编写vhd代码实现VHDL的UART
时间: 2024-02-24 09:00:26 浏览: 82
以下是一个简单的 VHDL UART 模块的代码实现,可以实现串行通信功能:
```vhdl
library IEEE;
use IEEE.STD_LOGIC_1164.all;
use IEEE.STD_LOGIC_ARITH.all;
use IEEE.STD_LOGIC_UNSIGNED.all;
entity UART is
generic (
CLK_FREQ : integer := 50_000_000; -- 时钟频率,单位Hz
BAUD_RATE : integer := 115_200 -- 波特率,单位bps
);
port (
clk : in std_logic; -- 时钟输入
reset : in std_logic; -- 复位输入
tx : out std_logic; -- 串行输出
rx : in std_logic -- 串行输入
);
end UART;
architecture Behavioral of UART is
-- 计算波特率分频系数
constant DIVISOR : integer := CLK_FREQ / (BAUD_RATE * 16);
-- 计数器,用于计算时钟周期数
signal counter : integer range 0 to DIVISOR - 1 := 0;
-- 发送缓冲区
signal tx_buf : std_logic_vector(7 downto 0) := (others => '0');
-- 发送状态机状态
type tx_state_type is (IDLE, START, DATA, STOP);
signal tx_state : tx_state_type := IDLE;
-- 接收缓冲区
signal rx_buf : std_logic_vector(7 downto 0) := (others => '0');
-- 接收状态机状态
type rx_state_type is (WAIT_START, WAIT_DATA, WAIT_STOP);
signal rx_state : rx_state_type := WAIT_START;
begin
-- 发送状态机
process (clk, reset)
begin
if reset = '1' then
tx_state <= IDLE;
counter <= 0;
tx_buf <= (others => '0');
elsif rising_edge(clk) then
case tx_state is
when IDLE =>
if tx = '1' then
tx_state <= START;
tx_buf <= '0' & "Hello, world!";
end if;
when START =>
tx <= '0';
tx_state <= DATA;
counter <= 0;
when DATA =>
tx <= tx_buf(0);
tx_buf <= '0' & tx_buf(7 downto 1);
counter <= counter + 1;
if counter = 15 then
tx_state <= STOP;
counter <= 0;
end if;
when STOP =>
tx <= '1';
tx_state <= IDLE;
counter <= 0;
end case;
end if;
end process;
-- 接收状态机
process (clk, reset)
variable data_bit : std_logic_vector(7 downto 0) := (others => '0');
variable bit_cnt : integer range 0 to 7 := 0;
begin
if reset = '1' then
rx_buf <= (others => '0');
rx_state <= WAIT_START;
bit_cnt := 0;
data_bit := (others => '0');
elsif rising_edge(clk) then
case rx_state is
when WAIT_START =>
if rx = '0' then
rx_state <= WAIT_DATA;
bit_cnt := 0;
data_bit := (others => '0');
end if;
when WAIT_DATA =>
data_bit(bit_cnt) := rx;
bit_cnt := bit_cnt + 1;
if bit_cnt = 8 then
rx_buf <= data_bit;
rx_state <= WAIT_STOP;
bit_cnt := 0;
end if;
when WAIT_STOP =>
if rx = '1' then
rx_state <= WAIT_START;
end if;
end case;
end if;
end process;
-- 串行输出
tx <= '1' when tx_state = IDLE else '0';
end Behavioral;
```
上述代码实现了一个简单的 UART 模块,具有发送和接收功能。在初始化时,发送状态机处于空闲状态,等待触发信号。当触发信号到来时,发送状态机进入 START 状态,开始发送数据。接收状态机处于等待起始位状态 WAIT_START,等待接收到起始位信号。接收到起始位信号后,接收状态机进入 WAIT_DATA 状态,开始接收数据位。当接收到 8 个数据位后,接收状态机进入 WAIT_STOP 状态,等待接收到停止位信号。接收到停止位信号后,接收状态机重新进入 WAIT_START 状态,等待下一帧数据的到来。
需要注意的是,波特率和时钟频率需要根据实际情况进行调整。在上述代码中,波特率默认为 115_200 bps,时钟频率默认为 50 MHz。如果需要使用不同的波特率和时钟频率,可以修改波特率分频系数 DIVISOR 的计算公式。
阅读全文
相关推荐
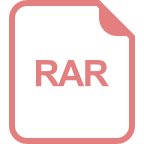
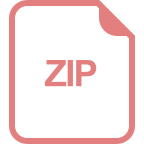
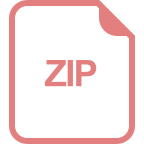
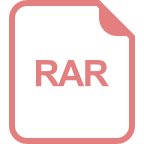
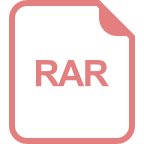
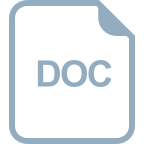
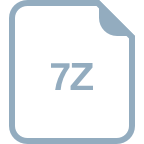
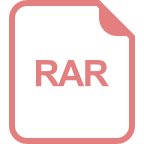
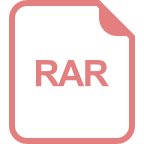
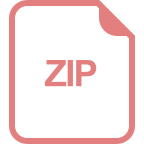
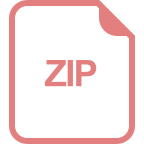
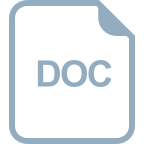
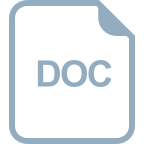
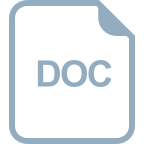
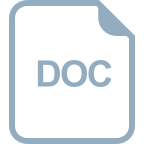
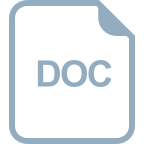