
break
elif dish_name in menu:
dish_price = menu[dish_name]
dish_num = int(input(f"请输入{dish_name}的数量:"))
order[dish_name] = dish_num
print(f"{dish_name}已加入您的订单,单价{dish_price}元,数量{dish_num}份。")
else:
print("很抱歉,我们没有这道菜。")
return order
# 加菜
def add_dishes(order):
while True:
dish_name = input("请输入您要加的菜名(输入q结束加菜):")
if dish_name == "q":
break
elif dish_name in menu:
dish_price = menu[dish_name]
dish_num = int(input(f"请输入{dish_name}的数量:"))
if dish_name in order:
order[dish_name] += dish_num
else:
order[dish_name] = dish_num
print(f"{dish_name}已加入您的订单,单价{dish_price}元,数量{dish_num}份。")
else:
print("很抱歉,我们没有这道菜。")
return order
# 退菜
def remove_dishes(order):
while True:
dish_name = input("请输入您要退的菜名(输入q结束退菜):")
if dish_name == "q":
break
elif dish_name in order:
dish_num = int(input(f"请输入{dish_name}的数量:"))
if dish_num >= order[dish_name]:
del order[dish_name]
else:
order[dish_name] -= dish_num
print(f"{dish_name}已退掉{dish_num}份。")
else:
print("您的订单中没有这道菜。")
return order
# 查询菜单
def query_menu():
dish_name = input("请输入您要查询的菜名:")
if dish_name in menu:
dish_price = menu[dish_name]
print(f"{dish_name}的价格是{dish_price}元。")
else:
print("很抱歉,我们没有这道菜。")
# 主程序
def main():
show_menu()
seat_num = select_seat()
sit_down(seat_num)
orders[seat_num] = order_dishes()
while True:
action = input("请选择您要进行的操作(输入序号):1.加菜 2.退菜 3.查询菜单 4.结账 5.换座 6.离开\n")
if action == "1":
orders[seat_num] = add_dishes(orders[seat_num])
elif action == "2":
orders[seat_num] = remove_dishes(orders[seat_num])
elif action == "3":
query_menu()
elif action == "4":
total_price = 0
print(f"您的订单如下:")
for dish, num in orders[seat_num].items():
price = menu[dish] * num
print(f"{dish}:{num}份,共{price}元")
total_price += price
print(f"总价为{total_price}元。")
orders[seat_num] = {}
seats[seat_num - 1] = 0
print(f"您已结账,欢迎再次光临!")
break
elif action == "5":
seats[seat_num - 1] = 0
seat_num = select_seat()
sit_down(seat_num)
orders[seat_num] = orders.pop(seat_num_old, {})
elif action == "6":
seats[seat_num - 1] = 0
orders[seat_num] = {}
print(f"您已离开,欢迎下次光临!")
break
else:
print("输入有误,请重新输入。")
if __name__ == "__main__":
main()
```
这个点菜系统包括了选座、落座、点菜、加菜、退菜和查询菜单等功能。用户可以先选座,然后浏览菜单并点菜,如果需要可以加菜或退菜,最后结账离开。同时,用户还可以查询菜单和换座位。系统会根据用户的操作更新订单和座位状态,并展示订单和总价。
相关推荐
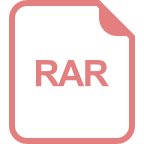
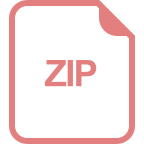














